Why can"t I use switch statement on a String?
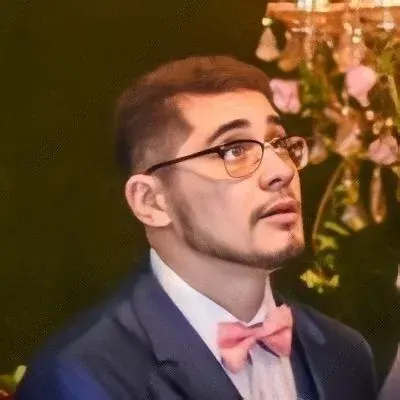
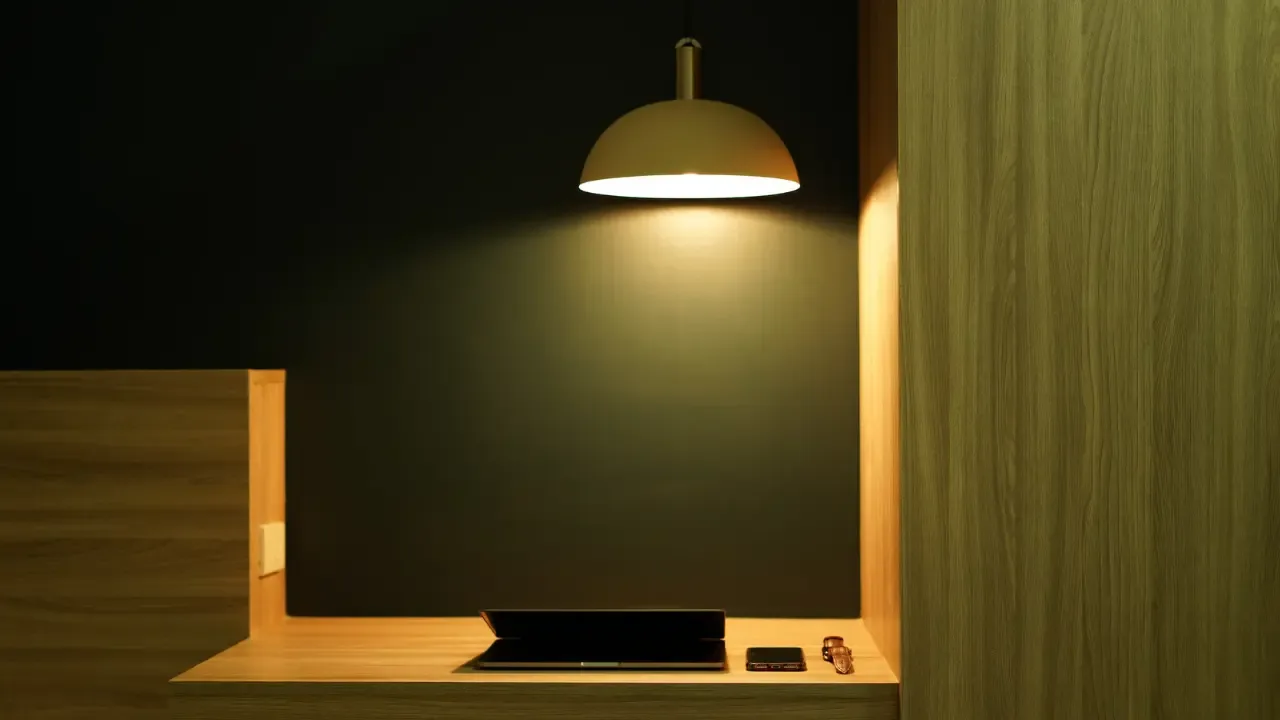
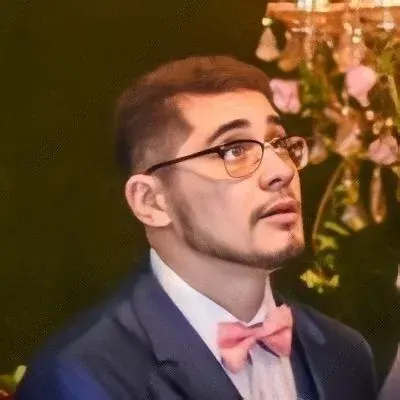
Why š I Can't Use š¤ switch
Statement on a String? š¤
š Hey there, tech enthusiasts! Are you curious about why we can't use the beloved switch
statement on a String in Java? š¤·āāļø Don't worry; I've got you covered! Let's dive into the technicalities of how the switch
statement works and uncover the reasons behind this limitation. š”
š¤ So, why the limitation? The answer lies within the inner workings of the switch
statement. Let's break it down! š
The Inside Scoop on š switch
Statement in Java
In Java, the switch
statement is designed to operate efficiently on a limited set of constant values. This makes it a powerful alternative to long and complex if-else
chains. š The switch
statement evaluates the value of an expression and compares it against a list of possible cases. When the expression matches a case, the corresponding block of code is executed. Simple, right?
The š switch
Statement and Integral Types
The switch
statement works wonderfully with integral types like int
, byte
, short
, and char
in Java. These types have a well-defined range of values. The switch
statement can quickly jump to the correct case by using an index derived from the expression value. It's like playing a game of "Guess the case" with a carefully organized list of constants. š
The š« No-Go for Strings ā
Strings, on the other hand, are non-integral objects in Java. š That means they don't have a fixed range of values like integral types do. Therefore, Java doesn't generate a compact index-based structure when you use a switch
statement on a String. Instead, the switch
statement would need to iterate over each possible case to find a matching value. š¢
This sequential search approach would be relatively slow and impractical given the flexibility and potentially vast number of Strings in Java. So, to maintain efficiency and keep your code speedier, the designers of Java decided not to allow the use of switch
on Strings. š«š¢
ā” Alternatives š”
Thankfully, Java provides alternative approaches to achieve similar functionality when you need to match a String variable. Let's take a look at two popular alternatives:
1ļøā£ if-else
Statements: Good old if-else
statements come to the rescue! You can easily compare Strings using multiple if
and else if
conditions. While this approach might be longer than using a switch
, it's reliable and offers flexibility.
if (myString.equals("case1")) {
// Handle case 1
} else if (myString.equals("case2")) {
// Handle case 2
} else if (myString.equals("case3")) {
// Handle case 3
} else {
// Handle the default case
}
2ļøā£ HashMap
: Another nifty option is to utilize the power of a HashMap
. This allows you to map each String case to its corresponding action. Using this method, you can swiftly retrieve the action associated with a particular String.
HashMap<String, Runnable> caseMap = new HashMap<String, Runnable>();
caseMap.put("case1", () -> {
// Handle case 1
});
caseMap.put("case2", () -> {
// Handle case 2
});
caseMap.put("case3", () -> {
// Handle case 3
});
Runnable action = caseMap.get(myString);
if (action != null) {
action.run();
} else {
// Handle the default case
}
š£ Engage with Us! š£
Now that you know why you can't use a switch
statement on a String in Java, it's time to put your newly acquired knowledge to use! Have you encountered any challenges while working with Strings in Java or any other programming language? Share your thoughts, experiences, and alternative solutions with us in the comments section below! Let's geek out together! š¬š¤
Remember, understanding the limitations of programming constructs is crucial in becoming a skilled developer. Keep exploring and happy coding! āØļøāØ