What"s the difference between Spring Data"s MongoTemplate and MongoRepository?
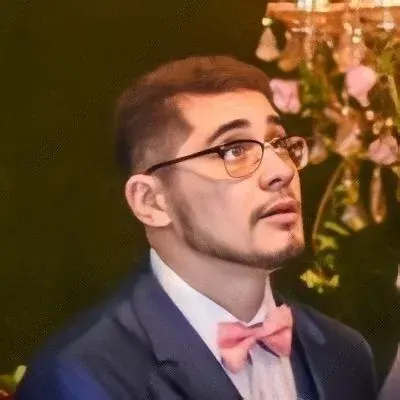
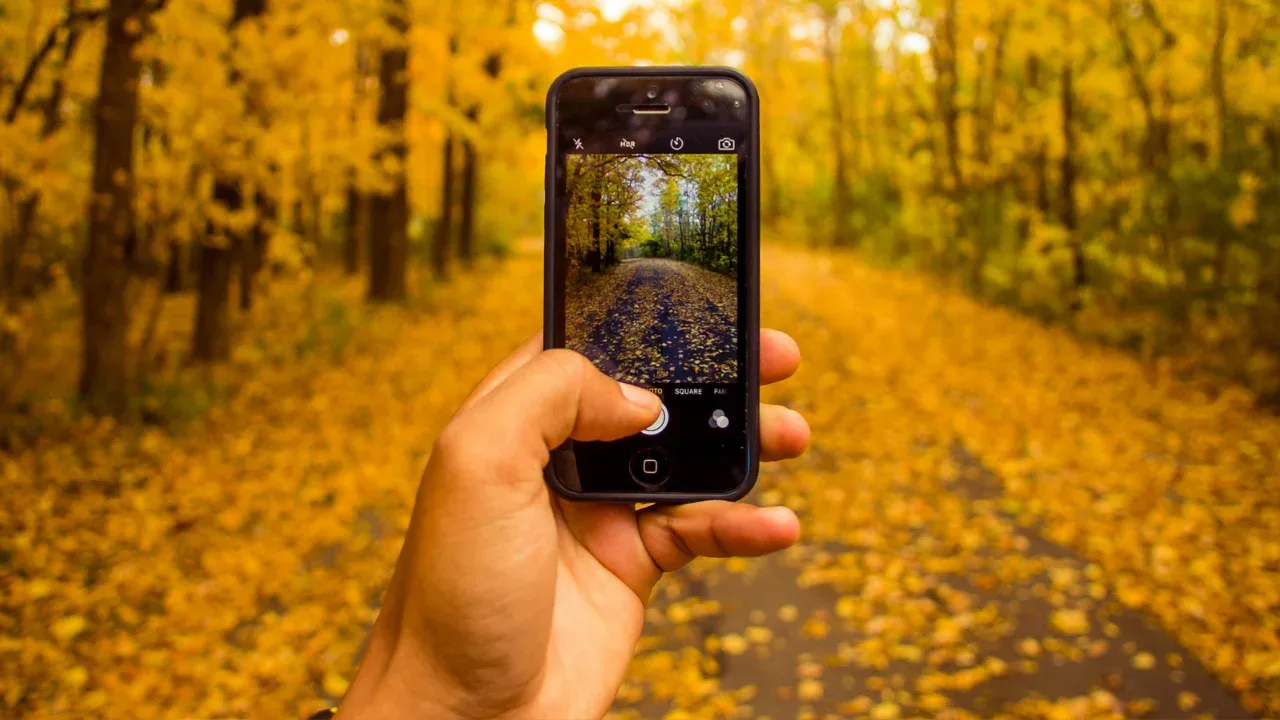
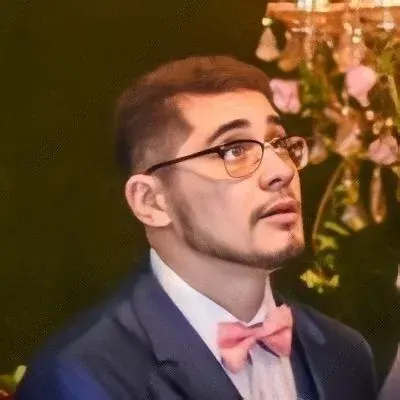
Understanding Spring Data's MongoTemplate and MongoRepository: Which One Should You Use?
So you're developing an application using Spring Data and MongoDB, and you've come across two options: MongoTemplate and MongoRepository. π€
You're not alone in wondering which one is more convenient and powerful to use. Many developers struggle to find examples or understand the syntax when dealing with complex queries. But fear not! π¦ΈββοΈ In this blog post, we'll dive into the differences between MongoTemplate and MongoRepository and help you choose the right one for your project.
The Basics: What Are MongoTemplate and MongoRepository?
Both MongoTemplate and MongoRepository are part of the Spring Data MongoDB library. They provide abstractions for interacting with MongoDB, making it easier to perform database operations in a Spring application.
MongoTemplate
MongoTemplate is a powerful class that allows you to perform any MongoDB operation directly. It gives you full control over the queries you write and the data you manipulate. You can think of it as the low-level API for working with MongoDB in Spring Data.
MongoRepository
On the other hand, MongoRepository is a higher-level abstraction on top of MongoTemplate. It follows the repository pattern and provides a set of predefined methods for common database operations such as CRUD (Create, Read, Update, Delete). With MongoRepository, you can eliminate a lot of boilerplate code and focus more on your application's logic.
When to Use MongoTemplate?
MongoTemplate is a great choice when you need ultimate flexibility and control over your queries. It allows you to write custom queries using various MongoDB query methods, criteria, or even raw JSON queries. You can handle complex scenarios and leverage MongoDB's advanced features.
Let's take a look at an example:
@Repository
public interface UserRepository extends MongoRepository<User, String> {
List<User> findByEmailOrLastName(String email, String lastName);
}
In this case, you can see that we're using a predefined method from MongoRepository to search for users based on their email or last name. But what if you have more complex requirements that can't be expressed easily with predefined methods? That's where MongoTemplate shines.
@Repository
public class UserRepositoryImpl implements UserRepositoryCustom {
private final MongoTemplate mongoTemplate;
public UserRepositoryImpl(MongoTemplate mongoTemplate) {
this.mongoTemplate = mongoTemplate;
}
public List<User> findByCustomCriteria(String criteria) {
Query query = new Query();
// Perform your custom query operations using the MongoTemplate API
// ...
return mongoTemplate.find(query, User.class);
}
}
By implementing a custom repository interface (e.g., UserRepositoryCustom
), you can use MongoTemplate to perform advanced queries tailored to your specific needs.
When to Use MongoRepository?
MongoRepository becomes handy when you have straightforward CRUD operations or standard queries. It offers a range of predefined methods like findById
, findAll
, save
, and delete
, which cover the majority of common scenarios. Using MongoRepository, you can drastically reduce boilerplate code and focus on building your application's business logic.
For instance, let's say you want to search for users based on a search string that matches their first name, last name, or email. Here's an example of how you can achieve this using MongoRepository:
@Repository
public interface UserRepository extends MongoRepository<User, String> {
List<User> findByEmailOrFirstNameOrLastNameLike(String searchText);
}
MongoRepository automatically generates the query based on the method name, saving you from writing complex and error-prone queries manually.
Which One Should You Choose?
Choosing between MongoTemplate and MongoRepository depends on your project's requirements and complexity. Here's a summary to help you decide:
Use MongoTemplate when you need ultimate flexibility, want to leverage advanced MongoDB features, or require complex query operations beyond what predefined methods offer.
Use MongoRepository when you have standard CRUD operations or common query scenarios covered by predefined methods. It helps reduce boilerplate code and provides a cleaner, more maintainable codebase.
Remember, you can always mix and match both approaches in the same project. If you find yourself outgrowing the predefined methods, you can extend your MongoRepository with a custom implementation backed by MongoTemplate.
Wrapping Up
Understanding the difference between Spring Data's MongoTemplate and MongoRepository is crucial when working with MongoDB. By choosing the right approach for your project, you can ensure better productivity, maintainability, and code readability.
So go ahead and explore the power of MongoTemplate or embrace the simplicity of MongoRepository. And if you still need more clarity or have specific queries, don't hesitate to reach out and engage with the community. Happy coding! π