Spring @Transaction method call by the method within the same class, does not work?
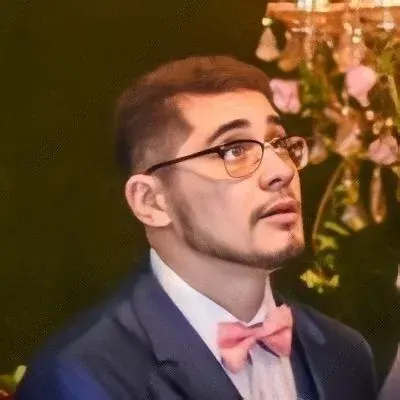
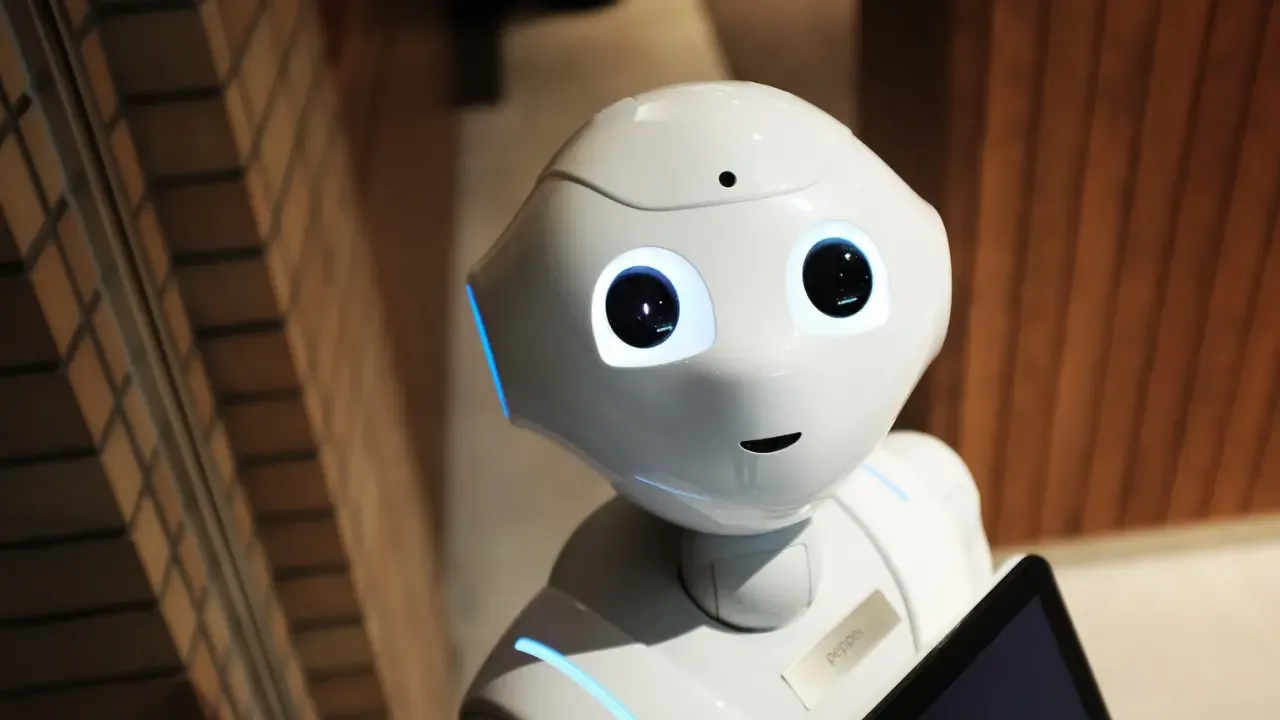
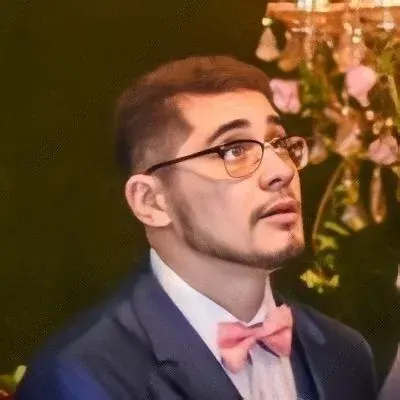
🌼 Understanding the issue with calling a @Transaction method within the same class in Spring 🌼
So you stumbled upon an interesting situation while working with Spring Transactions, huh? It seems that you're having trouble calling a method with the @Transactional annotation within the same class. Don't worry, you're not alone in this confusion! 🤔
The code snippet you shared gave us a clear understanding of what you're facing. Let's break it down and dive into the issue you're experiencing. 😎
🤷♂️ The problem
In your UserService class, you have defined two methods: addUser
and addUsers
. The addUser
method is annotated with @Transactional to ensure transactional behavior. However, when you call addUser
from the addUsers
method within the same UserService class, the transactional behavior seems to be not working. 🙅♂️
🤔 Understanding the issue
Indeed, when you call a @Transactional method from within the same class, Spring cannot apply transactional behavior to that method. Why? Because Spring works as a proxy for your beans, introducing dynamic proxies to manage transactions. These proxies can only intercept calls to @Transactional methods when they come from another bean. 😮
✨ The solution
Luckily, there's a straightforward solution to this problem! To make the @Transactional annotation work when calling a method within the same class, you need to refactor your code slightly. Consider creating a separate bean (another class) that will act as the caller for your transactional method. This way, Spring can intercept the call and apply the transactional behavior properly. 🌟
Here's an updated version of your code to demonstrate how to resolve this issue:
public class UserService {
@Autowired
private UserCaller userCaller;
public boolean addUsers(List<User> users) {
for (User user : users) {
userCaller.addUser(user.getUserName, user.getPassword);
}
}
}
@Component
public class UserCaller {
@Transactional
public boolean addUser(String userName, String password) {
try {
// call DAO layer and add to the database.
} catch (Throwable e) {
TransactionAspectSupport.currentTransactionStatus()
.setRollbackOnly();
}
}
}
By creating the UserCaller
class, we moved the addUser
method to a separate bean. Now, when addUser
is called from the addUsers
method in the UserService
class, Spring's proxy mechanism will work correctly, and the transactional behavior will be applied. Problem solved! 🎉
🚀 Take action and engage!
I hope this guide helped you understand and resolve the issue with calling a @Transactional method from within the same class. If you have any further questions or face any other roadblocks, feel free to reach out. I'm here to help! 🙌
Have you faced any similar Spring Transaction challenges? Share your experiences and solutions in the comments section below. Let's learn from each other and conquer Spring together! 🌱💪
Stay tuned for more exciting tech tips, guides, and solutions. Don't forget to subscribe and share this blog post with your fellow developers. Together, we can make the tech world a better place! 🌍✨
Until next time, happy coding! 💻🚀