Understanding checked vs unchecked exceptions in Java
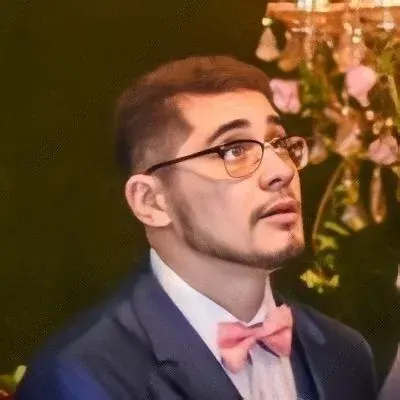
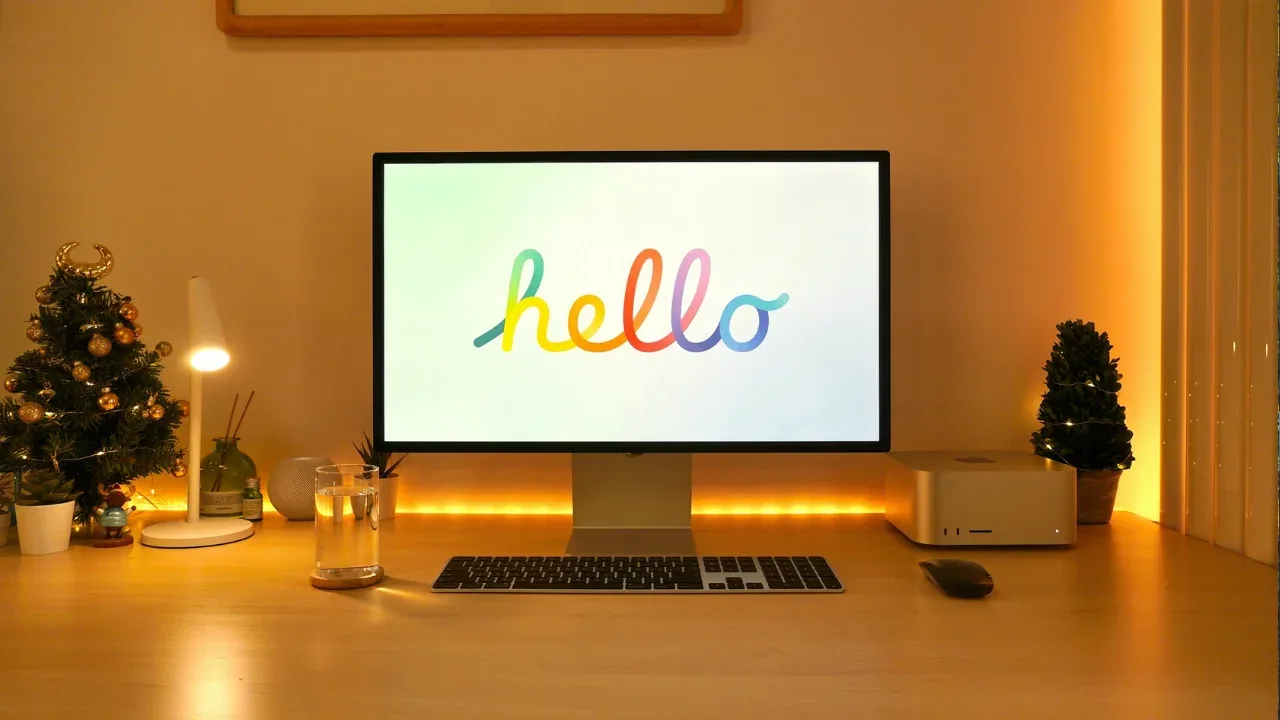
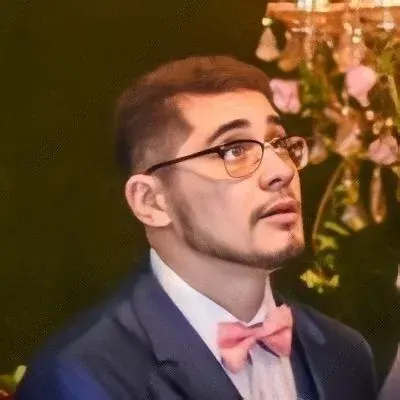
📝 Understanding Checked vs Unchecked Exceptions in Java 🕵️
<p>Hey there! Have you ever come across these terms - checked exceptions and unchecked exceptions in Java? 🤔 Trust me, I understand how confusing it can be. But worry not, I'm here to break it down for you in the simplest way possible! Let's dive right in! 🏊♀️</p>
✅ Checked Exceptions
<p>A checked exception is a type of exception that **must be declared** in a method's signature or caught within the method's body. These exceptions are mainly used for **recoverable conditions** and indicate situations where a method can potentially encounter an expected problem and needs to be handled explicitly.</p>
<p>Let's take an example. Consider the following code snippet:</p>
try {
String userInput = //read in user input
Long id = Long.parseLong(userInput);
} catch (NumberFormatException e) {
id = 0; //recover the situation by setting the id to 0
}
<p>So, is the code above considered a checked exception? 🤔 Yes, absolutely! In this case, we are trying to convert a string to a `Long`. If the string cannot be parsed as a number, a `NumberFormatException` is thrown. To handle this exception, we catch it and recover the situation by setting the `id` to 0. 🎉</p>
❌ Unchecked Exceptions
<p>An unchecked exception, on the other hand, is a type of exception that **is not required to be declared** in a method's signature or caught within the method's body. These exceptions typically represent **programming errors** rather than recoverable conditions, hence they can be left unhandled.</p>
<p>Let's take a look at another example:</p>
try {
File file = new File("my/file/path");
FileInputStream fis = new FileInputStream(file);
} catch (FileNotFoundException e) {
// What should I do here? 🤷♀️
// Should I throw a new FileNotFoundException("File not found")?
// Should I log the exception?
// Or should I exit the program using System.exit(0)?
}
<p>In this case, we are trying to open a file using `FileInputStream`. If the file is not found, a `FileNotFoundException` is thrown. Now, here comes the question - what should we do in the catch block? 🤔 Well, it depends on the specific situation. You could throw a new exception, log the exception for debugging purposes, or even exit the program if it's a critical error. The choice is yours! 😄</p>
<p>But wait, couldn't the code above also be a checked exception? Can we recover the situation? Let's see:</p>
try {
String filePath = //read in from user input file path
File file = new File(filePath);
FileInputStream fis = new FileInputStream(file);
} catch (FileNotFoundException e) {
// Kindly prompt the user with an error message
// Somehow ask the user to re-enter the file path.
}
<p>Yes, absolutely! In this case, we are handling the `FileNotFoundException` by prompting the user with an error message and asking them to re-enter the file path. It's all about handling the exception and providing a fallback mechanism to recover from the error. 🛠️</p>
🤔 Why the Exception Bubbling?
<p>Have you ever encountered a situation where a method simply declares that it throws `Exception`? Ever wondered why some people let the exception bubble up instead of handling it sooner? Let's explore this a bit! ⏩</p>
<p>There could be several reasons for letting an exception bubble up instead of handling it immediately. Sometimes, it might be more appropriate for the calling method or the higher-level components to handle the exception based on the specific context. Additionally, it allows for better separation of concerns, maintainability, and code readability. Remember, handling the error sooner is not always better! 🚫✋</p>
<p>Furthermore, should you bubble up the exact exception or mask it using `Exception`? That depends on the situation as well. If the specific exception provides meaningful information about the error, it's better to bubble up the exact exception. However, if you feel that the specific exception might be too low-level or irrelevant for the higher-level components, you can choose to catch it and throw a more generalized `Exception`. It's all about finding the right balance and making your code more understandable! ✨💡</p>
📖 Further Reading
<p>Still curious to learn more about checked and unchecked exceptions? Check out these resources:</p>
<p>Feel free to explore these links and enhance your understanding of this fascinating topic! 📚🔍</p>
💥 Conclusion and Call-to-Action
<p>There you have it! We've covered the basics of checked and unchecked exceptions in Java. You now have a solid foundation to handle recoverable conditions and programming errors effectively. So, go ahead, dive into your code, and conquer those exceptions like a pro! 💪 If you found this article helpful, don't forget to share it with your fellow Java enthusiasts. Let's spread the knowledge!</p>
<p>Got any more questions or insights? 🤔 I'd love to hear from you! Leave a comment below and let's spark a discussion. Happy coding! 💻🔥</p>