When and how should I use a ThreadLocal variable?
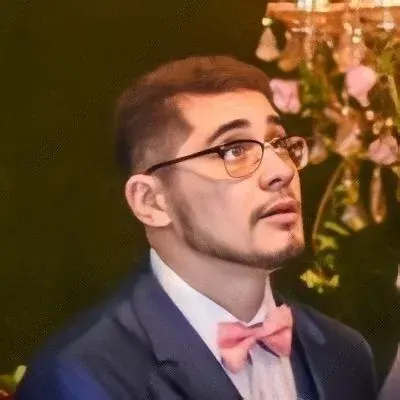
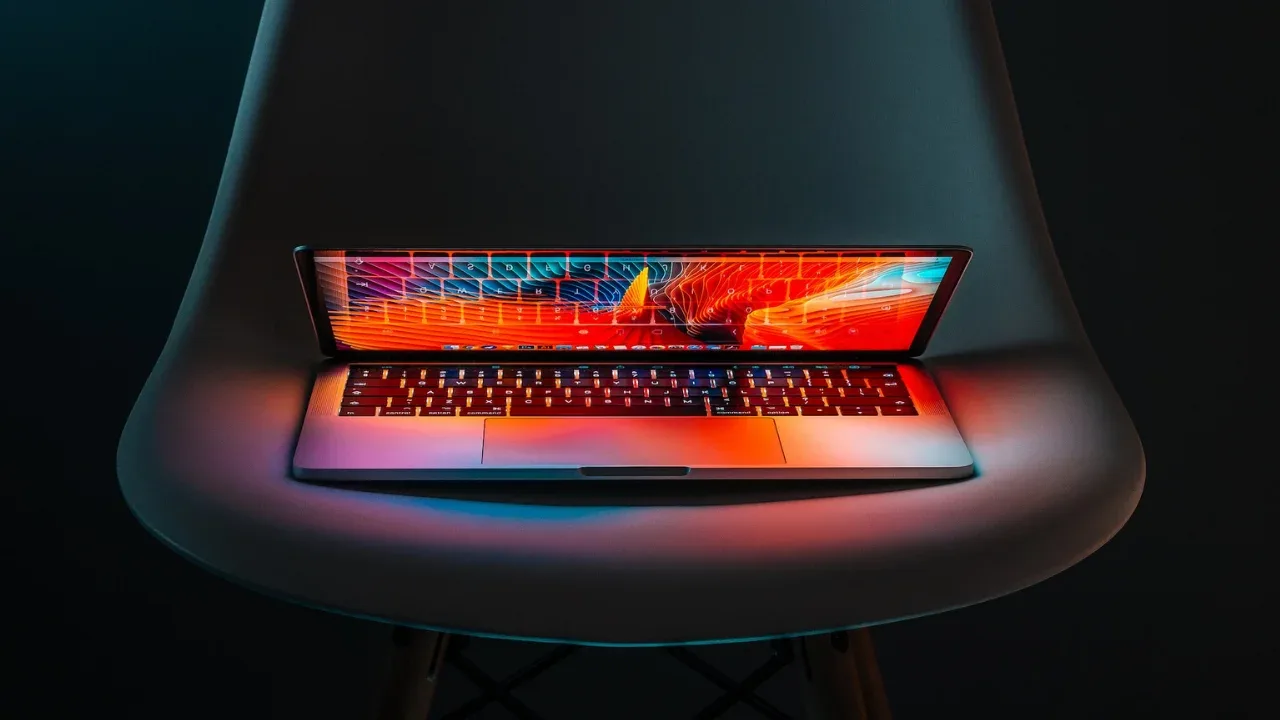
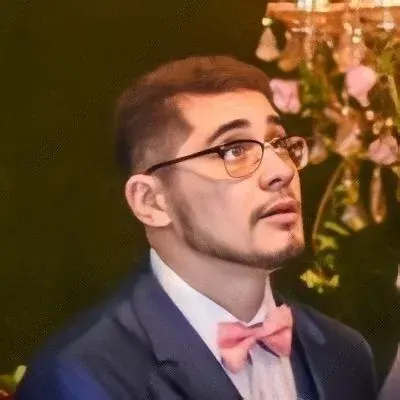
š Blog Post: When and How to Use ThreadLocal Variables
š Have you ever wondered when and how to use a ThreadLocal
variable in your Java code? š¤ Look no further! In this blog post, we'll dive into this topic to help you understand the ins and outs of ThreadLocal
variables and provide easy solutions to common issues. So let's get started!
š¤·āāļø When should I use a ThreadLocal
variable?
The ThreadLocal
class in Java allows you to create thread-local variables. These variables have different values for each thread that accesses them. This can be particularly useful in scenarios where different threads require separate instances of an object.
Here are a few situations in which you might consider using a ThreadLocal
variable:
1ļøā£ Multithreading: If you're developing a multithreaded application and need to maintain separate data or state for each thread, ThreadLocal
can come to the rescue. For example, in a web application, you might want to store the user's session data in a ThreadLocal
variable to ensure proper isolation between requests.
2ļøā£ Dependency Injection: In some cases, you might need to provide different instances of a dependency to different threads. By using ThreadLocal
, you can easily manage this situation and ensure that each thread gets its own instance of the dependency.
3ļøā£ Thread-Level Caching: ThreadLocal
variables can also be handy when you want to cache thread-specific data. For example, if your application performs expensive computations that can be reused within a thread, storing the computed result in a ThreadLocal
variable can help improve performance and reduce redundant calculations.
ā”ļø How is ThreadLocal
used?
To use a ThreadLocal
variable, follow these steps:
1ļøā£ Declare a ThreadLocal
variable: Start by declaring a ThreadLocal
variable of the desired type. For example: ThreadLocal<MyClass> myThreadLocal = new ThreadLocal<>();
2ļøā£ Initialize the ThreadLocal
variable: Assign a unique value to the ThreadLocal
variable for each thread that needs access to it. For instance: myThreadLocal.set(new MyClass());
3ļøā£ Access the ThreadLocal
variable: Retrieve the value specific to the current thread using myThreadLocal.get()
. Make sure to remove the reference to the object using myThreadLocal.remove()
when you're done to prevent memory leaks.
š Easy Solutions to Common Issues
Now, let's address some common issues you might encounter while using ThreadLocal
variables:
1ļøā£ Memory Leaks: Remember to remove the reference to the object stored in the ThreadLocal
variable using myThreadLocal.remove()
when you no longer need it. Failing to do so can lead to memory leaks.
2ļøā£ Thread Pooling: Be cautious when using ThreadLocal
with thread pools. Since the threads in a thread pool are typically reused, make sure to clean up the ThreadLocal
variables appropriately to avoid outdated or invalid data.
3ļøā£ Sharing Data Between Threads: Keep in mind that ThreadLocal
variables are not suitable for sharing data between threads. Each thread should have its own instance of the ThreadLocal
variable, and the values stored in the variable should not be modified by other threads.
š£ Get Engaged!
We hope this guide has shed light on the usage of ThreadLocal
variables and provided solutions to any issues you might encounter. Now it's your turn to engage!
š Share this blog post with your fellow developers who might find it helpful. š»š”
š¬ Tell us your experiences with ThreadLocal
variables. Have you encountered any challenges or discovered innovative use cases? Share your thoughts below in the comments section.
š Keep exploring our blog for more exciting tech tips and tricks! And remember, threading can be tricky, but with the right understanding of ThreadLocal
, you'll be threading like a pro in no time! šŖ
Happy coding! ššØāš»š