What"s the simplest way to print a Java array?
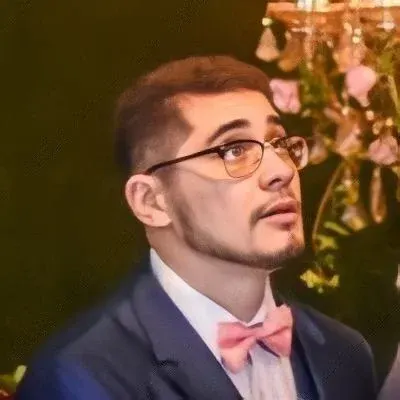
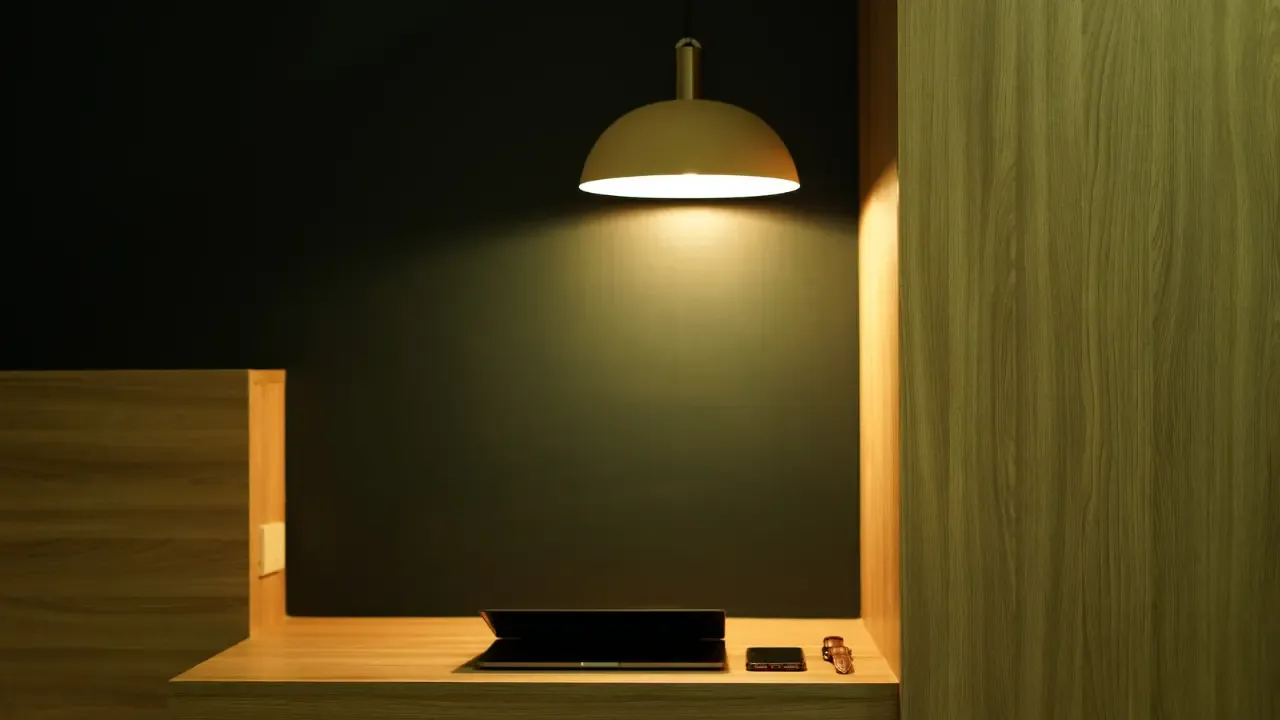
πTitle: Printing a Java Array Made Simple: Say Goodbye to the Hash!
Introduction: Hey there tech enthusiasts! π Are you tired of seeing the obscure hash code when trying to print a Java array directly? Well, you're not alone! Whether you're dealing with an array of primitives or object references, we've got you covered. In this blog post, we'll explore the simplest way to print a Java array and bid farewell to the cryptic hash. Let's dive in! π»
The Problem: Array Printing Woes π£
You may have noticed that when you try to print a Java array directly, you're greeted with something like className@hashCode
. Not very helpful, is it? Let's take an example:
int[] intArray = new int[] {1, 2, 3, 4, 5};
System.out.println(intArray); // Prints something like '[I@3343c8b3'
Yikes! That's definitely not what we want. But fear not, for there is a simpler solution! π
The Simple Solution: Arrays.toString() to the Rescue! π
The simplest way to print a Java array is by using the Arrays.toString()
method. This handy method is available in the java.util
package and saves us from the hassle of manually converting the array elements to a human-readable format. Let's take a look at how it works:
int[] intArray = new int[] {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(intArray)); // Output: [1, 2, 3, 4, 5]
Voila! π© With just a single line of code, our array is printed in a clean and understandable format. But wait, there's more to it!
Handling Arrays of Object References π
The Arrays.toString()
method not only works for arrays of primitives but also handles arrays of object references seamlessly. Let's take an example:
String[] strArray = new String[] {"John", "Mary", "Bob"};
System.out.println(Arrays.toString(strArray)); // Output: [John, Mary, Bob]
Oh, the joy of simplicity! π Our array of names is now beautifully displayed without any hassle. Isn't that what we all want? π
Engage with Us! π£
We hope this simple guide has helped you conquer the challenge of printing Java arrays. But don't stop here! Feel free to share your own experiences, ask questions, or suggest alternative solutions in the comments section below. Let's geek out together! π€
Remember, simplicity is key. Say goodbye to the hash and embrace the elegance of Arrays.toString()
. Happy coding, my fellow tech aficionados! β¨
Conclusion:
Printing a Java array doesn't have to be complicated. By using Arrays.toString()
, you can effortlessly display arrays of both primitives and object references in a readable and concise format. No more cryptic hashes, just pure simplicity. Give it a try, and let us know how it goes!
Keep coding, keep learning, and stay tuned for more tech adventures! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
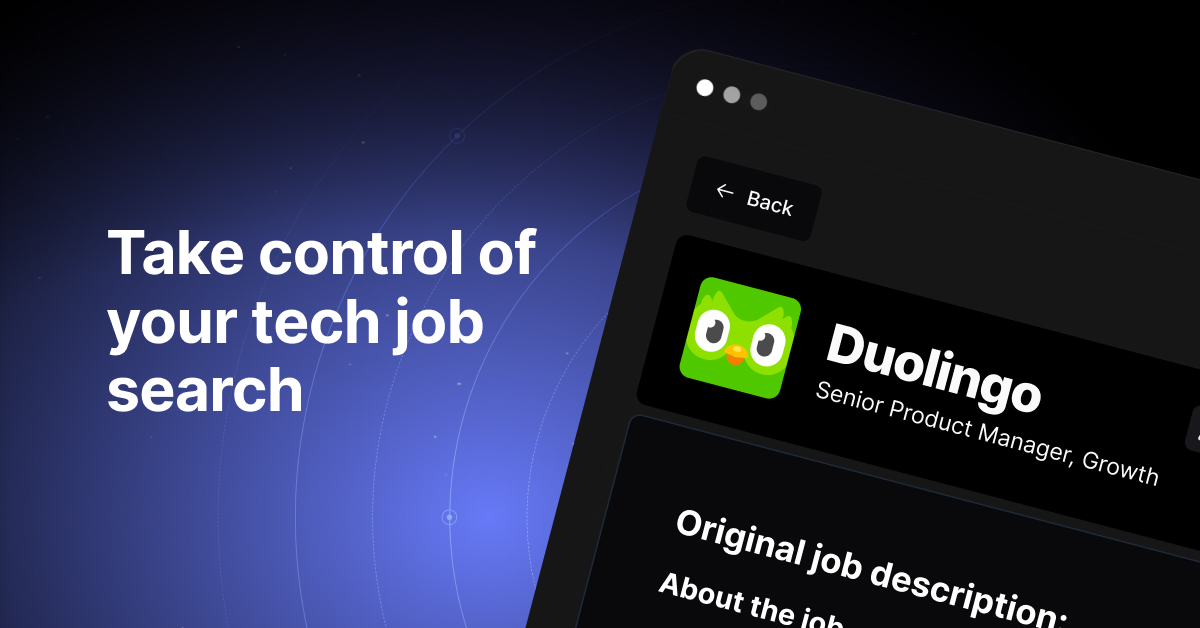