Use JAXB to create Object from XML String
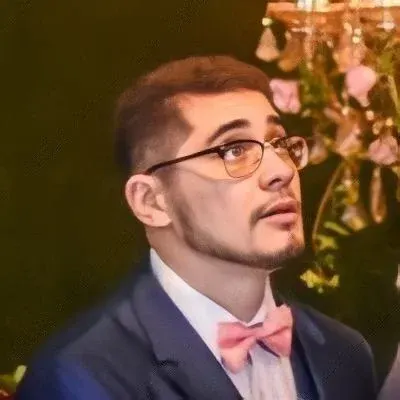
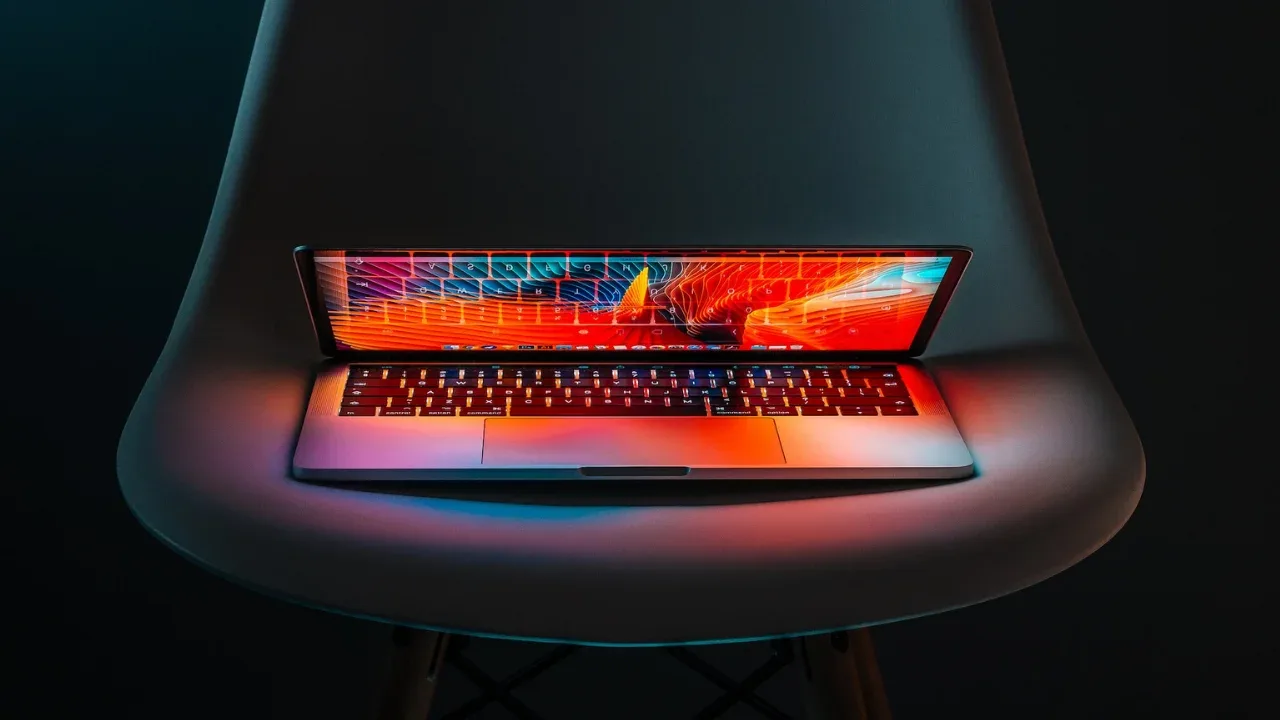
📢 Hey tech enthusiasts! Today, we're going to explore the exciting world of JAXB 🌐 and learn how to create an object from an XML string 📜. Sounds awesome, right? Let's dive right in! 💦💥
So, the scenario is pretty straightforward. We've got a code snippet that uses JAXB to unmarshal an XML string and map it to a JAXB object 📦. Here's the code in question:
JAXBContext jaxbContext = JAXBContext.newInstance(Person.class);
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
Person person = (Person) unmarshaller.unmarshal("xml string here");
Moreover, we have a Person
class 🧍♂️ with a few fields annotated with @XmlElement
. Let's take a look at it:
@XmlRootElement(name = "Person")
public class Person {
@XmlElement(name = "First-Name")
String firstName;
@XmlElement(name = "Last-Name")
String lastName;
// Getters and setters omitted for brevity
}
Now that we've set the stage, let's address some common issues and provide easy solutions to unmarshal that XML string into a Person
object 🚀.
Common Issue: Unmarshalling XML String
🔍 The issue at hand is how to correctly unmarshal the XML string into the Person
object using JAXB.
🔧 Solution: To unmarshal the XML string, we need to follow these steps:
Create a new
JAXBContext
instance by passing thePerson
class as a parameter. This creates a context for the JAXB API to work with ourPerson
object.
JAXBContext jaxbContext = JAXBContext.newInstance(Person.class);
Create an
Unmarshaller
from theJAXBContext
that we just created. TheUnmarshaller
is responsible for converting XML data into Java objects.
Unmarshaller unmarshaller = jaxbContext.createUnmarshaller();
Finally, use the
unmarshal()
method of theUnmarshaller
to convert the XML string into aPerson
object.
Person person = (Person) unmarshaller.unmarshal("xml string here");
And just like that, we've successfully unmarshalled the XML string into a Person
object 🙌.
Now it's your turn to give it a try! ✨ Play around with the code, test it out, and see the magic of JAXB in action.
If you found this post helpful or have any questions or cool insights to share, let us know in the comments below ⬇️. We'd love to hear from you! And don't forget to share this post with your tech-savvy friends who might find this information useful. Until next time, happy coding! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
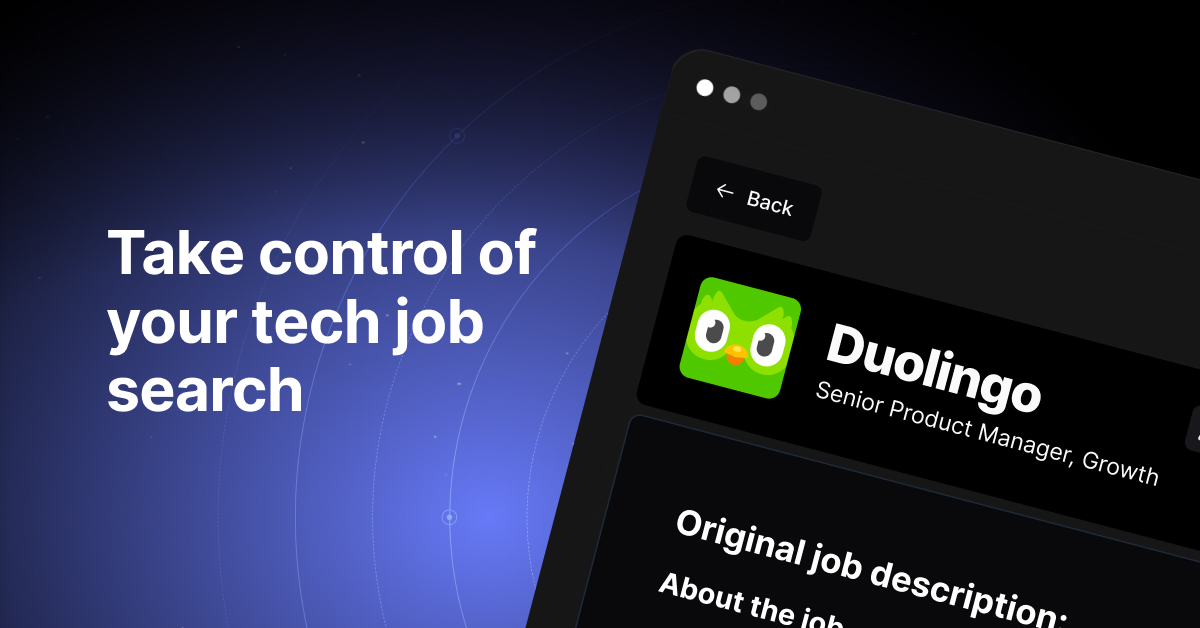