What is a daemon thread in Java?
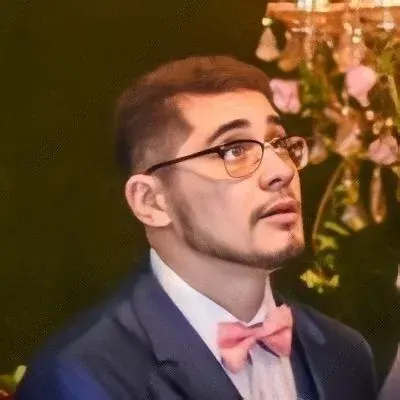
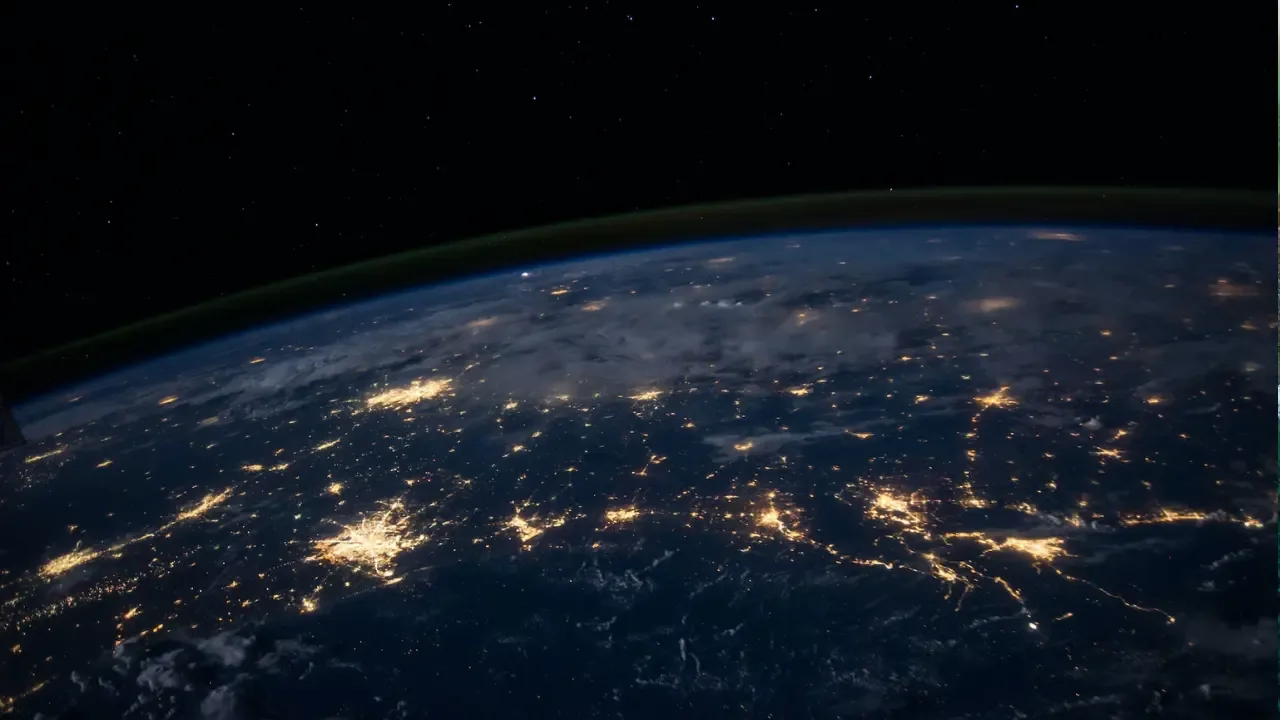
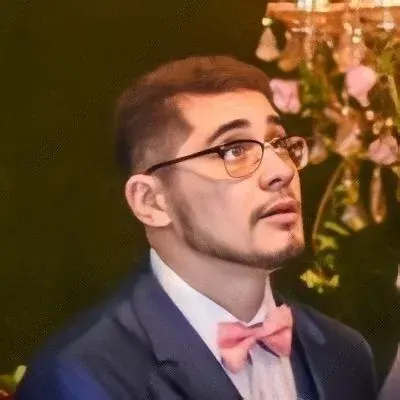
What is a Daemon Thread in Java? ๐งตโจ
Have you ever wondered what those mysterious "daemon threads" in Java are? ๐ค Well, you've come to the right place! In this blog post, we will demystify daemon threads and explain what they are, how they work, and when you might encounter them in your Java programming adventures. ๐
Understanding Daemon Threads ๐ฐ๏ธ
In Java, a daemon thread is a special type of thread that runs in the background and doesn't prevent the JVM (Java Virtual Machine) from terminating. Unlike regular threads, daemon threads don't keep the application alive if all other non-daemon threads have finished executing. ๐งต๐ค
Daemon threads are typically used for tasks that don't require explicit termination and can safely be abandoned when the main application thread finishes. Some common examples of daemon threads include garbage collection, background logging, and various maintenance tasks. ๐๏ธ๐๐ง
Identifying Daemon Threads in Java ๐
You might be wondering how to determine whether a thread is a daemon thread or not. ๐ก Luckily, Java provides a simple method to check whether a given thread is a daemon thread or a regular (non-daemon) thread.
To check if a thread is a daemon thread, you can use the isDaemon()
method. This method returns true
if the thread is a daemon thread, and false
otherwise. Here's a quick example:
Thread myThread = new Thread(() -> {
// Thread logic goes here
});
if (myThread.isDaemon()) {
System.out.println("This is a daemon thread!");
} else {
System.out.println("This is a regular thread.");
}
Creating Daemon Threads in Java ๐งตโ๏ธ
Creating a daemon thread in Java is straightforward. Before starting a thread, you can simply call the setDaemon(true)
method on it to mark it as a daemon thread. Here's an example:
Thread myThread = new Thread(() -> {
// Thread logic goes here
});
myThread.setDaemon(true); // Marking it as a daemon thread
myThread.start(); // Starting the thread
Remember that you must mark a thread as a daemon thread before starting it; otherwise, you'll get an IllegalThreadStateException
.
A Caveat to Keep in Mind โ ๏ธ
One important thing to note is that if all non-daemon threads finish their execution, any remaining daemon threads are abruptly terminated, often without having a chance to clean up or complete their tasks. So, be cautious when using daemon threads and ensure that they can handle unexpected termination gracefully. ๐ฎ๐ฅ
Call-to-Action: Embrace the Power of Daemon Threads! ๐ช๐ซ
Now that you know what daemon threads are and how they work in Java, you can leverage their power to handle background tasks and streamline your applications. ๐ So, go ahead and experiment with daemon threads in your next Java project, and remember to use them wisely.
If you have any stories or insights about using daemon threads, we'd love to hear from you in the comments! Let's spark a discussion and share our experiences with this fascinating aspect of Java threading. ๐๐ฃ๏ธ
Keep coding and threading! ๐ฉโ๐ป๐งต๐จโ๐ป