Unit testing with Spring Security
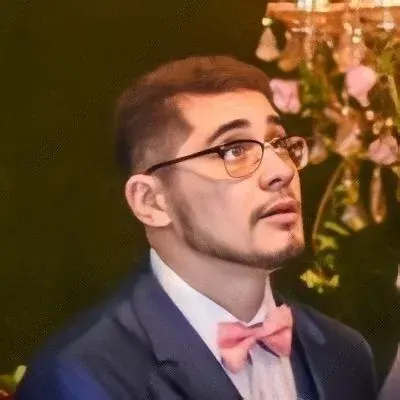
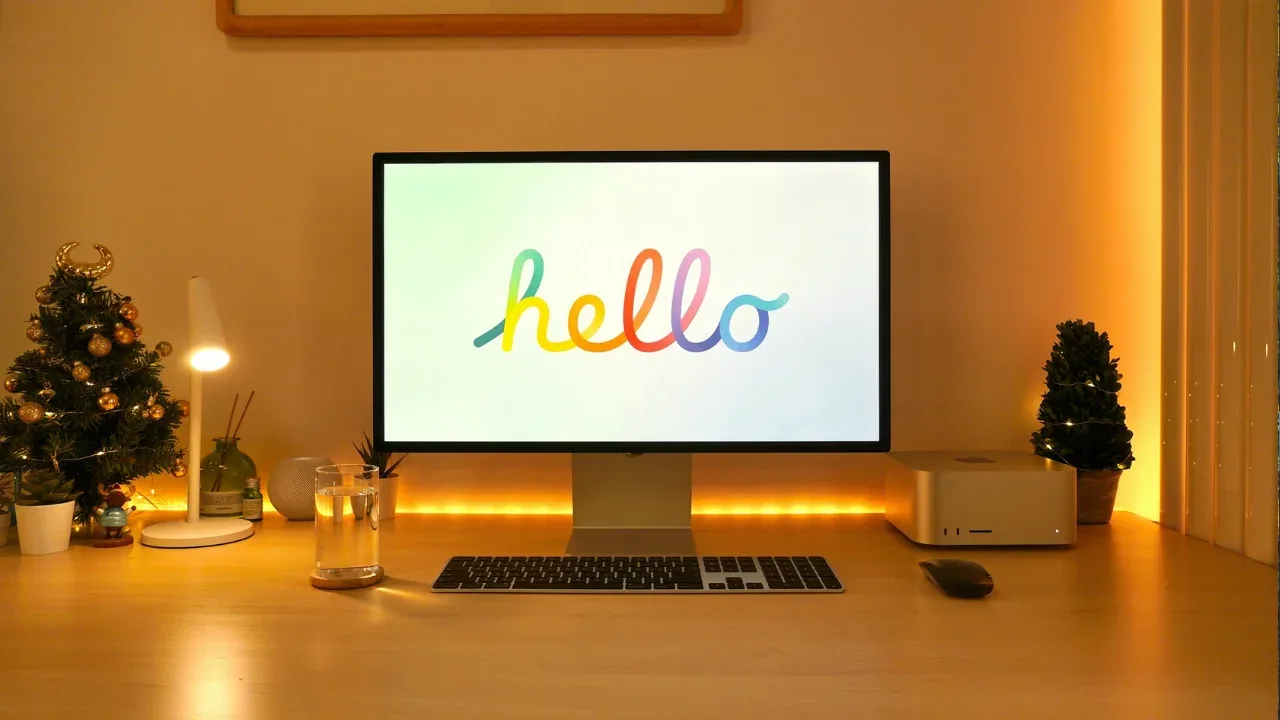
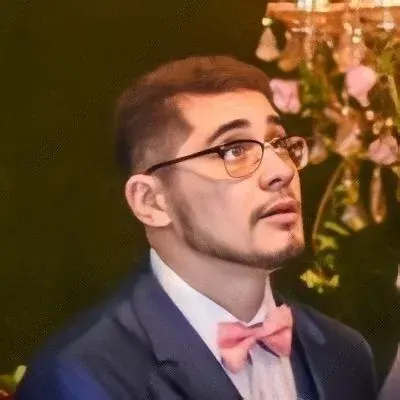
Unit Testing with Spring Security: Simplifying Authentication for Your Tests š”ļø
š Welcome to our blog! Today, we'll address a common issue that many developers face when unit testing with Spring Security. We'll provide you with easy solutions to simplify authentication in your tests and make your life a whole lot easier. So, let's dive in! šŖ
Understanding the Challenge š
In our quest to determine if Spring Security is the right fit for our project, we stumbled upon an issue with authentication in our unit tests. Our goal is to replace our home-grown logic with Spring Security, but we need a way to inject an Authentication
object into the SecurityContext
during testing.
The Traditional Approach š¶āāļø
One way to tackle this challenge is through the SecurityContextHolder
. This Spring Security object provides a thread-local SecurityContext
to access information about the authenticated user. However, this approach feels a bit un-Spring-like, as the SecurityContextHolder
is a global singleton.
To authenticate a user in our unit tests, we would typically wire it up in the initialization method of each test case, like this:
protected void setUp() throws Exception {
...
SecurityContextHolder.getContext().setAuthentication(
new UsernamePasswordAuthenticationToken(testUser.getLogin(), testUser.getPassword()));
...
}
While this solution works, it can become verbose and repetitive, increasing the likelihood of errors creeping into our codebase. š
A Simpler Solution: Using Spring Security Test Mocks š
Luckily, Spring Security provides a way to simplify the authentication process in our unit tests: the @WithMockUser
annotation. This annotation allows us to mock an authenticated user, making our tests more concise and readable.
To use @WithMockUser
, we can annotate our test methods or entire test classes with it. Let's see how it works in action:
@SpringBootTest
class YourUnitTest {
@Test
@WithMockUser(username = "john", roles = { "USER" })
void testYourMethod() {
// Your test logic here
}
}
By providing the username
and roles
attributes in the annotation, we can easily simulate an authenticated user during our unit tests. This way, we can focus on testing the specific functionalities of our application without worrying about authentication boilerplate code. š
Level Up Your Testing Game: Parameterized Authentication š®
What if you need to test various scenarios or different roles for your authenticated users? Fear not! Spring Security's test mocks have got you covered.
Let's say we have two roles, "USER" and "ADMIN," and we want to test a method that requires different roles. We can achieve this by leveraging the Roles
attribute of @WithMockUser
. Look at this example:
@SpringBootTest
class YourUnitTest {
@ParameterizedTest
@WithMockUser(username = "john", roles = { "USER" })
@WithMockUser(username = "jane", roles = { "ADMIN" })
void testYourMethod(String username) {
// Your test logic here
}
}
Using the @ParameterizedTest
from JUnit, we can now pass multiple usernames to our test method, each with their respective roles. This enables us to cover a wider range of scenarios and ensure our authentication and authorization mechanisms are working as expected. š§©
Conclusion and Your Call to Action š
Congratulations! We've explored a simpler way to handle authentication during unit tests with Spring Security. By using Spring Security test mocks, such as @WithMockUser
, we can ensure our tests are concise, readable, and reliable.
Now it's your turn! Give these techniques a try in your own testing environment. Share your experiences with us in the comments below and let us know if you have any other questions or challenges you'd like us to address.
Happy testing! š