What is an efficient way to implement a singleton pattern in Java?
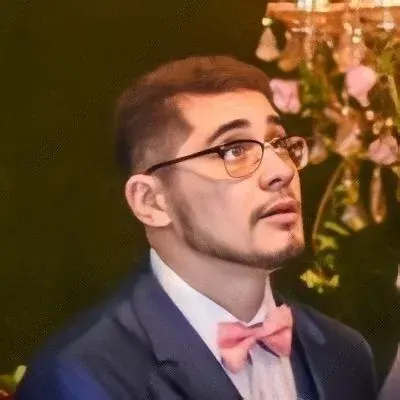
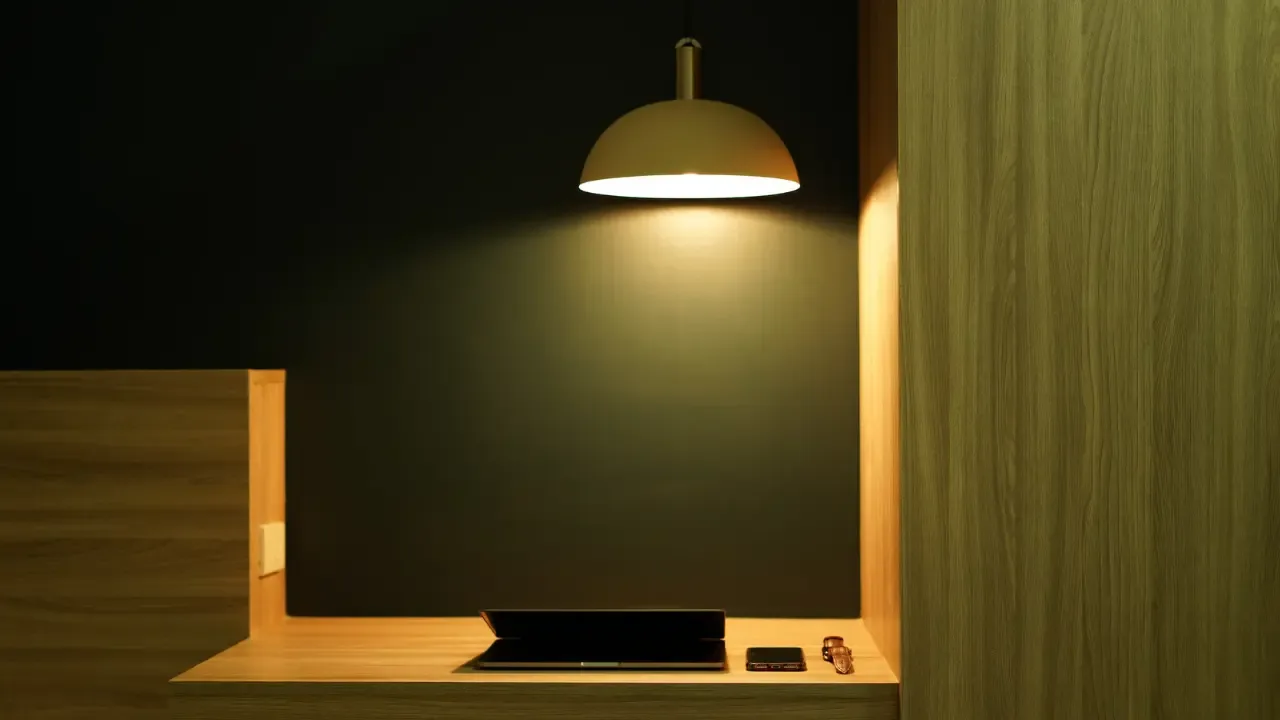
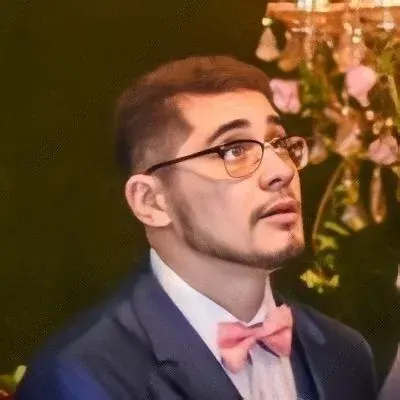
🌟 Calling all Java developers! 🌟
Have you ever wondered what's the most efficient way to implement a singleton design pattern in Java? 🤔 Look no further! In this blog post, we'll dive deep into the world of singletons and discover easy solutions to common issues. So grab your coffee ☕ and let's get started!
But first, let's quickly recap what a singleton is. Simply put, a singleton is a design pattern that ensures a class has only one instance, while providing a global point of access to it. It's super handy when you want to control access to a shared resource or limit the number of instances of a class.
Now let's address a common problem when implementing singletons - thread safety. Without proper synchronization, multiple threads might create multiple instances of the singleton class. 😱 To tackle this, we can use one of the following approaches:
Approach 1: Eager Initialization
One way to ensure thread safety is to eagerly create the singleton instance. This means that the instance is created when the class is loaded, even if it is not immediately being used. Here's an example:
public class MySingleton {
private static final MySingleton instance = new MySingleton();
private MySingleton() {
// private constructor to prevent instantiation
}
public static MySingleton getInstance() {
return instance;
}
}
With this approach, the singleton instance is created as soon as the class is loaded, ensuring thread safety. However, it has a downside - the instance is created even if it's not used, which might be wasteful in some cases.
Approach 2: Lazy Initialization
If eager initialization doesn't suit your requirements, you can opt for lazy initialization. This means that the singleton instance is created when it is first requested. Here's an example:
public class MySingleton {
private static MySingleton instance;
private MySingleton() {
// private constructor to prevent instantiation
}
public static synchronized MySingleton getInstance() {
if (instance == null) {
instance = new MySingleton();
}
return instance;
}
}
In this approach, the getInstance
method checks if the instance is null, and if so, creates it. Although this approach is thread-safe, it can introduce performance bottlenecks due to the synchronized keyword.
Approach 3: Double-checked Locking
To overcome the performance issue in the lazy initialization approach, we can use double-checked locking. This approach ensures that locking only occurs when the instance is null. Here's an example:
public class MySingleton {
private static volatile MySingleton instance;
private MySingleton() {
// private constructor to prevent instantiation
}
public static MySingleton getInstance() {
if (instance == null) {
synchronized (MySingleton.class) {
if (instance == null) {
instance = new MySingleton();
}
}
}
return instance;
}
}
By using the volatile
keyword and double-checked locking, we achieve both lazy initialization and thread safety without incurring the synchronized overhead in every call to getInstance
.
Now that you know some efficient ways to implement a singleton pattern in Java, it's time to put your knowledge into practice! 💪 Remember, the key to successful coding is to understand the problem at hand, choose the right solution, and never stop learning.
We hope you found this blog post helpful! If you have any questions or other tips you'd like to share, feel free to leave a comment below. Happy coding! ✨