Use String.split() with multiple delimiters
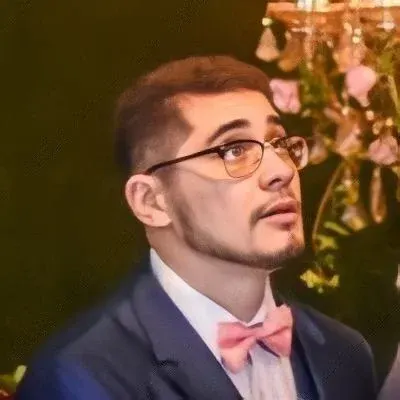
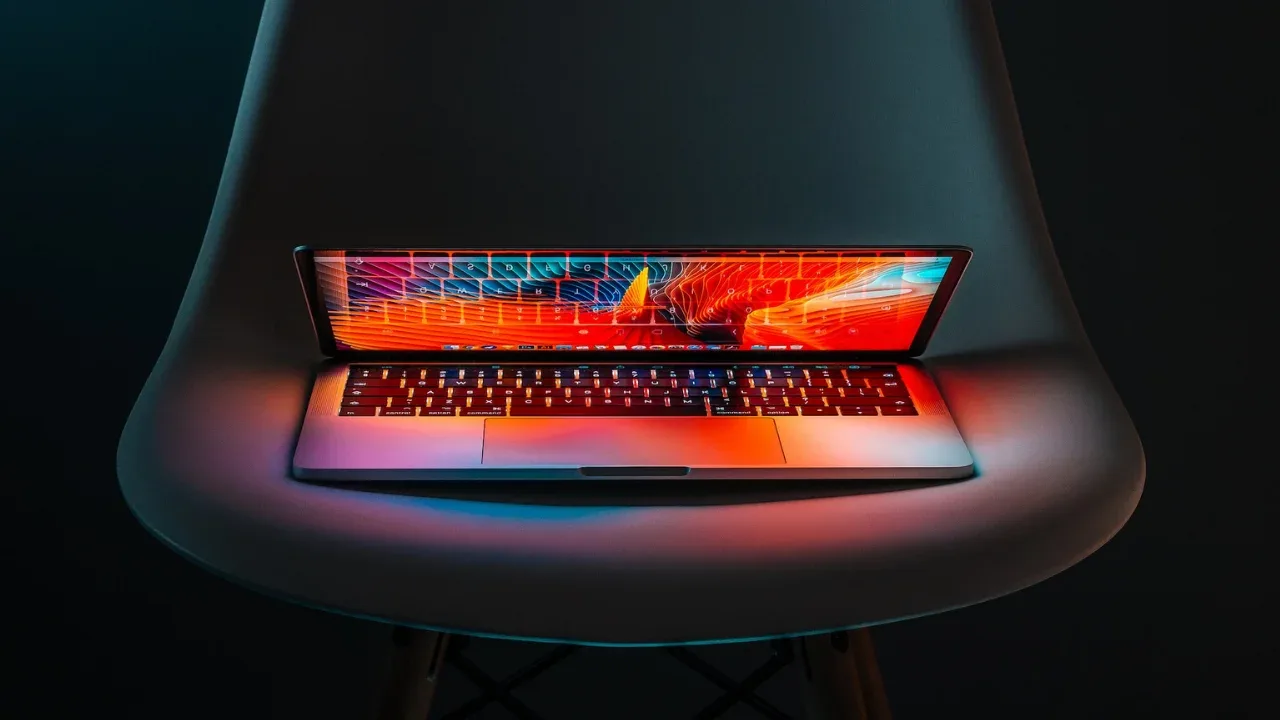
Splitting a String with Multiple Delimiters: A Comprehensive Guide ๐
Are you struggling to split a string based on multiple delimiters? Don't worry, you're not alone! This common issue can be tricky to tackle, but fear not, we're here to help you master this useful technique. In this guide, we'll walk you through the steps to split a string using multiple delimiters using the String.split()
method in Java. Let's dive in! ๐ช
The Problem: Splitting a String with Multiple Delimiters
So, you need to split a string based on the delimiters "-" and ".". Let's take a look at the desired output:
Input:
AA.BB-CC-DD.zip
Desired Output:
AA
BB
CC
DD
zip
The Failed Attempt: A Closer Look at the Code
As mentioned in the code snippet you provided, you attempted to split the string using the String.split()
method. However, your approach did not yield the desired result. Let's analyze what went wrong:
private void getId(String pdfName){
String[] tokens = pdfName.split("-\\.");
}
The issue here lies in your regular expression. You used -\\.
as the delimiter, but this is incorrect. In regular expressions, the dot (.
) has a special meaning and matches any character. To split based on a literal dot, you need to escape it using the backslash (\
). However, in this case, you want to split on either a dash (-
) or a dot (.
). So, we need to approach this problem differently.
The Solution: Using Regular Expressions with Character Classes
To split a string based on multiple delimiters in Java, we need to leverage the power of regular expressions and character classes. Character classes allow us to define a set of characters that should be considered as delimiters. In our case, the character class should include both the dash and the dot.
Here's the corrected code that will give us the desired output:
private void getId(String pdfName){
String[] tokens = pdfName.split("[-.]");
}
By using [-.]
as the regular expression parameter, we're instructing the split()
method to use either a dash or a dot as a delimiter. Java will split the string wherever it encounters either of these characters.
Putting It All Together
Let's run the corrected code snippet on the example input string and see the magic happen:
private void getId(String pdfName){
String[] tokens = pdfName.split("[-.]");
for(String token : tokens){
System.out.println(token);
}
}
Output:
AA
BB
CC
DD
zip
Voila! We've successfully split the string based on the delimiters "-" and ".".
Keep Exploring and Experimenting ๐งช
Now that you've learned how to split a string using multiple delimiters in Java, the possibilities are endless! You can try using different input strings, add more delimiters, or even combine different regular expression patterns to suit your needs. The key is to keep exploring and experimenting to become a regex ninja! ๐
Engage with Us!
Did this guide help you solve your problem? Do you have any other questions or topics you'd like us to cover? Let us know in the comments below! We love hearing from our readers and providing tailored solutions to their coding conundrums. ๐
Happy coding! ๐ป๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
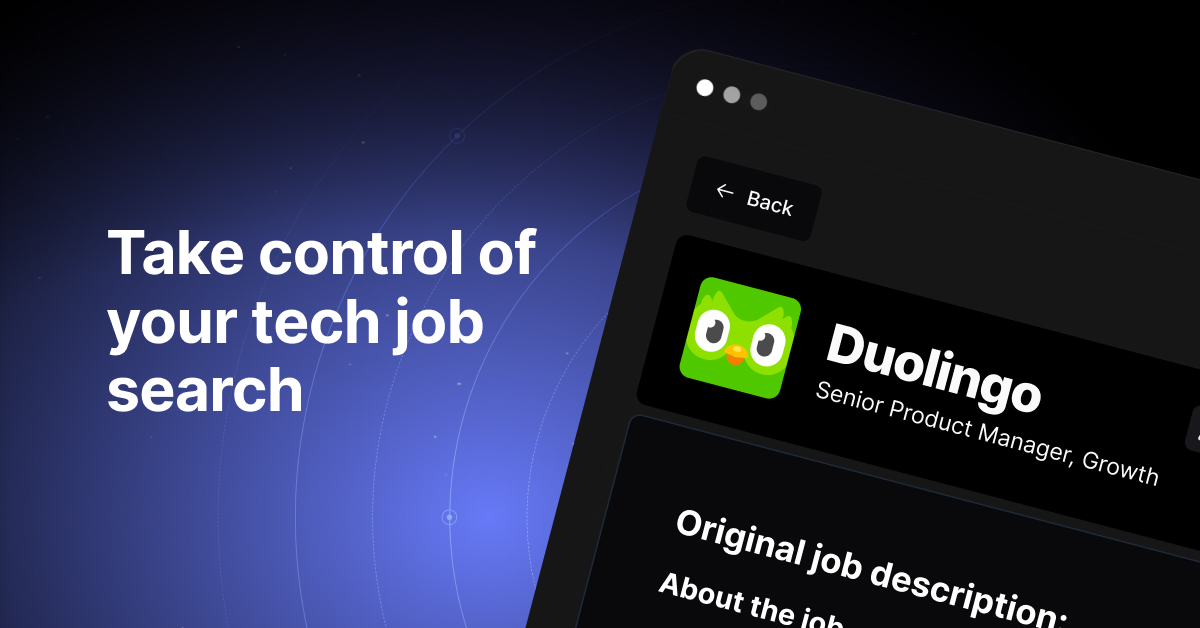