What is the volatile keyword useful for?
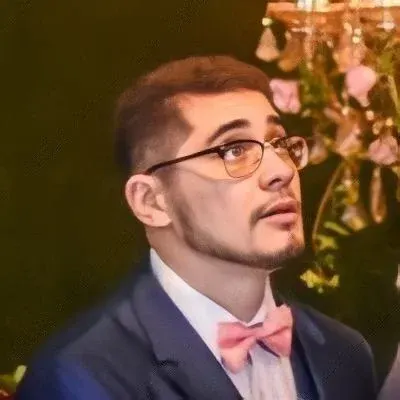
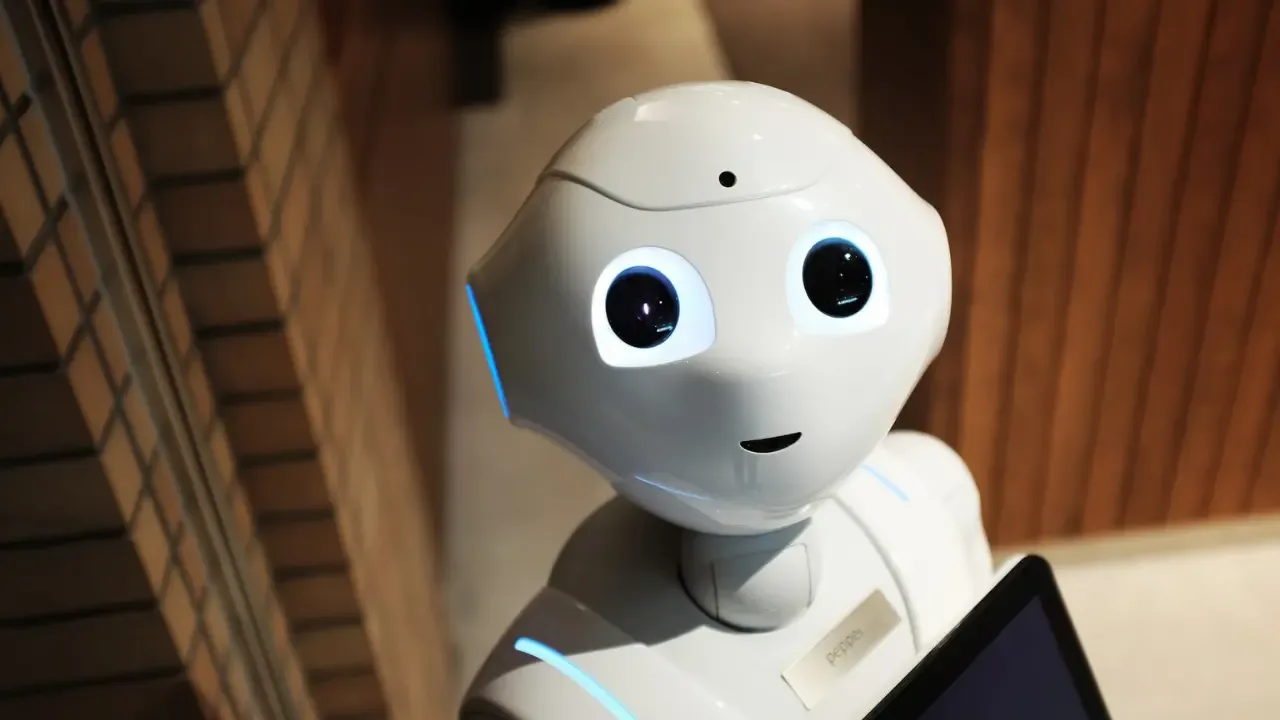
🔐💥 Understanding the Volatile Keyword: Protecting Data in Java
Have you ever encountered the mysterious "volatile" keyword in Java and wondered what it's all about? Today, I stumbled upon it at work and, like many developers, found myself scratching my head. But fear not, my fellow tech enthusiasts! I did some digging and found a gem of an article that explains the intricacies of this keyword.
🧐 What is the Volatile Keyword?
In Java, the "volatile" keyword is used to indicate that a variable's value might be modified by multiple threads simultaneously. It serves as a hint to the JVM (Java Virtual Machine) that certain optimizations should not be applied to that variable. In other words, it ensures visibility and atomicity when dealing with shared variables.
🌪️ The Problem: Thread Interference & Memory Consistency Errors
Without the "volatile" keyword, there is a chance that different threads accessing the same variable may end up with outdated or inconsistent values. This is known as thread interference and can lead to subtle bugs that are notoriously difficult to debug. 😱
Imagine a scenario where two threads are trying to increment a variable simultaneously. Without proper synchronization, they might overwrite each other's changes, resulting in incorrect values. 😫
🔑 The Solution: Using the Volatile Keyword
By declaring a variable as "volatile," you ensure that any changes to it are immediately visible to all threads. This eliminates the possibility of thread interference and guarantees memory consistency. Sounds like magic, doesn't it? ✨
Here's an example to illustrate its usage:
public class Counter {
private volatile int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In this simplified counter class, the "count" variable is declared as volatile. This guarantees that when multiple threads call the increment()
method, they will always see the latest value of "count" and avoid any interference.
💡 When to Use the Volatile Keyword
While the volatile keyword solves the problem of thread interference, it's important to note that it does not handle complex operations involving multiple steps (e.g., reading and writing a variable together). For such scenarios, other synchronization mechanisms like locks or atomic classes should be used.
You should consider using the volatile keyword when:
A variable is accessed by multiple threads without any locks or synchronization.
The variable's value is updated frequently, and thread interference is a concern.
🚀 Your Call-To-Action: Share Your Experiences
Now that you've grasped the concept of the volatile keyword, it's time to put your newfound knowledge to the test! Have you ever used the volatile keyword in your projects, or can you imagine a scenario where it would be useful? Share your thoughts and experiences in the comments below. Let's learn from each other and foster a vibrant tech community! 💪😊
📚 Reference: IBM DeveloperWorks - Understanding Java's volatile keyword
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
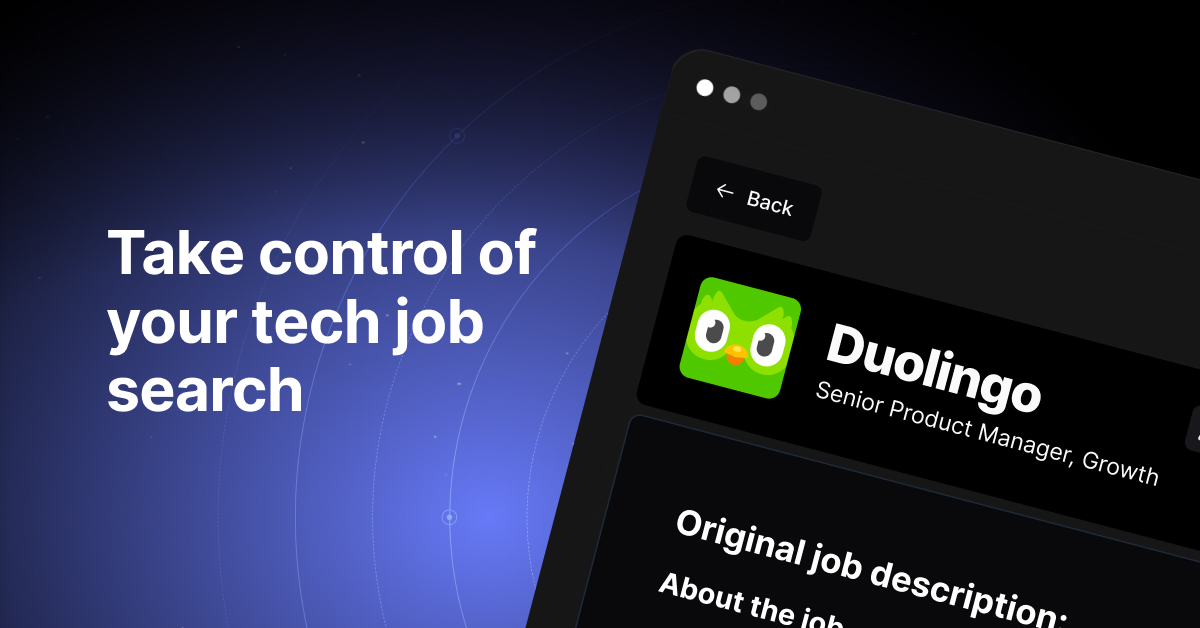