Spring @Transactional - isolation, propagation
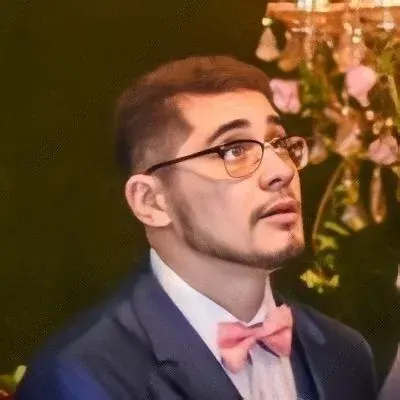
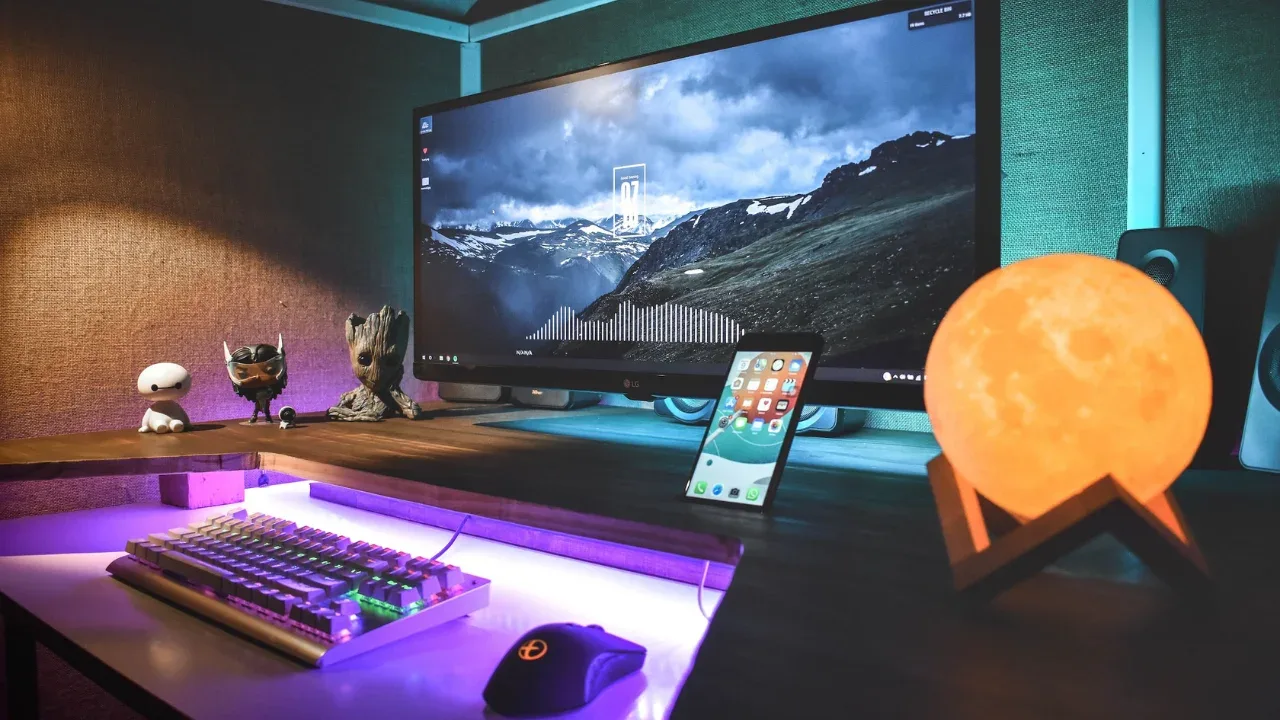
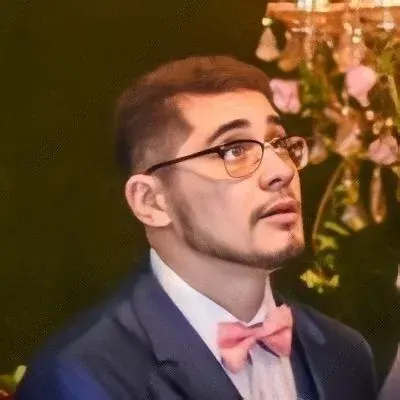
Understanding Spring @Transactional: Isolation and Propagation Explained with Real-World Examples
<p>Imagine this: you're designing a banking application and you have a method that transfers money from one account to another. Now, what if two threads simultaneously trigger this method? This could lead to inconsistencies in the account balances.</p>
<p>Transaction management plays a crucial role in handling scenarios like this, ensuring data integrity and concurrency control. In Spring, the <code>@Transactional</code> annotation comes to the rescue. It allows you to declaratively define transaction management for your methods.</p>
What is Isolation?
<p>Isolation deals with how concurrent transactions interact with each other when multiple transactions are happening simultaneously.</p>
<p>Let's dive into the different isolation levels you can choose from:</p>
1. Read Uncommitted
<p>This is the lowest isolation level. It allows dirty reads, meaning that one transaction can see uncommitted changes made by other concurrent transactions. In our banking application example, if one transaction is updating an account balance, another transaction in the "Read Uncommitted" isolation level could see that change before it is committed.</p>
2. Read Committed
<p>This isolation level ensures that a transaction sees only the committed changes made by other transactions, eliminating dirty reads. In our banking application example, if one transaction is updating an account balance, a transaction in the "Read Committed" isolation level will only see the updated balance after it is committed.</p>
3. Repeatable Read
<p>This isolation level ensures that a transaction will always see the same data throughout its execution, even if other transactions make changes to the same data. In our banking application example, if one transaction transfers funds between accounts, a transaction in the "Repeatable Read" isolation level will still see the same account balances throughout its execution, regardless of any other updates happening concurrently.</p>
4. Serializable
<p>This is the highest isolation level, ensuring that transactions are executed serially, one at a time. It provides the strictest form of isolation. In our banking application example, if two transactions simultaneously attempt to transfer funds from the same account using this isolation level, one of them will need to wait until the other completes.</p>
What is Propagation?
<p>Propagation determines how a transaction behaves when it encounters an existing transaction context.</p>
<p>Here are the various propagation options:</p>
1. Propagation.REQUIRED
<p>This is the default propagation value in Spring. It means that if a transactional method is called within an existing transaction context, it will participate in that transaction. If a transactional method is called without an existing transaction context, a new transaction will be created.</p>
2. Propagation.REQUIRES_NEW
<p>This propagation value always creates a new transaction. It suspends the current transaction, if any, and starts a new one. This can be useful in scenarios where you want to ensure that a specific block of code is executed in its own separate transaction, regardless of any outer transactional context.</p>
3. Propagation.MANDATORY
<p>This propagation value states that a transactional method must be invoked within an existing transaction context. If there is no surrounding transaction, an exception will be thrown. Use this when you need to enforce that a method should always run within a transactional context.</p>
4. Propagation.NESTED
<p>This propagation value allows nested transactions within an existing transaction. The nested transactions can be rolled back independently or as part of the outer transaction. It provides a savepoint mechanism within the transaction. If the outer transaction is rolled back, all nested transactions and their changes will be rolled back as well.</p>
Choosing the Right Values for Isolation and Propagation
<p>Now that we understand the concept of isolation and propagation, let's take a step back and consider when and why we should change their default values.</p>
<p>The default values of isolation and propagation are usually sufficient for most scenarios. However, there may be cases where you need to fine-tune them to achieve specific requirements for data consistency and transaction behavior.</p>
<p>Here are a few scenarios where you might consider customizing the isolation and propagation levels:</p>
Highly concurrent systems: If you're working on a system that experiences a high volume of concurrent transactions, you might need to adjust the isolation level to prevent conflicts and ensure data integrity. For example, choosing the "Serializable" isolation level may be necessary to ensure that critical resources are accessed in a serialized manner.
Partial rollback requirements: If you have a method that performs multiple independent operations, and you want some of them to roll back while others persist, you can leverage the "Propagation.NESTED" propagation value. This allows you to create nested transactions and selectively roll them back.
Enforcing transaction boundaries: In some cases, you may want to enforce that a specific method always executes within a transactional context. This can be achieved by setting the "Propagation.MANDATORY" propagation value, which will throw an exception if the method is invoked without a surrounding transaction.
<p>Remember, the choice of isolation and propagation levels depends on your specific use case and requirements. Always thoroughly analyze your application's needs before customizing these settings.</p>
Conclusion
<p>Understanding and utilizing the <code>@Transactional</code> annotation's isolation and propagation parameters is essential for effective transaction management in your Spring applications. By correctly setting the isolation level, you can prevent data inconsistencies, and by choosing the appropriate propagation behavior, you can handle nested transactions and enforce necessary transactional boundaries.</p>
<p>Experiment with the different isolation and propagation levels in your own projects to gain a deeper understanding of their impact on your application's behavior.</p>
<p>Now it's your turn! Have you encountered any challenges with Spring's <code>@Transactional</code> annotation? What are some other scenarios where customizing isolation and propagation levels can be useful? Share your thoughts and experiences in the comments below!</p>
🔗 Check out our previous blog post on Spring Boot's AutoConfiguration magic: link