Testing two JSON objects for equality ignoring child order in Java
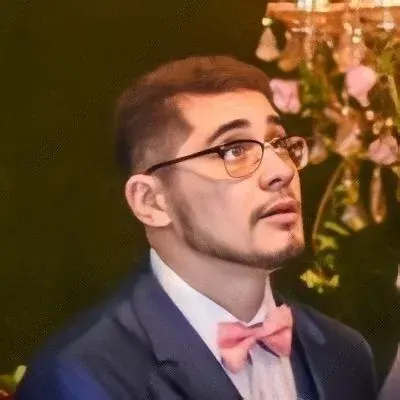
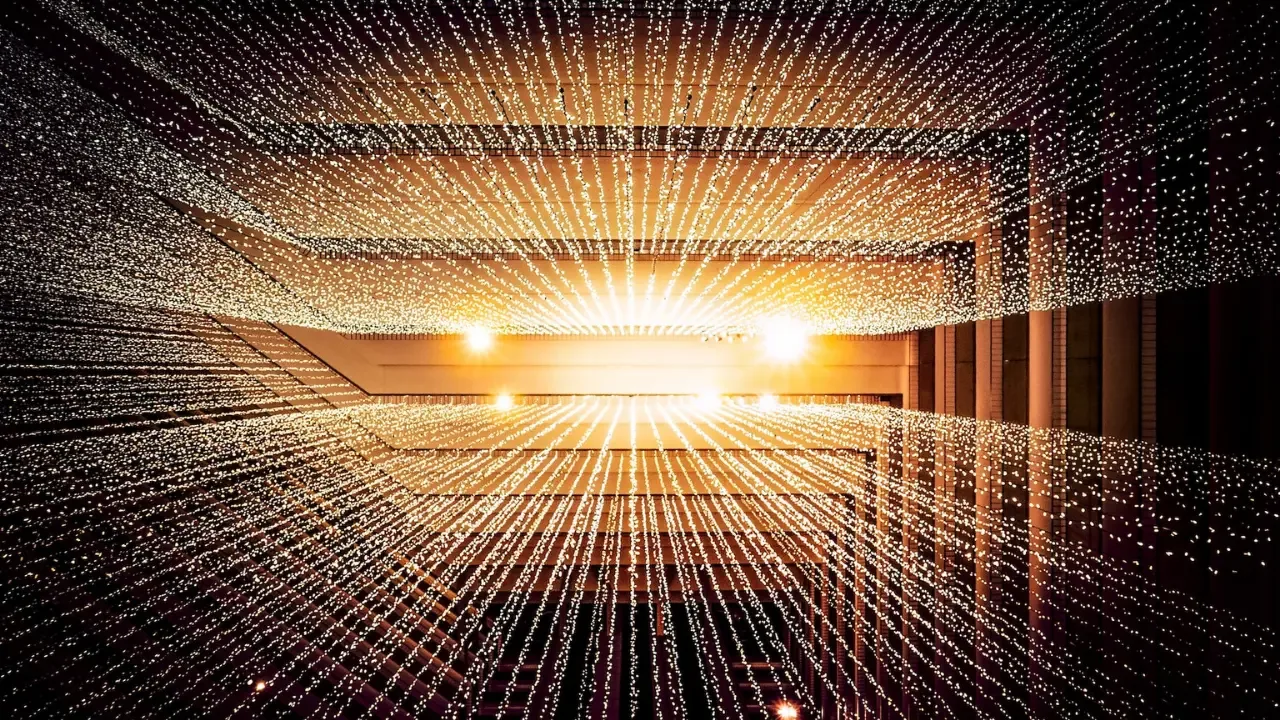
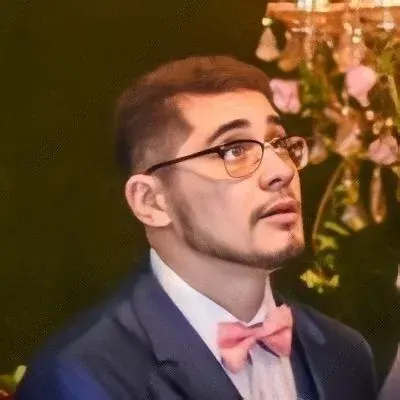
Title: Crushing the JSON Comparison Madness in Java π
Introduction π
Welcome to the mind-bending world of JSON comparison in Java! π€― If you've ever struggled with comparing two JSON objects, specifically when the child order doesn't matter, then you're in the right place! In this blog post, we will dive deep into this issue and provide you with easy solutions to conquer this challenge. Let's make JSON comparison a breeze! πͺ
The Quest for JSON Equality πΊοΈ
Problem: You need to compare two JSON objects in Java, but their child order shouldn't affect the equality. Moreover, you want a JSON parsing library that can handle this scenario gracefully, especially for unit testing JSON responses from web services.
Solution: Fortunately, there are libraries out there that can ride this JSON equality rollercoaster. Let's explore some popular options together. π’
JSON Libraries to the Rescue! π¦ΈββοΈ
Gson: Gson is a widely-used JSON library in the Java ecosystem. While it doesn't provide built-in support for ignoring child order during comparison, we can leverage its powerful features to achieve our goal. Here's a code snippet to get you started:
Gson gson = new Gson(); JsonElement jsonElement1 = gson.fromJson(jsonString1, JsonElement.class); JsonElement jsonElement2 = gson.fromJson(jsonString2, JsonElement.class); boolean isEqual = jsonElement1.equals(jsonElement2);
By converting the JSON strings into
JsonElement
, we can utilize theequals()
method to perform equality comparison that ignores child order. Simple, right? πJackson: Another heavyweight contender in the JSON realm is Jackson. Similar to Gson, Jackson doesn't have an out-of-the-box method to compare JSON objects while ignoring child order. Nonetheless, we can employ some Jackson magic to achieve our desired outcome. Check out this code snippet:
ObjectMapper objectMapper = new ObjectMapper(); JsonNode jsonNode1 = objectMapper.readTree(jsonString1); JsonNode jsonNode2 = objectMapper.readTree(jsonString2); boolean isEqual = jsonNode1.equals(jsonNode2);
By using
JsonNode
from Jackson, we can leverage itsequals()
method for JSON comparison that ignores child order. It's like having a superpower, isn't it? π¦ΈββοΈ
The Unveiling of the Ultimate Solution π
Now, what if I told you there's a library solely dedicated to solving this JSON equality problem? Yes, it exists, and it's called JSONassert! π JSONassert provides a concise and elegant solution for comparing JSON objects while ignoring child order. Let's see how it works:
String jsonString1 = "{ 'name': 'John', 'age': 30 }";
String jsonString2 = "{ 'age': 30, 'name': 'John' }";
JSONAssert.assertEquals(jsonString1, jsonString2, JSONCompareMode.LENIENT);
By utilizing JSONAssert.assertEquals()
with the JSONCompareMode.LENIENT
option, we can peacefully compare JSON objects regardless of child order. JSONassert handles all the nitty-gritty details for us! π
In Closing π
Congratulations, my fellow Java developer, you are now equipped with the knowledge to conquer the JSON comparison madness! Whether you opt to leverage the features of Gson or Jackson or unleash the power of JSONassert, rest assured that JSON equality with ignoring child order is within your grasp. π₯
Now, go forth and empower your unit tests, tame those unruly JSON objects, and share your success stories! Don't forget to let me know in the comments which approach worked best for you. Together, we can make the Java world a better place, one JSON at a time! πβ€οΈ
π₯ Call to Action: Have you encountered other JSON-related challenges? Or do you want to learn more about JSON parsing in Java? Subscribe to our newsletter to receive more mind-blowing tech content and join our vibrant community of developers. Let's level-up together! ππ©