What is a raw type and why shouldn"t we use it?
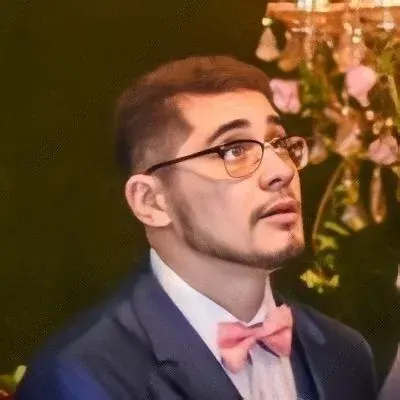
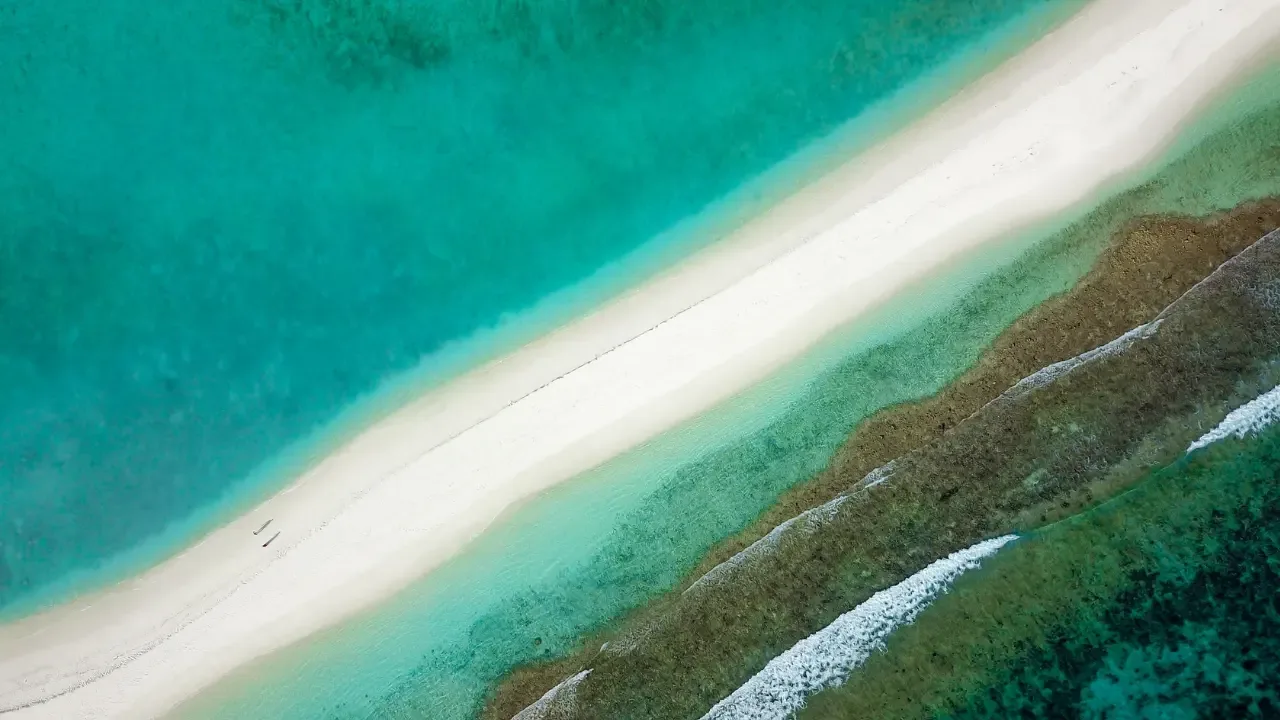
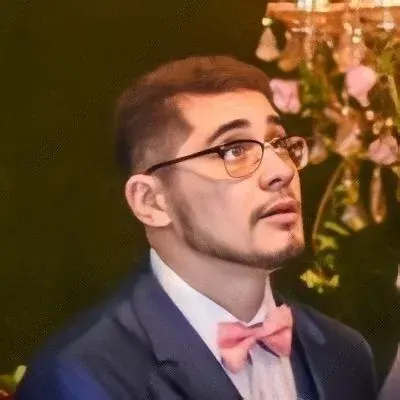
Raw Types: Unveiling the Mystery 🎭
You're about to embark on a journey that will unravel the mystery surrounding raw types in Java 🚀. You may have heard whispers in the tech realm that raw types shouldn't be used in new code, and we're here to explain why! We'll also explore the perfect alternative that will elevate your code to new heights of sophistication ✨.
What Are Raw Types? 🤔
In Java, a raw type is a reference to a generic class or interface that omits type arguments. Essentially, it's a generic class where you've omitted the type parameter(s) in the angle brackets. This allows you to interact with generics in a non-generic way 😮.
Here's an example to illustrate the concept. Let's say we have a generic class called Box<T>
:
public class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
Now, let's see how it looks when we use a raw type:
Box rawBox = new Box();
By omitting the type parameter, we create a raw type. This means we lose type-safety 😱 because we can insert objects of any type into the Box
, defeating the purpose of generics.
Why Are Raw Types a No-Go? 🙅♂️
Using raw types can lead to several perils in your codebase:
💥 Type Safety Issues: Without type information, the compiler cannot enforce type checks, throwing type-safety out the window. This leaves your code vulnerable to runtime errors.
💡 Lost Benefits of Generics: Generics provide compile-time type checking and eliminate the need for explicit casting. With raw types, you lose all these benefits, resulting in less-readable, error-prone code.
🐛 Hidden Bugs and Maintenance Nightmares: Raw types can hide bugs and make your code a breeding ground for elusive errors. Tracking down and fixing these issues can consume valuable time and resources.
The Alternative: Embrace Wildcards! 🌟
Now that we understand the perils of raw types, let's introduce you to the fantastic alternative: wildcards! 🎉 Wildcards allow us to write generic code while still providing flexibility.
There are two different wildcard types: ? extends T
and ? super T
. The ? extends T
wildcard represents an unknown subtype of T
, while the ? super T
wildcard represents an unknown supertype of T
.
Let's refactor our previous Box
example to showcase the power of wildcards:
public class Box<T> {
private List<? extends T> items;
public void setItems(List<? extends T> items) {
this.items = items;
}
public List<? extends T> getItems() {
return items;
}
}
By using <? extends T>
, we can safely handle different subtypes of T
, while maintaining type safety and flexibility. This way, we ensure that we never violate the type contract.
Your Call to Action: Level Up Your Code 💪
Now that you've gained a deeper understanding of raw types and their risks, it's time to put your newfound knowledge into action! Here's what you can do:
Refactor Raw Types: Identify any instances of raw types in your codebase and refactor them using wildcard types. Embrace the power of generics to enhance type safety and maintainability.
Spread the Word: Share this blog post with your fellow developers! Let them know about the dangers of raw types and the benefits of using wildcards. Together, we can build a codebase that is cleaner and more robust.
Remember, embracing wildcards opens up a world of possibilities while keeping your codebase secure. So, what are you waiting for? Let's level up our code! 💻✨
(In case you have any questions or further insights to share, feel free to leave a comment below!)