Type safety: Unchecked cast
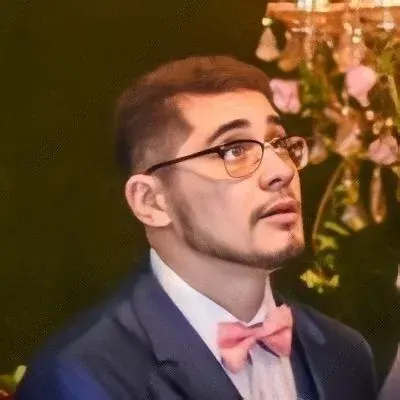
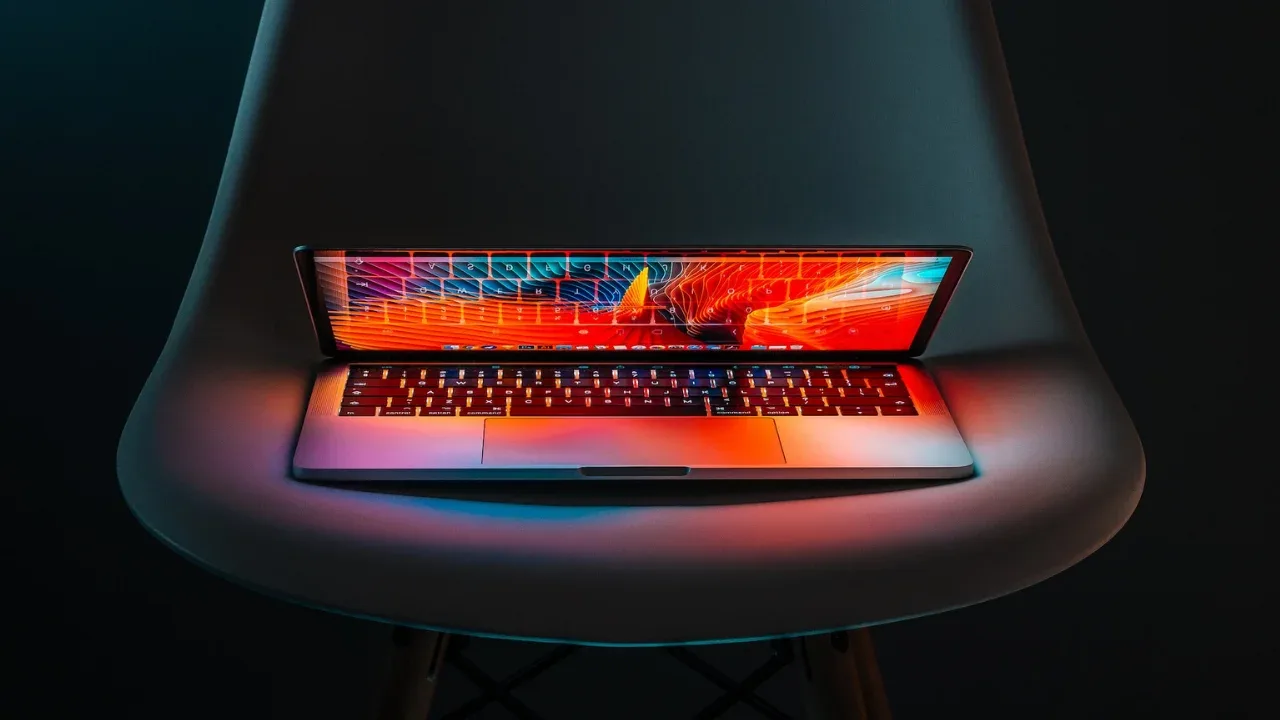
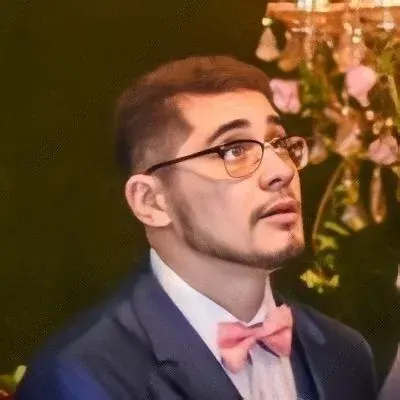
Type safety: Unchecked cast - What's the Deal? 😮
So you're working on your Java project, feeling all confident and mighty, when suddenly you come across this pesky warning in Eclipse:
Type safety: Unchecked cast from Object to HashMap<String,String>
And you're like, "What the heck does this even mean? And more importantly, what went wrong?"
Well, fear not! In this blog post, we're going to dive deep into this issue, understand what's happening, and explore some easy solutions to make that annoying warning vanish into thin air. Let's get started! 🙌
Understanding the Problem 👀
To understand what's going on, let's dissect the code that triggered the warning:
private Map<String, String> someMap = new HashMap<String, String>();
someMap = (HashMap<String, String>)getApplicationContext().getBean("someMap");
In simple terms, you're trying to assign a bean obtained from the application context to your someMap
object. The problem arises with the line (HashMap<String, String>)getApplicationContext().getBean("someMap")
.
The warning is telling you that you're performing an unchecked cast. An unchecked cast occurs when you're casting an object to a different type without any type-checking at compile-time. In this case, you're casting the Object
returned by getBean("someMap")
to a HashMap<String, String>
.
Now, why is this a problem? Well, it's because the getBean()
method returns an Object
type, and at compile-time, the compiler cannot guarantee that the Object
is indeed a HashMap<String, String>
. Hence, it throws that little warning at you, just to let you know that there might be some mischief happening under the hood. 🚨
Easy Solutions to Silence the Warning 👊
Don't fret! There are a couple of easy and safe solutions to get rid of that pesky warning. Let's explore them:
Solution 1: Use the Correct Map Type 💡
Instead of declaring someMap
as a HashMap<String, String>
, you can declare it as Map<String, String>
. This way, you're programming against an interface, which provides more flexibility and allows you to swap implementation types without any casting headaches. Here's how it would look:
private Map<String, String> someMap = new HashMap<String, String>();
someMap = getApplicationContext().getBean("someMap");
By doing this, you're essentially treating someMap
as a Map
, and the warning magically disappears! 🪄
Solution 2: Use ParameterizedTypeReference 💡
Another fancy solution is to use the ParameterizedTypeReference
class provided by Spring. This class helps you retain the generic type information during the bean retrieval process. To use it, you need to tweak the getBean()
method call a bit. Check it out:
private Map<String, String> someMap = new HashMap<String, String>();
someMap = getApplicationContext().<Map<String, String>>getBean("someMap");
Now, with the help of ParameterizedTypeReference
, the warning is gone for good! 🎉
Wrap Up and Get Rid of Those Warnings! 🎁
Congratulations, you made it to the end of our journey! We hope you now understand what the infamous "Type safety: Unchecked cast" warning is all about. Remember, this warning is just the compiler's way of keeping you safe from potential type-based disasters.
But fear not, we've provided you with two easy solutions to conquer this warning and reclaim your peace of mind. So go ahead, pick your favorite solution, apply it to your code, and enjoy a warning-free coding experience! 🚀
If you found this blog post helpful, feel free to share it with your fellow developers who might be facing the same warning. And don't forget to leave a comment below to let us know your thoughts and any other cool tricks you have up your sleeve to tackle this issue. Happy coding! 😄✨