Why does my Spring Boot App always shutdown immediately after starting?
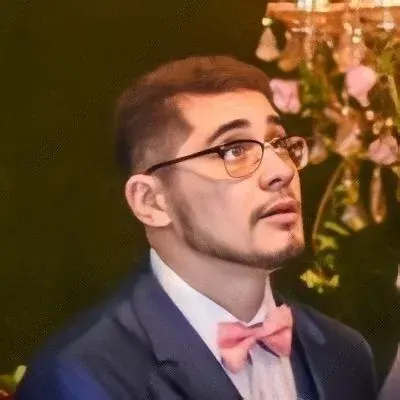
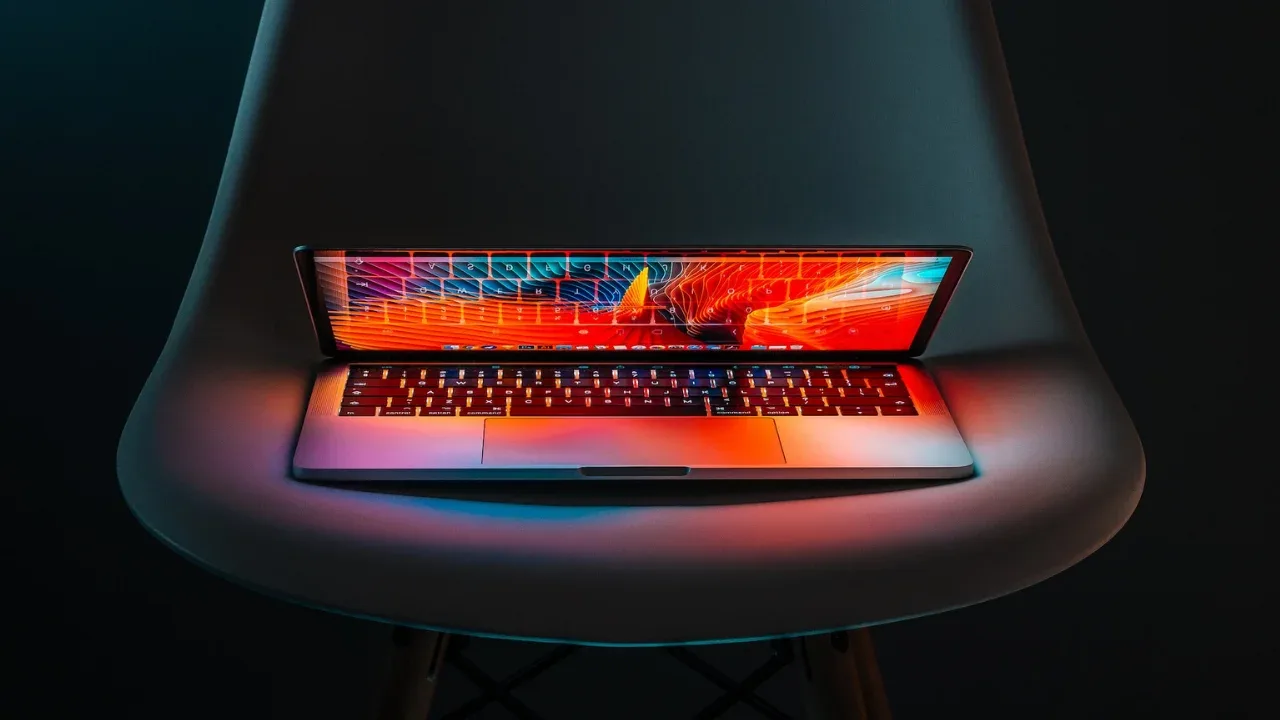
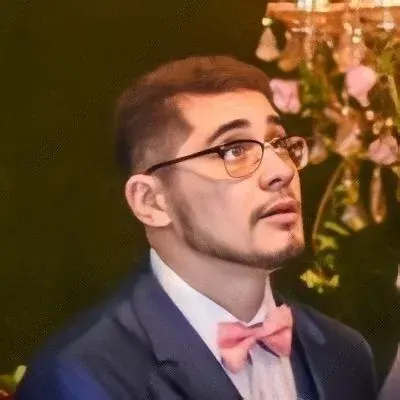
Why does my Spring Boot App always shutdown immediately after starting?
So you've just started working on your first Spring Boot application, but every time you run it, the application shuts down immediately after starting. You were expecting it to run continuously so that your web client can get some data from the browser. 😫
Don't worry, you're not alone! This is a common issue that many developers face when using Spring Boot. The good news is that there are easy solutions to fix this problem. Let's dive in and find out what might be causing your application to shut down.
The Code
Here's the code snippet of the SampleController class from your Spring Boot application:
package hello;
import org.springframework.boot.*;
import org.springframework.boot.autoconfigure.*;
import org.springframework.stereotype.*;
import org.springframework.web.bind.annotation.*;
@Controller
@EnableAutoConfiguration
public class SampleController {
@RequestMapping("/")
@ResponseBody
String home() {
return "Hello World!";
}
public static void main(String[] args) throws Exception {
SpringApplication.run(SampleController.class, args);
}
}
The Solution
The issue lies in the build.gradle file of your Spring Boot application. You need to exclude the "spring-boot-starter-tomcat" module from your dependencies.
Here's the modified build.gradle file:
dependencies {
// Exclude spring-boot-starter-tomcat
compile("org.springframework.boot:spring-boot-starter-web") {
exclude module: "spring-boot-starter-tomcat"
}
}
By excluding the "spring-boot-starter-tomcat" module, you're telling Spring Boot to use the embedded Jetty server instead of Tomcat. This should solve the problem of your application shutting down immediately after starting.
Why does this work?
The reason your application shuts down is that by default, Spring Boot starts an embedded Tomcat server. If the Tomcat server doesn't find any requests to handle, it assumes that the application is not needed and shuts down.
By excluding the "spring-boot-starter-tomcat" module and using the embedded Jetty server instead, your application will not shut down immediately because Jetty doesn't have the same behavior as Tomcat.
The Result
Now when you run your Spring Boot application, it should no longer shut down immediately. Instead, it will keep running, allowing your web client to get data from the browser.
A Word of Advice
If you encounter any other issues or have questions about Spring Boot or any other technology, don't hesitate to reach out to the community for help. Stack Overflow, GitHub, and various forums are great places to get answers and learn from experienced developers.
Remember, every problem you encounter is an opportunity to learn something new and improve your skills as a developer. Embrace the challenges and enjoy the journey!
And please, share this blog post if you found it helpful. You never know who might be facing the same issue and could benefit from this solution. Together, we can make the development community a better place! 💪
Stay curious and keep coding! 🚀