Where does the @Transactional annotation belong?
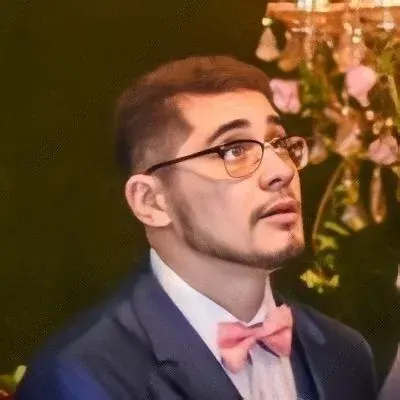
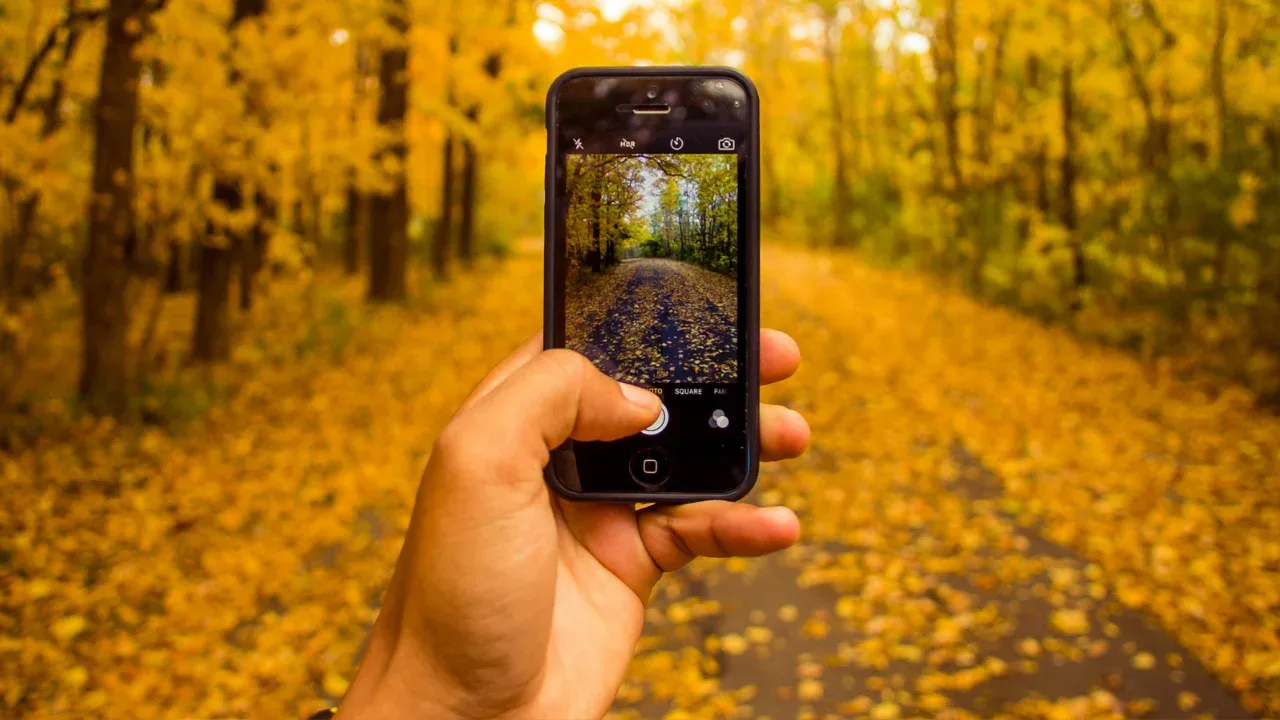
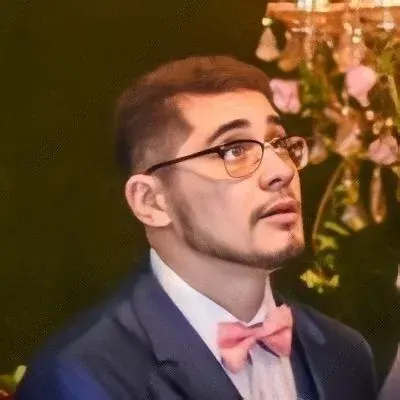
Where does the @Transactional
annotation belong? 🤔
You may have come across the @Transactional
annotation in your Java Spring projects and wondered where it should be placed. Should it be used in the DAO
classes, their methods, or the Service classes that use the DAO objects? Or maybe both? Let's dive into this common issue and find easy solutions.
😕## The Dilemma: DAO
or Service?
When working with Java Spring and JPA, it's common to separate your code into layers - the Data Access Object (DAO
) layer and the Service layer. The DAO layer is responsible for database operations, while the Service layer handles business logic. This separation helps maintain code modularity and improves maintainability.
Now, let's get back to our question. Where should the @Transactional
annotation be placed? 🤷♀️
💡## Solution 1: Annotating the DAO
Methods
One approach is to annotate the DAO methods with @Transactional
. This ensures that the database operations within each DAO method are executed in a single transaction. However, this approach can lead to some issues.
Consider an example where you have a Service method that calls multiple DAO methods. If each DAO method has its @Transactional
annotation, these methods will be executed in separate transactions. This can result in data inconsistencies and unexpected behavior.
Let's take a closer look at this example:
@Service
public class ProductService {
private final ProductDAO productDAO;
private final OrderDAO orderDAO;
@Autowired
public ProductService(ProductDAO productDAO, OrderDAO orderDAO) {
this.productDAO = productDAO;
this.orderDAO = orderDAO;
}
@Transactional
public void placeOrder(Order order) {
// business logic...
productDAO.updateProductStock(order.getProduct());
orderDAO.saveOrder(order);
// business logic...
}
}
In this example, if the updateProductStock
and saveOrder
methods in the ProductDAO
and OrderDAO
classes, respectively, are also annotated with @Transactional
, multiple transactions will be created. This can lead to potential issues when rolling back or committing the transaction.
🤷♂️## So, should we not use @Transactional
in the DAO layer at all?
Not so fast! There are cases where you might want to use @Transactional
in the DAO layer. For example, if you have a complex method that performs multiple database operations within the same transaction. But, be cautious and avoid mixing multiple transactions within a single Service method.
💡## Solution 2: Annotating the Service Layer
An alternative approach is to annotate the Service methods with @Transactional
. This allows you to control the entire business logic operation within a single transaction. By doing this, the transactions span across multiple DAO calls, ensuring data consistency.
Let's modify the previous example:
@Service
public class ProductService {
private final ProductDAO productDAO;
private final OrderDAO orderDAO;
@Autowired
public ProductService(ProductDAO productDAO, OrderDAO orderDAO) {
this.productDAO = productDAO;
this.orderDAO = orderDAO;
}
@Transactional
public void placeOrder(Order order) {
// business logic...
productDAO.updateProductStock(order.getProduct());
orderDAO.saveOrder(order);
// business logic...
}
}
In this updated code snippet, only the Service method placeOrder
is annotated with @Transactional
. This ensures that both the updateProductStock
and saveOrder
methods in the DAO layer are executed in a single transaction.
😎## One ring to rule them all
Choosing to use @Transactional
in the Service layer is generally a good practice. It provides better control over your transactions and helps maintain data integrity. However, as mentioned before, there are cases where you might still want to use it in the DAO layer for specific situations.
🏁## Wrapping Up
To summarize, the @Transactional
annotation belongs to the Service layer, where it allows you to control the transactions that involve multiple DAO operations. Annotating DAO methods individually can lead to unexpected behavior and data inconsistencies.
So, next time you come across the @Transactional
annotation, remember to use it wisely and place it where it belongs - in the Service layer. Happy coding! 💻
✨## Join the Discussion
Have you encountered issues with the @Transactional
annotation? Share your experiences and insights in the comments below. Let's learn from each other and improve our Java Spring projects together!