What is PECS (Producer Extends Consumer Super)?
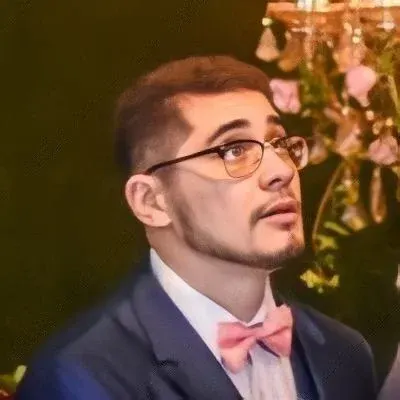
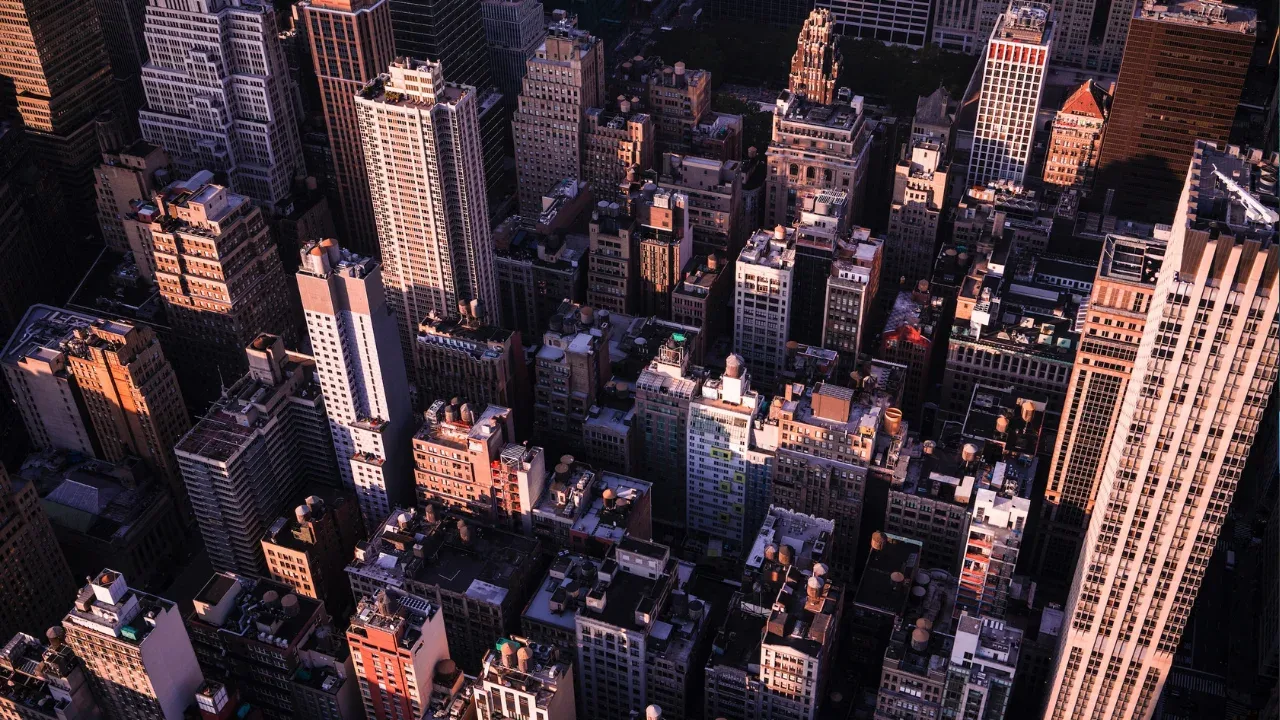
π The Ultimate Guide to PECS (Producer Extends Consumer Super) in Java Generics π
Are you scratching your head over the confusion between extends
and super
in Java generics? Don't worry, you're not alone! π€·ββοΈ
In this blog post, we'll demystify PECS (Producer Extends Consumer Super), a clever mnemonic that helps clear up the confusion and use extends
and super
in the right way. Let's dive in! π‘
Understanding the Problem π€
When working with generics in Java, you may encounter situations where you need to specify type bounds using the extends
and super
keywords. The issue arises when we're not sure which one to use in different scenarios, leading to confusion and runtime errors. π«
Introducing PECS π
PECS, short for "Producer Extends Consumer Super," is a simple acronym that serves as a guiding principle for using extends
and super
in generics. It helps us understand the role of the type parameter in different contexts. Let's break it down:
Producer π£: If your code produces objects or values of a generic type, use
? extends T
(theextends
keyword), whereT
is the upper bound for the type. It allows you to access the elements using methods defined in the upper bound type.Consumer π½οΈ: If your code consumes objects or values of a generic type, use
? super T
(thesuper
keyword), whereT
is the lower bound for the type. It allows you to pass different subtypes ofT
and work with them using methods defined in the lower bound type.
Resolving Confusion with Examples β
Producer (extends) π£
Let's say we have a method printList
that accepts a list and prints its contents. To make it more flexible, we want it to accept not just any list but a list of any subtype of Number
. Here's how PECS can help:
public void printList(List<? extends Number> list) {
for (Number num : list) {
System.out.println(num);
}
}
By using ? extends Number
, we can pass a List<Integer>
, List<Double>
, or any other subtype of Number
to printList
. This allows us to produce elements of a generic type in a safe way.
Consumer (super) π½οΈ
Now, imagine we have another method addElement
that accepts a list and adds an element to it. We want it to be capable of accepting not just any list but a list of any supertype of Integer
. Again, let's see how PECS comes to the rescue:
public void addElement(List<? super Integer> list, Integer element) {
list.add(element);
}
By using ? super Integer
, we can pass a List<Number>
, List<Object>
, or any super type of Integer
to addElement
. This allows us to consume elements of a generic type in a safe manner.
Time to Master PECS! β‘οΈ
Understanding PECS is crucial in writing flexible and reusable code with generics. By applying this simple mnemonic, you can eliminate confusion and avoid runtime errors caused by incorrect use of extends
and super
.
So, the next time you encounter generics in Java, remember PECS! Whether you're producing or consuming objects, PECS will be your trustworthy companion, guiding you to the right path. πΊοΈ
Feel free to share your thoughts and experiences in the comments below. Let's master PECS together! π
π£ Your Action Is Needed! π
Now that you're equipped with a clear understanding of PECS, it's time to put it into practice! Jump into your code editor and try implementing a few examples using extends
and super
. Share your newfound knowledge and ask any questions you may have.
Keep the discussion going and help fellow developers by sharing this blog post on your favorite social media platforms. Let's spread the word about PECS and make Java generics easier for everyone! πβ¨
Happy coding! π»π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
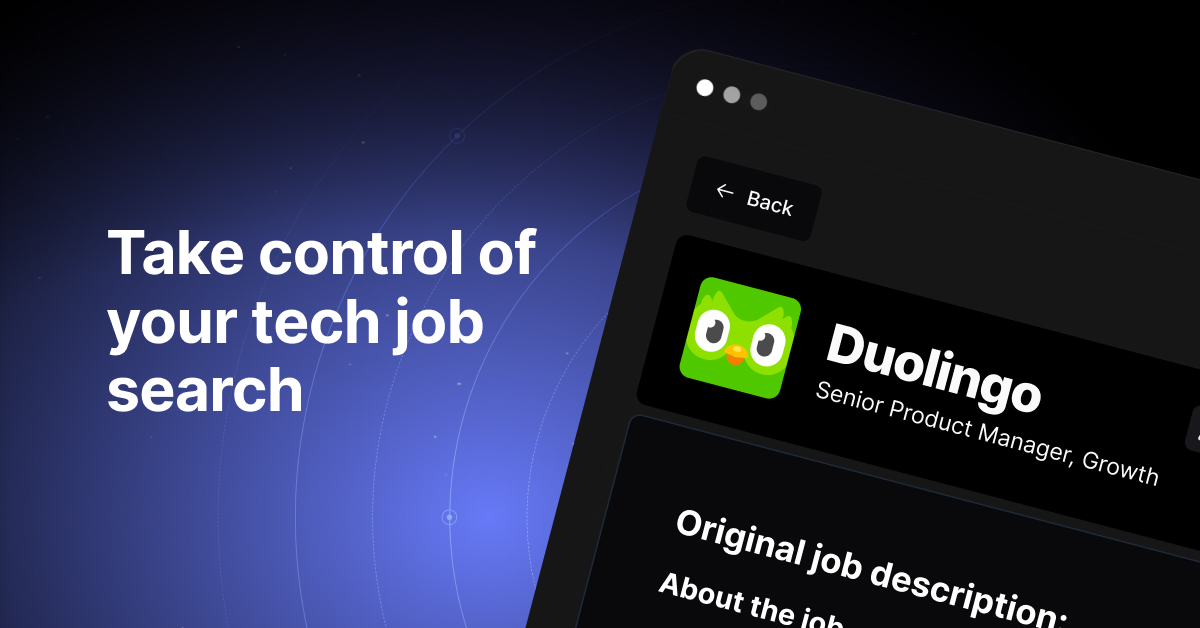