View"s getWidth() and getHeight() returns 0
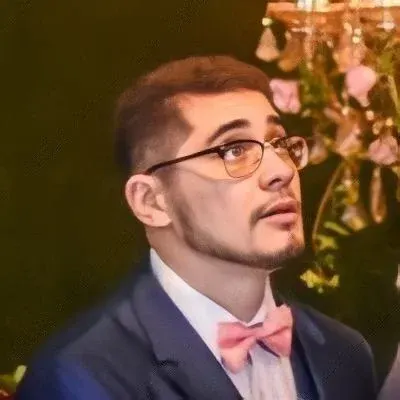
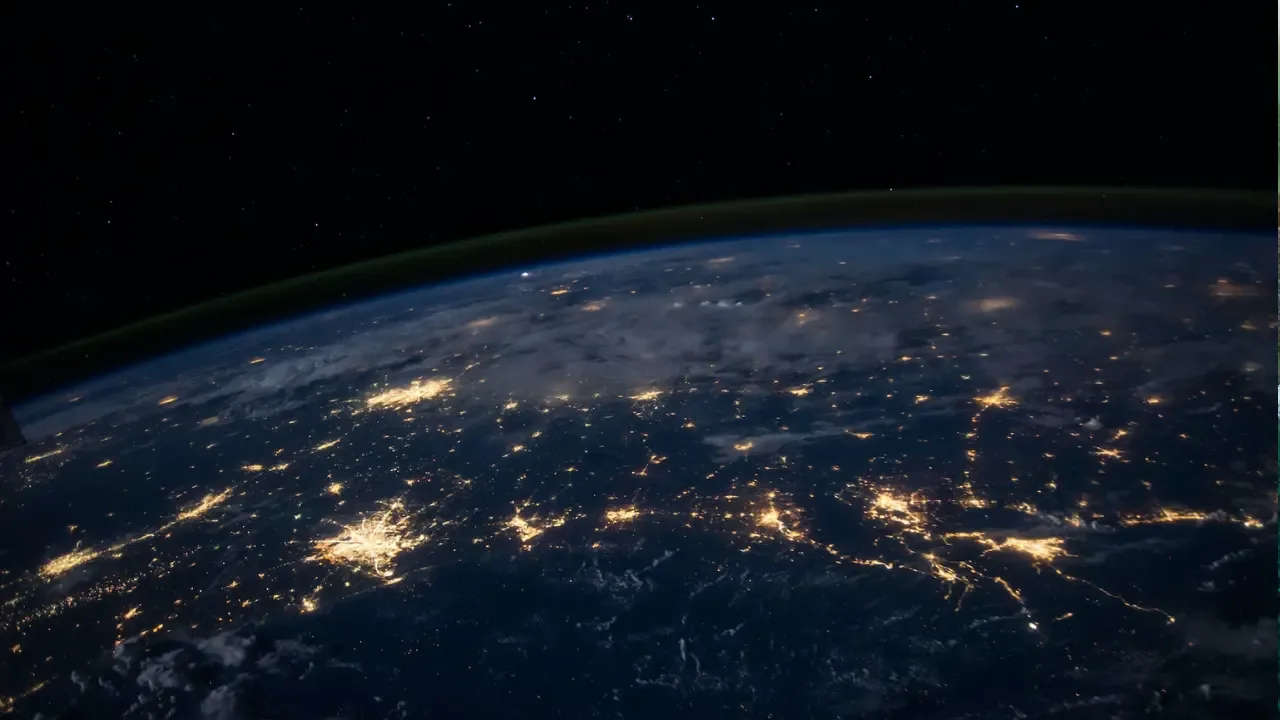
📝 Guide: How to Solve the Issue with View's getWidth() and getHeight() Returning 0
So, you're developing an Android project and you've encountered a problem. The getWidth()
and getHeight()
methods of a View
are returning 0 when you're trying to obtain the width and height of a button dynamically. Don't worry, we've got you covered! In this guide, we'll address this common issue and provide you with easy solutions to fix it.
🔍 Understanding the Problem
The issue you're facing is quite common when working with dynamically created elements in Android. In your case, you're trying to rotate a button by obtaining its width and height, but these methods are returning 0. This happens because the getWidth()
and getHeight()
methods are called too early in the activity lifecycle.
💡 Solution 1: Using ViewTreeObserver
One way to solve this problem is by using the ViewTreeObserver
to get the dimensions of the button after it has been laid out on the screen. Here's an example of how you can implement this:
// Add this code inside the onCreate() method, after creating the button
bt.getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
// Remove the listener to avoid multiple calls
bt.getViewTreeObserver().removeOnGlobalLayoutListener(this);
// Now you can obtain the width and height of the button
int width = bt.getWidth();
int height = bt.getHeight();
// Continue with your button rotation logic
RotateAnimation ra = new RotateAnimation(0, 360, width / 2, height / 2);
ra.setDuration(3000L);
ra.setRepeatMode(Animation.RESTART);
ra.setRepeatCount(Animation.INFINITE);
ra.setInterpolator(new LinearInterpolator());
bt.startAnimation(ra);
}
});
By adding an OnGlobalLayoutListener
to the button's ViewTreeObserver
, we ensure that the code inside the onGlobalLayout()
method is executed when the button's dimensions are available.
💡 Solution 2: Using post() Method
Another approach to solving this issue is by using the post()
method of the button's View
object. This method allows you to run a piece of code after the view has been laid out. Here's how you can implement it:
// Add this code inside the onCreate() method, after creating the button
bt.post(new Runnable() {
@Override
public void run() {
// Now you can obtain the width and height of the button
int width = bt.getWidth();
int height = bt.getHeight();
// Continue with your button rotation logic
RotateAnimation ra = new RotateAnimation(0, 360, width / 2, height / 2);
ra.setDuration(3000L);
ra.setRepeatMode(Animation.RESTART);
ra.setRepeatCount(Animation.INFINITE);
ra.setInterpolator(new LinearInterpolator());
bt.startAnimation(ra);
}
});
Using the post()
method is another way to ensure that the code inside the run()
method is executed after the button's dimensions are available.
⚡️ Take Action and Engage
Now that you have learned two easy solutions to fix the issue with getWidth()
and getHeight()
returning 0, it's time to put your knowledge into practice! Choose the solution that works best for your specific case and integrate it into your code.
Feel free to experiment and share your experience in the comments below. If you have any questions or need further assistance, our community is always here to help!
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
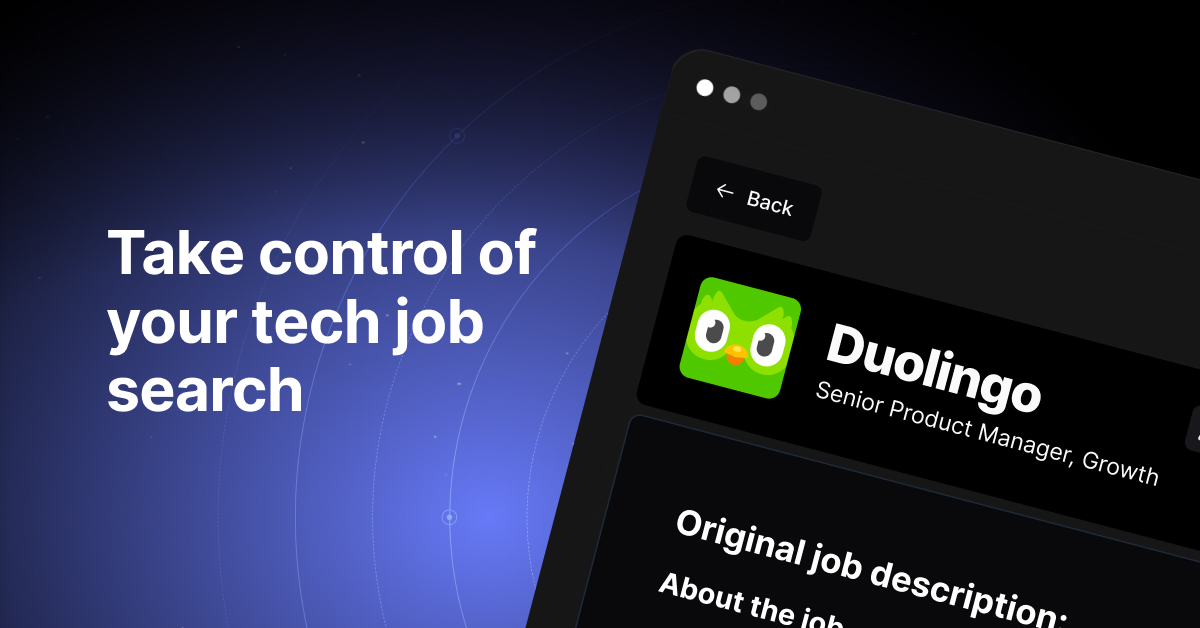