What is the Java equivalent for LINQ?
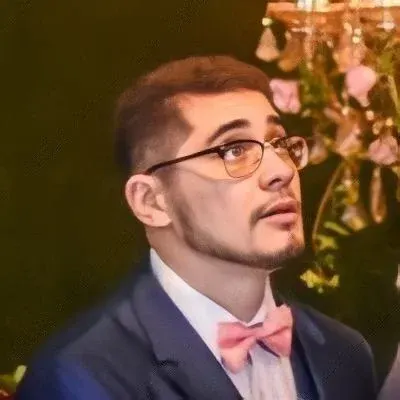
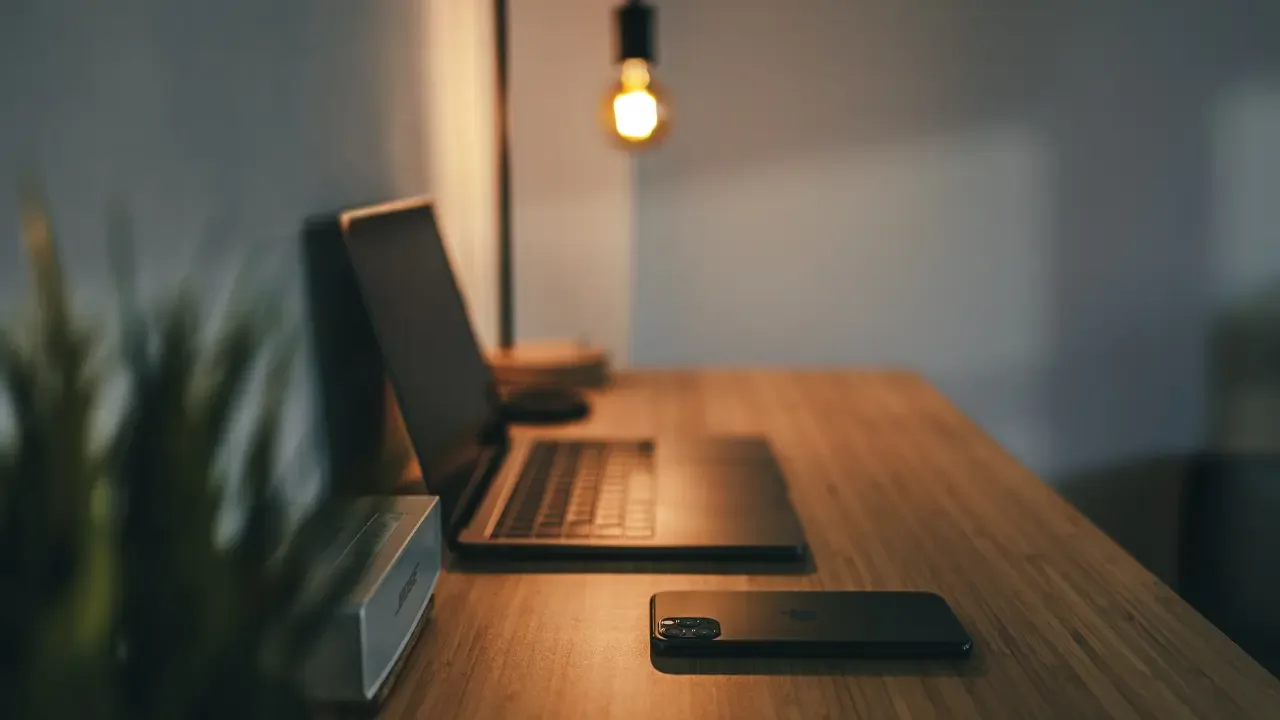
💡 What is the Java equivalent for LINQ?
If you're a Java developer and have come across the term LINQ, you might be wondering what its equivalent is in the Java world. LINQ, or Language Integrated Query, is a powerful feature in .NET that allows developers to query and manipulate data using a familiar syntax. Unfortunately, Java doesn't have a direct equivalent to LINQ, but fear not! In this blog post, we'll explore some alternatives and workarounds to achieve similar functionality in Java.
🔄 The Alternatives
While Java doesn't have a built-in language feature like LINQ, there are libraries available that can help you achieve similar results. Let's take a look at a couple of popular options:
1️⃣ Stream API
The Stream API introduced in Java 8 provides functional-style operations on streams of elements. It allows you to perform filter, map, reduce, and other operations on collections or data streams. Here's a simple example:
List<String> names = Arrays.asList("John", "Jane", "Mike");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
In this example, we filter the names
list to only include names starting with "J". The resulting filteredNames
list will contain ["John", "Jane"].
2️⃣ Querydsl
Querydsl is a comprehensive library that provides a fluent API for querying SQL, JPA, and other data sources in a type-safe manner. This library allows you to write queries using its DSL (Domain-Specific Language) that closely resembles the LINQ syntax. Here's a simple example using Querydsl and JPA:
QUser user = QUser.user;
List<User> users = new JPAQueryFactory(entityManager)
.selectFrom(user)
.where(user.age.between(20, 30))
.fetch();
In this example, we select users from the database where their age is between 20 and 30. The resulting users
list will contain the matching entities.
🤝 The Call-to-Action
While these alternatives provide similar functionality to LINQ, it's important to note that they have their own learning curves and might not cover all the aspects of LINQ. If you're specifically looking for LINQ-like features, you might want to consider exploring .NET technologies.
That being said, Java has a rich ecosystem of libraries and frameworks that can help you accomplish virtually anything. So dive in, experiment, and keep exploring the vast world of Java development!
Did you find this post helpful? Have you tried any of these alternatives to LINQ in Java? Let us know in the comments below and share your experience with the community! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
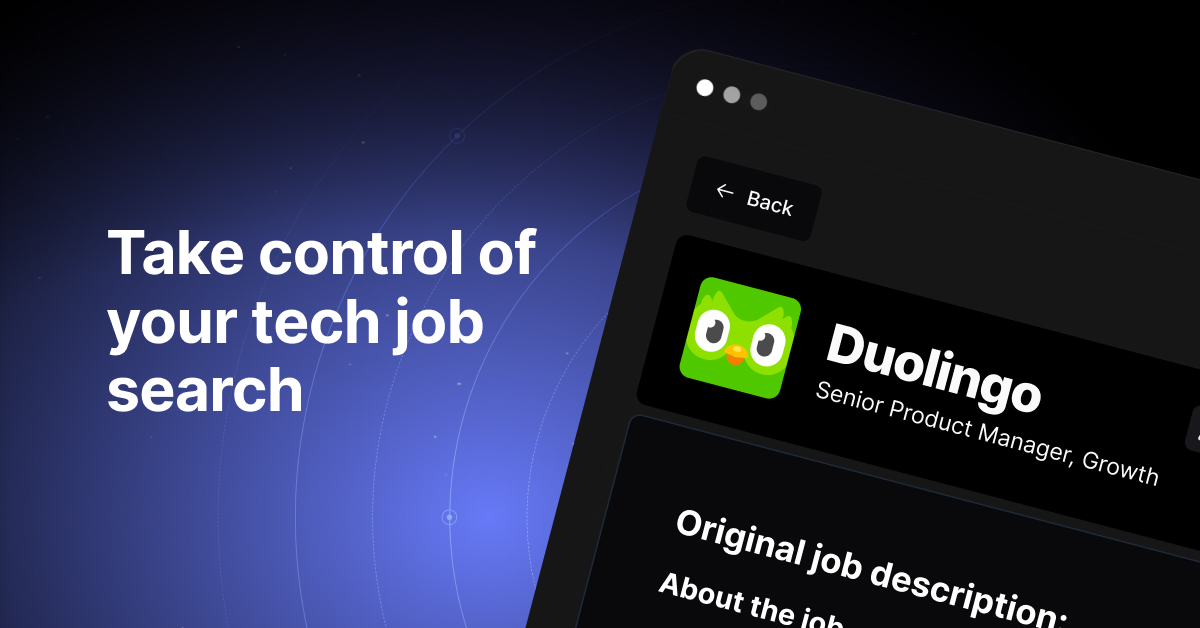