When using Spring Security, what is the proper way to obtain current username (i.e. SecurityContext) information in a bean?
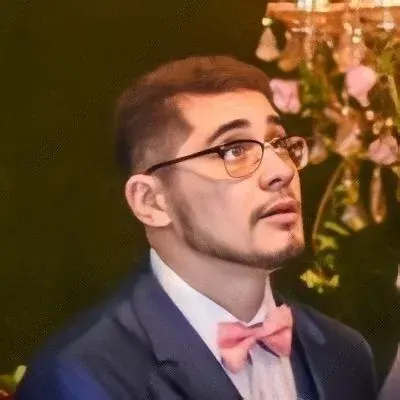
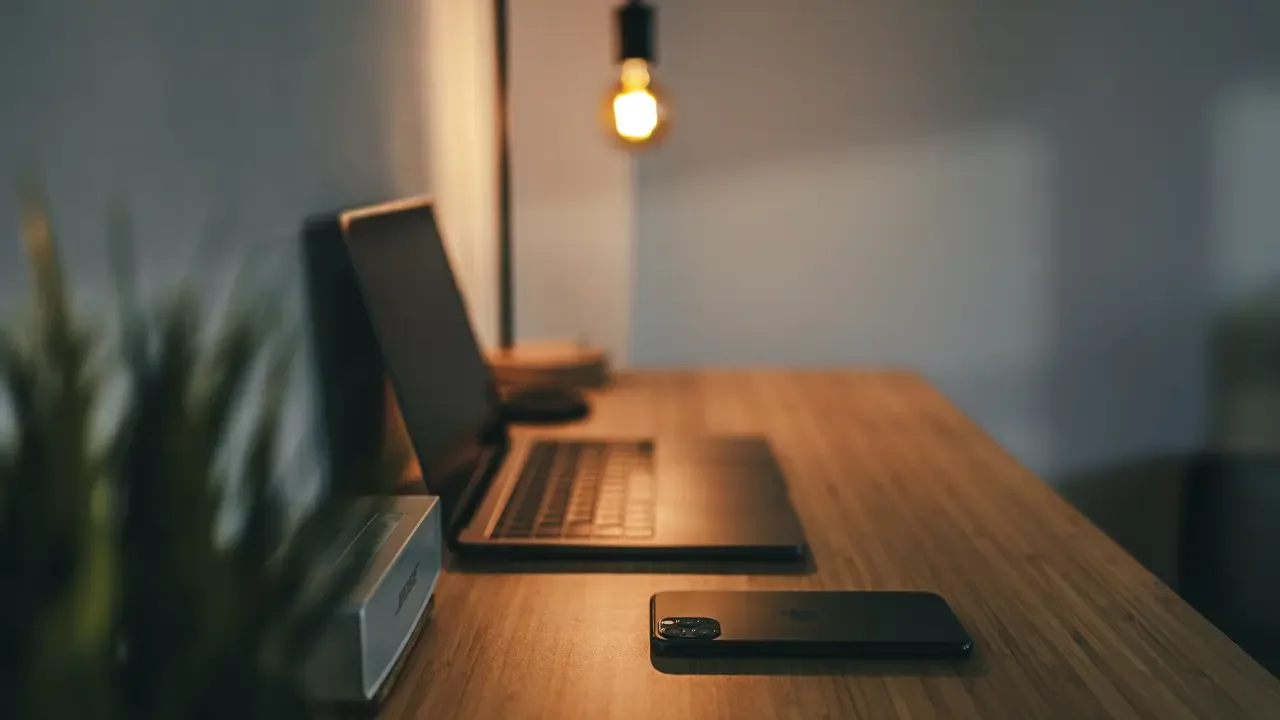
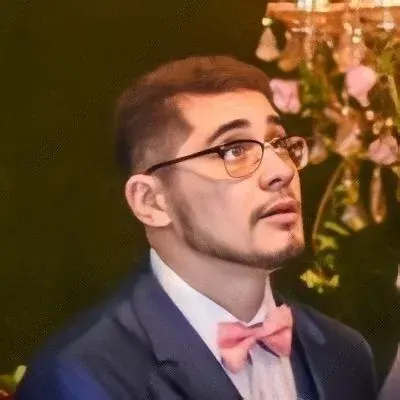
🔒⚙️ Securely Obtaining Current Username in Spring Security with BuenoSecurity 💪
Welcome to another exciting edition of the BuenoTech blog! 🥳📝 Today, we're going to explore a burning question that many Spring Security enthusiasts have: "What is the proper way to obtain current username information in a bean using Spring Security?" 🔍💡
If you're building a Spring MVC web app that utilizes Spring Security, you might have found yourself in a situation where you needed to know the username of the currently logged-in user. 🤔💻 Well, fret not! We've got you covered with an easy solution that will keep you in the good graces of Spring while effectively retrieving the current username. 🌟✅
The Dilemma: Static Methods and Spring's Essence
In the code snippet you provided, you're correctly using the SecurityContextHolder
to access the current Authentication
and retrieve the username. However, you expressed concerns about the usage of this static method within your controller. And guess what? We totally agree with you! 😉🙌
Having a static call in your controller not only defeats the purpose of Spring's dependency injection but also hinders testability and maintainability. So, let's dive into the proper way of retrieving the current username, shall we? 🙌🕵️♀️
Injecting Current Security Context: BuenoSecurity to the Rescue
🎉 Introducing BuenoSecurity 🎉—a powerful and elegant solution that will make obtaining the current username as smooth as butter. With BuenoSecurity, you can forget about static calls and configure your app to have the current SecurityContext or Authentication injected directly into your beans! 🌈🔧
To achieve this, follow these simple steps:
Add the BuenoSecurity dependency to your
pom.xml
file:
<dependency>
<groupId>com.buenotech</groupId>
<artifactId>buenosecurity</artifactId>
<version>2.0.0</version>
</dependency>
Configure BuenoSecurity in your project's configuration class:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
// Your existing security configuration goes here...
@Bean
public AuthenticationContextResolver authenticationContextResolver() {
return new BuenoSecurityContextResolver();
}
}
In your bean, simply inject the
Authentication
object:
@Autowired
private Authentication authentication;
Retrieve the current username:
String currentUser = authentication.getName();
And there you have it! 🎉 By following the above steps, you can now obtain the current username in a neatly injected way that is in line with Spring's principles and practices. It's a win-win! 🚀👏
Call-to-Action: Share Your Experience
🙌💡 BuenoTech thrives on community engagement and shared experiences. Have you faced similar challenges with Spring Security or found other creative ways to retrieve the current username? We'd love to hear from you! Share your thoughts, insights, and best practices in the comments below. Let's learn and grow together! 📣🗣️
As always, stay tuned to the BuenoTech blog for more exciting tech discussions, guides, and practical solutions. Until next time, happy coding! 💻✨