What"s the difference between @Component, @Repository & @Service annotations in Spring?
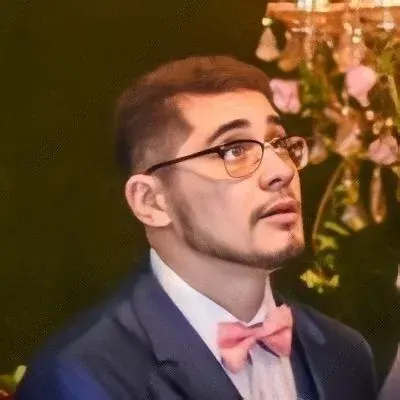
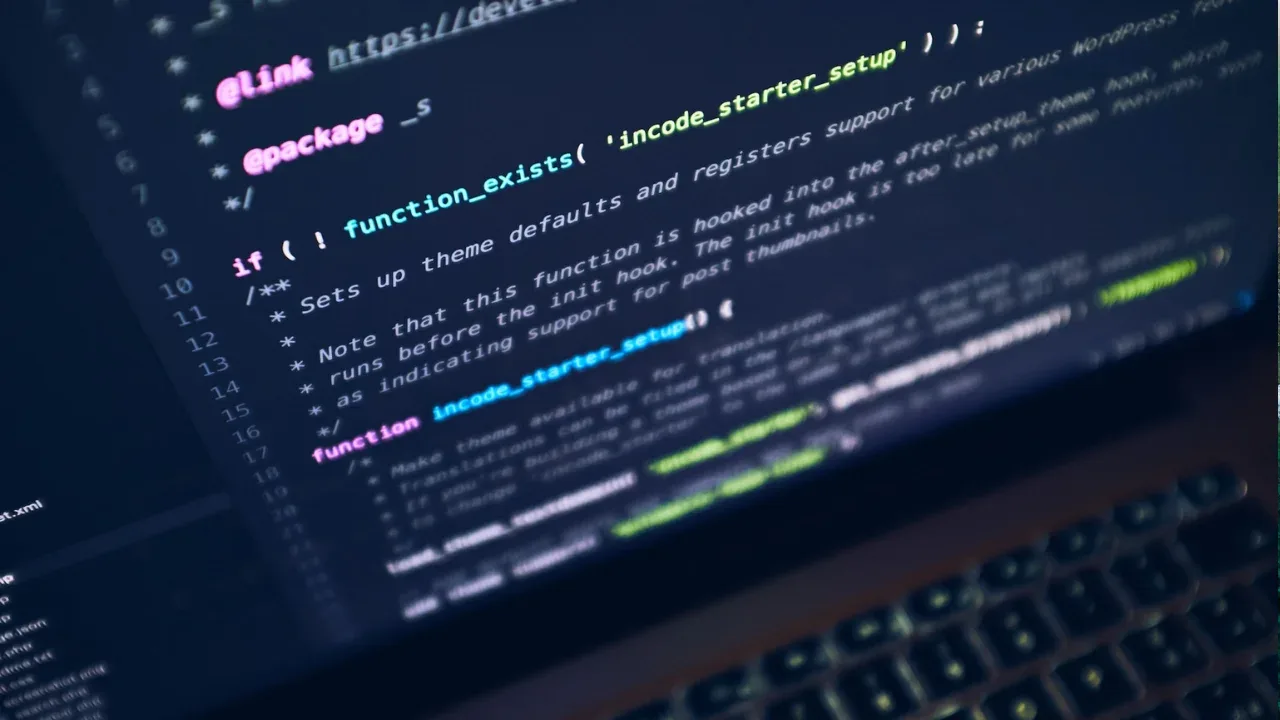
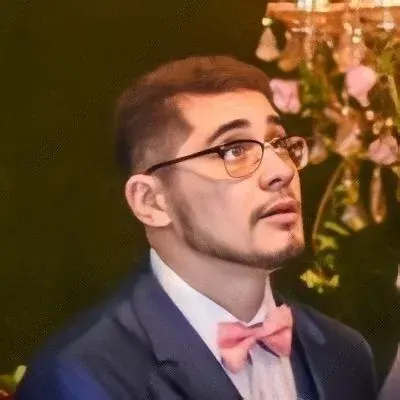
🌟🌟🌟 Welcome to my tech blog! Today, we're going to dive into the exciting world of Spring annotations. 🌟🌟🌟
If you've ever worked with Spring, you've probably seen annotations like @Component
, @Repository
, and @Service
floating around your code. But have you ever wondered what sets them apart? Do they really make a difference, or are they just fancy notations? 🤔
Well, my dear reader, let me enlighten you on this matter! Let's break it down and explore the unique characteristics of each annotation:
The Magic of @Component 🎩
The @Component
annotation is the foundation of all Spring-managed components. It identifies a class as a Spring bean, allowing it to be automatically detected and registered in the application's ApplicationContext. Think of it as a general-purpose annotation.
Here's an example:
@Component
public class MyComponent {
// Your awesome code here
}
The Charm of @Repository 📚
The @Repository
annotation is a specialization of @Component
. It's primarily used to define a class as a repository, representing the persistence layer of your application. Spring applies additional exception translation to @Repository
beans, making it easier to work with database-related operations.
For instance:
@Repository
public class MyRepository {
// Your database-related code here
}
The Power of @Service ⚡
Now, let's talk about the @Service
annotation. It's also a specialization of @Component
and has a specific purpose. It serves as a marker for classes that contain business logic or perform certain services within your application. It adds an extra layer of meaning and clarity to your code.
Check out this example:
@Service
public class MyService {
// Your business logic here
}
So, What's the Difference? 🤔
While all three annotations serve as stereotypes for Spring beans, they have their own unique characteristics. Changing from one annotation to another may not break your code, but it's important to understand their intended purposes:
Use
@Component
for general-purpose Spring beans.Use
@Repository
for classes that interact with your database.Use
@Service
for classes that provide business logic or perform specific services.
Remember, these annotations make your code more expressive and help fellow developers (including your future self) understand the role of each class in your application. 😎
Conclusion and Call-to-Action 🎉
Now that you're armed with the knowledge of the differences between @Component
, @Repository
, and @Service
annotations, it's time to put them into practice in your Spring projects! Remember, choosing the right annotation enhances code readability and maintainability.
If you found this blog post helpful, why not share it with your fellow Spring enthusiasts? 🌼✉️
Leave a comment below to let me know your thoughts and experiences with these annotations. Have you ever encountered any challenges or interesting use cases? I'd love to hear from you! 👇🗨️
Thanks for reading, and happy coding! 💻🚀