Why doesn"t RecyclerView have onItemClickListener()?
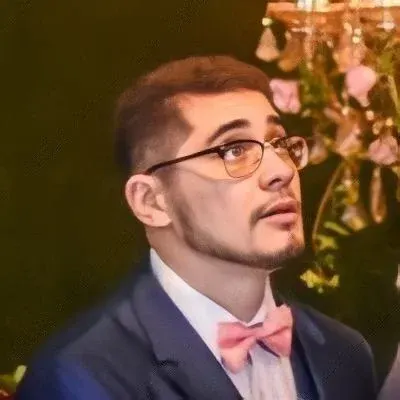
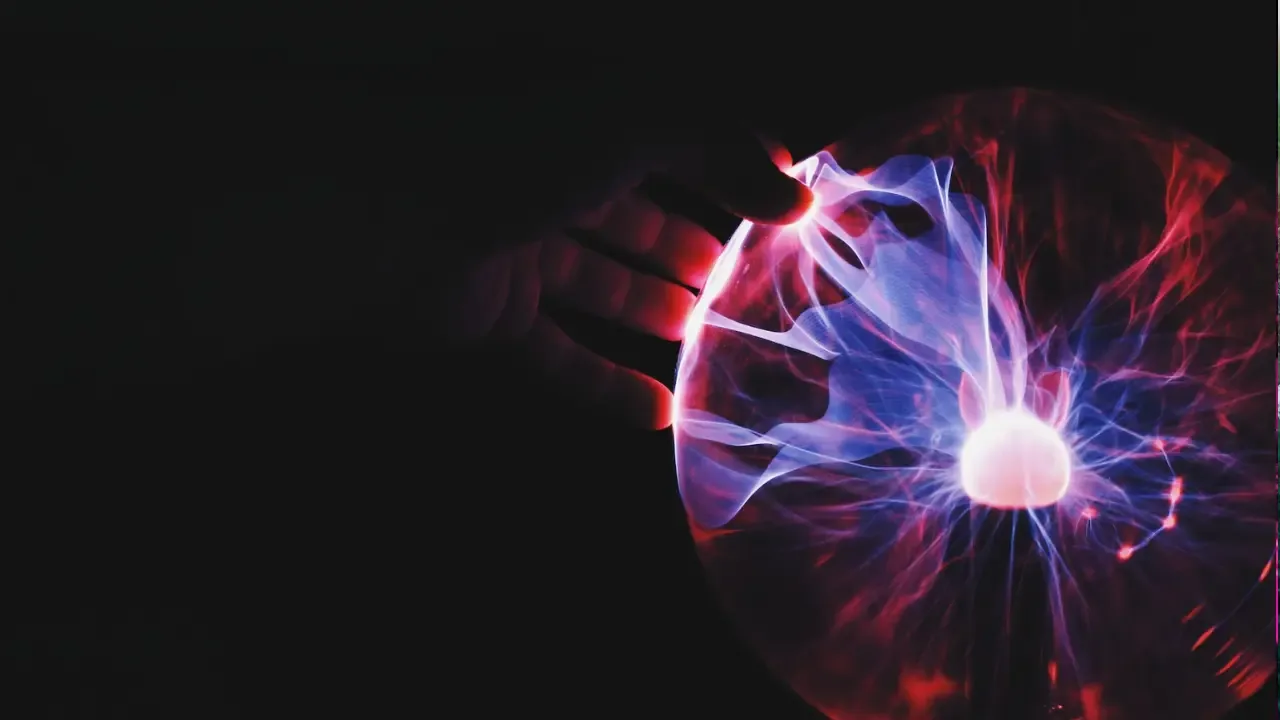
What happened to onItemClickListener() in RecyclerView?
š¢ Hey there fellow developers! š If you've been working with RecyclerView, you might have noticed that it doesn't have the handy onItemClickListener() method like its predecessor, the ListView. š® This can be a bit puzzling, but fear not! In this blog post, we'll dive into the reasons behind this change and explore alternative solutions. Let's get started! šŖ
š” The Main Question: Why Did Google Remove onItemClickListener()?
So, why did Google decide to remove onItemClickListener() from RecyclerView? š¤ The answer lies in the flexibility and performance factors of RecyclerView. Unlike ListView, RecyclerView was designed to provide better control over data and view recycling. By omitting the onItemClickListener() method, Google encourages developers to implement their own click handling logic.
š The Secondary Question: How Can We Handle Click Events in RecyclerView?
Now that we know onItemClickListener() is no longer available, let's explore a common approach for handling click events in RecyclerView. One popular solution is to implement an OnClickListener in the RecyclerView.Adapter class, just like you did, my friend! š Here's a code snippet to illustrate:
public static class ViewHolder extends RecyclerView.ViewHolder implements OnClickListener {
public TextView txtViewTitle;
public ImageView imgViewIcon;
public ViewHolder(View itemLayoutView) {
super(itemLayoutView);
txtViewTitle = itemLayoutView.findViewById(R.id.item_title);
imgViewIcon = itemLayoutView.findViewById(R.id.item_icon);
itemLayoutView.setOnClickListener(this);
}
@Override
public void onClick(View v) {
// Handle click event here
// You can access the clicked item's position using getAdapterPosition()
}
}
šš» By implementing OnClickListener in the ViewHolder, we can handle click events directly within the ViewHolder class itself. Simply register the listener on the itemView in the ViewHolder constructor and handle the click event within the onClick() method.
š Is There a Better Way?
The approach you took with implementing OnClickListener in your RecyclerView.Adapter is totally fine! However, if you prefer a more modular and reusable solution, you could consider using a separate click listener interface. Here's an example:
public interface OnItemClickListener {
void onItemClick(int position);
}
Then, in your ViewHolder, you can have a setter method to bind the click listener:
public void setItemClickListener(OnItemClickListener listener) {
itemView.setOnClickListener(v -> {
if (listener != null) {
int position = getAdapterPosition();
if (position != RecyclerView.NO_POSITION) {
listener.onItemClick(position);
}
}
});
}
By using this approach, you can create different click listeners for different purposes and easily swap them out when needed. It improves code readability and makes your RecyclerView adapter class more maintainable. š
š It's Your Turn!
Now that you understand why onItemClickListener() isn't available in RecyclerView and how to handle click events, it's time to put your newfound knowledge into action! š Experiment with different techniques and find the approach that suits your needs best.
If you have any tips, tricks, or alternative solutions to share, drop them in the comments below! Let's help each other out and make RecyclerView even more awesome! šŖš
⨠Remember, learning is all about sharing, so don't forget to spread the word by sharing this blog post with your fellow developers! Together, we can make the Android development community thrive! šš²
Happy coding! šš©āš»šØāš»
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
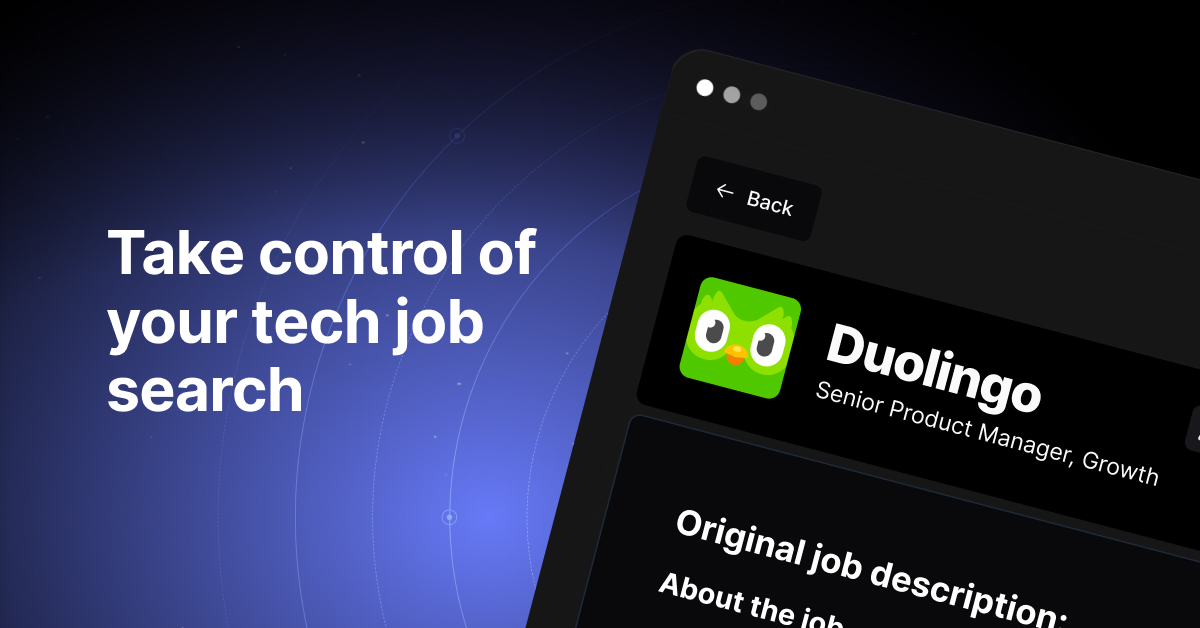