What"s the difference between SoftReference and WeakReference in Java?
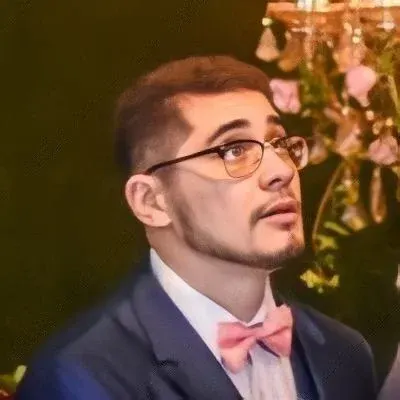
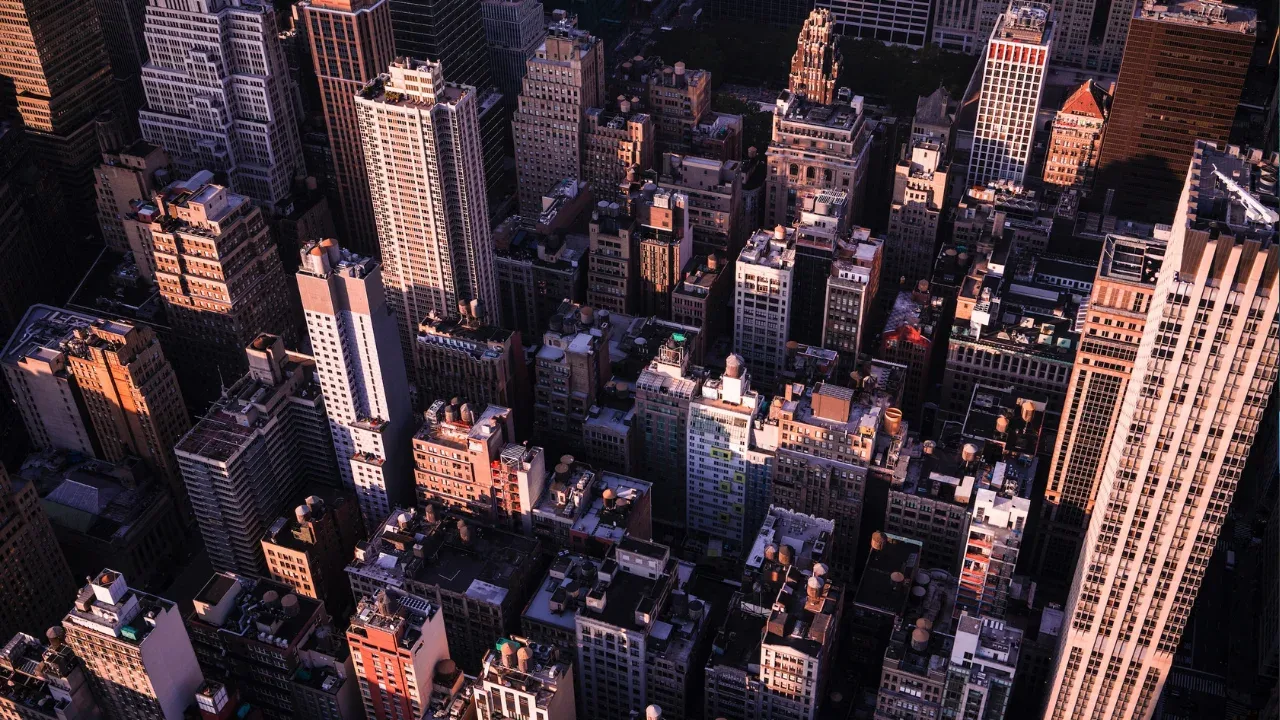
š What's the Difference Between SoftReference and WeakReference in Java?
Are you a Java enthusiast trying to wrap your head around the difference between java.lang.ref.WeakReference
and java.lang.ref.SoftReference
? š¤ It's time to put an end to your confusion! In this blog post, we'll demystify these concepts and explore their distinct functionalities. Let's dive right in! š”
Understanding the Basics š
Both WeakReference
and SoftReference
are part of the java.lang.ref
package and are used for handling references to objects in Java. The primary objective is memory management and garbage collection.
šŖļø WeakReference: A Delicate Thread
The WeakReference
class, as the name implies, maintains a weak reference to an object. It means that the reference is weakly held, making the object eligible for garbage collection even if the reference exists. š®
So, why would we use WeakReference
? š¤ The main use case is when we want to prevent an object from being held in memory and cause a memory leak. For example, imagine you have a cache that stores key-value pairs, and you want the values to be garbage collected when they're no longer in use. That's where WeakReference
comes to the rescue! š¦ø
Let's take a quick look at some code:
private Map<Key, WeakReference<Value>> cache = new HashMap<>();
public void addToCache(Key key, Value value) {
cache.put(key, new WeakReference<>(value));
}
public Value getFromCache(Key key) {
WeakReference<Value> weakRef = cache.get(key);
return weakRef != null ? weakRef.get() : null; // Access the value using get()
}
In the code snippet above, the values stored in the cache can be collected by the garbage collector if they're no longer strongly referenced anywhere else in the application. Easy, right? š
ā” SoftReference: A Gentle Grip
Unlike the WeakReference
, the SoftReference
class provides a slightly stronger reference. Objects referenced by SoftReference
are eligible for garbage collection when the JVM is running out of memory. This makes it perfect for implementing memory-sensitive caches or caches with eviction policies based on memory usage. š
Here's an example to get you started:
private Map<Key, SoftReference<Value>> cache = new HashMap<>();
public void addToCache(Key key, Value value) {
cache.put(key, new SoftReference<>(value));
}
public Value getFromCache(Key key) {
SoftReference<Value> softRef = cache.get(key);
return softRef != null ? softRef.get() : null; // Access the value using get()
}
As you can see, using SoftReference
allows your cache to retain values until memory becomes scarce. This ensures that important data stays in memory as long as possible. šļø
Which One to Use? š¤
Determining whether to use WeakReference
or SoftReference
depends on your specific requirements. Ask yourself the following questions:
š¹ Do I need the object to be cleared immediately when there are no strong references? Use WeakReference
.
š¹ Do I want the object to stick around until memory becomes a concern? Use SoftReference
.
Remember, both WeakReference
and SoftReference
provide elegant solutions to memory-related problems in Java. It's essential to assess your needs and choose the one that aligns with your application requirements. š§©
Time to Debrief š
Now that you understand the difference between WeakReference
and SoftReference
, you can apply this knowledge to write more efficient and memory-friendly Java programs. šŖ
So, next time you're implementing a cache, think about whether you need a delicate thread (WeakReference
) or a gentle grip (SoftReference
). Your memory management skills will surely impress! š
š Engage With Us! š£
We hope this blog post clarified the nuances between WeakReference
and SoftReference
in Java. If you found it helpful, don't hesitate to share it with your fellow developers. Also, we'd love to hear your thoughts on this topic! Let us know in the comments section below. šš¬
Stay tuned for more engaging tech content from us. Until then, happy coding! š»š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
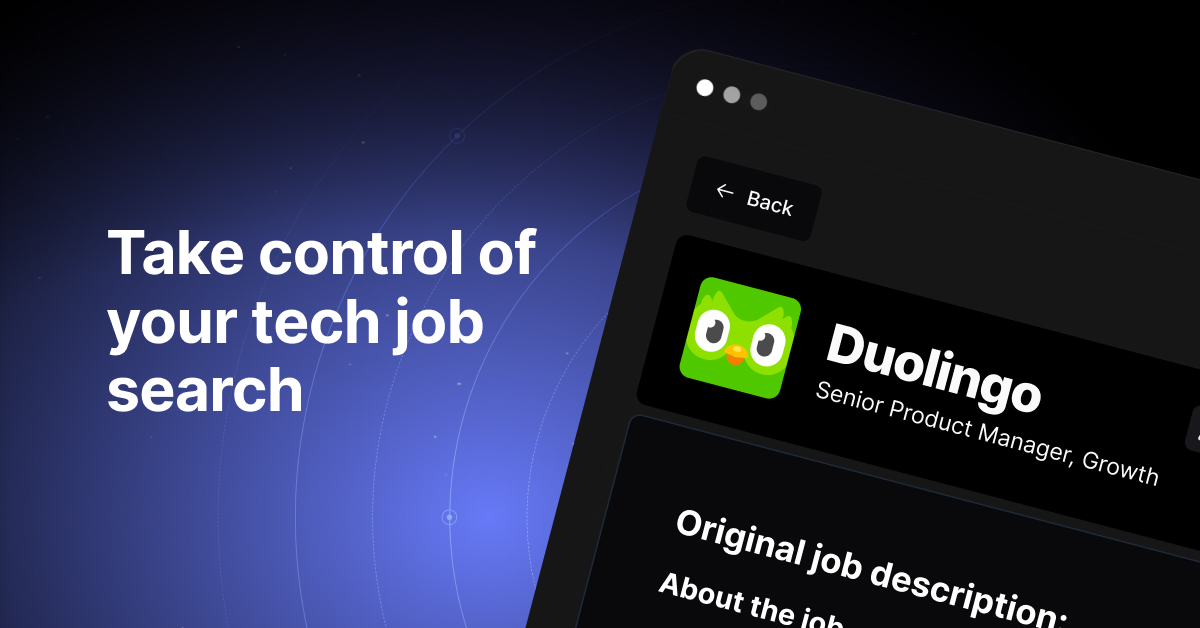