What is the proper way to re-attach detached objects in Hibernate?
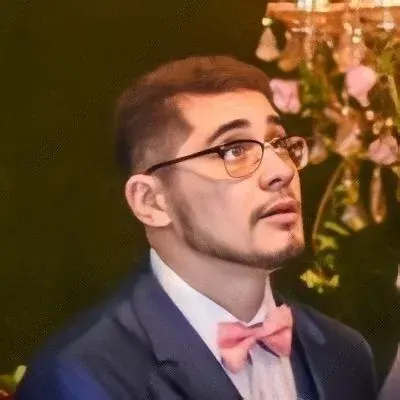
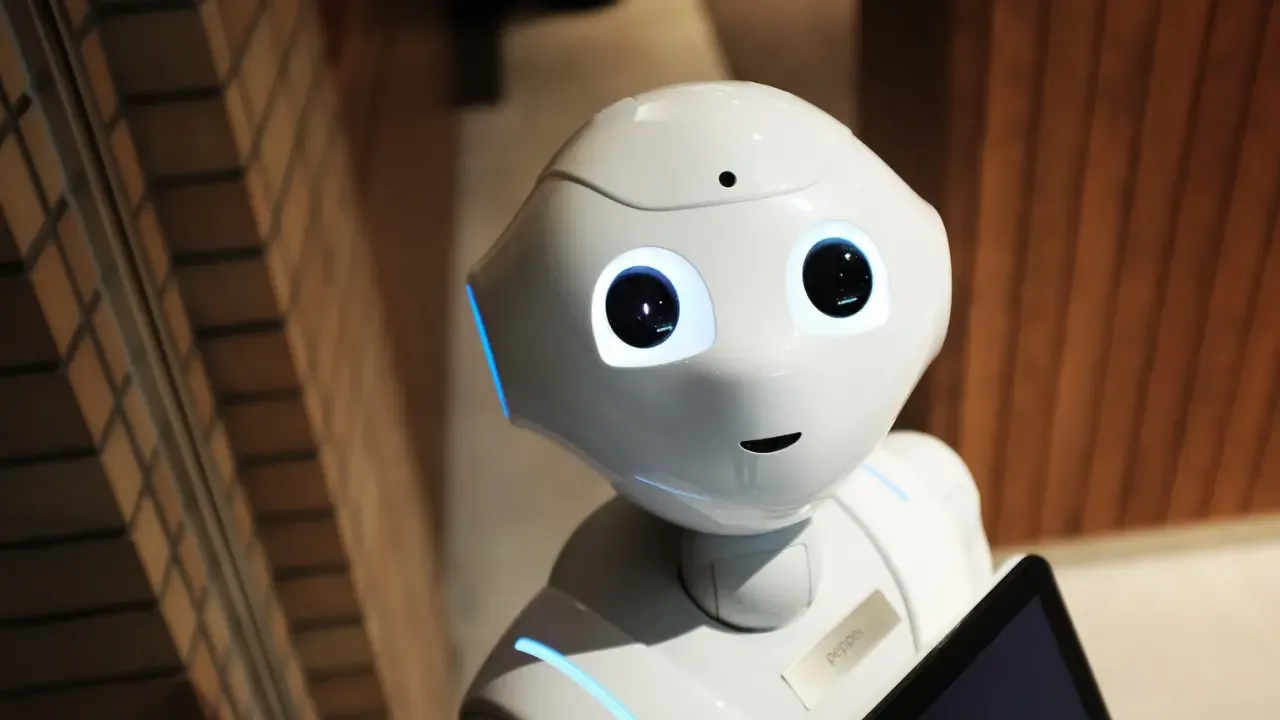
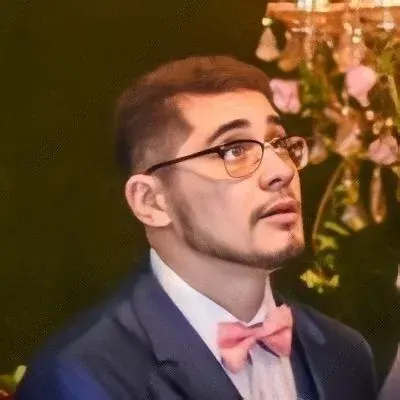
The Proper Way to Re-attach Detached Objects in Hibernate
š¤ Have you ever encountered a situation in Hibernate where you need to re-attach detached objects to a session, but you're facing errors because an object with the same identity already exists? š« Don't worry, we've got you covered! In this blog post, we'll address this common issue and provide you with easy solutions. Let's get started! šŖ
The Problem: Detached Objects and Existing Objects
First, let's understand the problem at hand. When a Hibernate object is detached from a session (i.e., no longer associated with a session), and you try to re-attach it to another session, conflicts may arise if an object with the same identity already exists in that session. š¬
Solution 1: update(obj)
- Only if Object Doesn't Exist
One way to re-attach detached objects is by using the update(obj)
method provided by Hibernate. This method works perfectly fine š but only when an object with the same identity doesn't already exist in the session. š« If it does, exceptions are thrown, and you won't get the desired result.
Solution 2: merge(obj)
- Only if Object Exists
Another option is to use the merge(obj)
method. This method is useful when the object already exists in the session. š However, if the object hasn't been loaded into the session yet, exceptions will be thrown when you try to access it later. š
The Elegant Solution: Re-attach Sessions to Objects Generically
Now, let's explore a more elegant and generic solution to this problem. Instead of relying on exceptions to control the flow of our code, we can use a combination of both update(obj)
and merge(obj)
methods. š¤ Here's how:
Check if the object exists in the session using
getSession().contains(obj)
.If the object is already in the session, use
update(obj)
to re-attach it.If the object isn't in the session, use
merge(obj)
to add it.
By following these steps, you can ensure that the object is properly re-attached to the session without any conflicts or exceptions. š
Session session = getSession();
if (session.contains(obj)) {
session.update(obj);
} else {
session.merge(obj);
}
Your Turn: Engage and Share Your Thoughts
We hope this guide has helped you understand the proper way to re-attach detached objects in Hibernate. Now, it's your turn! š¬ Share your thoughts, experiences, or any other solutions you've come across in the comments below. Let's learn from each other and make Hibernate even better! š
Remember, if you found this post helpful, don't hesitate to share it with your fellow developers and spread the knowledge. Together, we can overcome any Hibernate obstacles! š
So, what are you waiting for? Get back to coding, re-attach those objects like a pro, and share your success with us! š»šŖ
Happy Hibernate coding! šāØ