Spring - No EntityManager with actual transaction available for current thread - cannot reliably process "persist" call
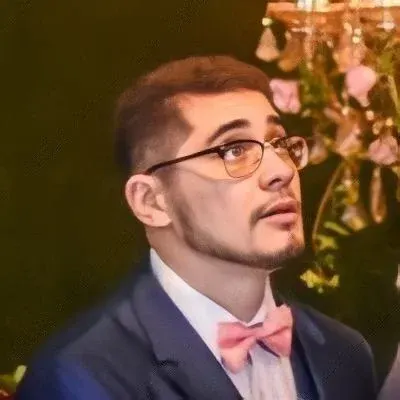

Spring - No EntityManager with actual transaction available for current thread - cannot reliably process 'persist' call
So, you're working on your Spring MVC web application, and you encounter this error when trying to save an entity model to the database using the "persist" method. You've searched the internet but can't find any relevant solutions. Don't worry, we've got you covered! In this blog post, we'll address the common issues and provide easy solutions to fix this error.
Understanding the Problem
This error usually occurs when there is no EntityManager with an actual transaction available for the current thread. It means that something is going wrong with the EntityManagerFactory bean, which is responsible for managing the EntityManager instances in your application.
Diagnosis: Looking into the Code
Let's take a look at the code provided to identify any potential issues.
dispatcher-servlet.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:jpa="http://www.springframework.org/schema/data/jpa"
xsi:schemaLocation="
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-4.0.xsd
...
"
>
<!-- Component scanning and bean definitions -->
<!-- EntityManagerFactory bean definition -->
<bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean">
...
</bean>
<!-- Other bean definitions -->
<mvc:annotation-driven />
<!-- View resolver and resource mappings -->
</beans>
RegisterController.java
@Controller
public class RegisterController {
@PersistenceContext
EntityManager entityManager;
...
@RequestMapping(value = "/addUser", method = RequestMethod.POST)
public String register(@ModelAttribute("person") @Valid @Validated Person person, BindingResult result) {
if(result.hasErrors()) {
return "register";
} else {
entityManager.persist(person);
return "index";
}
}
}
From the code, we can see that:
We have defined the
entityManagerFactory
bean in thedispatcher-servlet.xml
file.In the
RegisterController
class, we have injected theEntityManager
using@PersistenceContext
annotation.
Solutions: Fixing the Error
Based on our diagnosis, there are a few potential solutions to fix this error.
Solution 1: Configure Transaction Management
To ensure that an actual transaction is available for the current thread, we need to configure transaction management in our Spring application.
Add the following bean definition in
dispatcher-servlet.xml
:
<bean id="transactionManager" class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory" ref="entityManagerFactory" />
</bean>
Add the
@Transactional
annotation to theregister
method inRegisterController.java
:
@Transactional
@RequestMapping(value = "/addUser", method = RequestMethod.POST)
public String register(@ModelAttribute("person") @Valid @Validated Person person, BindingResult result) {
...
}
Solution 2: Verify EntityManagerFactory Configuration
It's possible that the configuration for the entityManagerFactory
bean is incorrect. Double-check the following:
Verify that the
persistenceXmlLocation
property in theentityManagerFactory
bean refers to a valid location of yourpersistence.xml
file.Ensure that the
dataSource
bean is correctly configured with the appropriate properties for your database connection.
Solution 3: Check Dependencies and Versions
Make sure that you have the required dependencies and compatible versions for Spring MVC, Spring Data JPA, and Hibernate in your project.
Check your project's dependencies and ensure that you have the necessary JAR files.
Verify that the versions of Spring MVC, Spring Data JPA, and Hibernate are compatible.
Put Your Solutions to Action
After applying one or more of these solutions, recompile and run your application to see if the error is resolved. If you encounter any other issues or have further questions, feel free to leave a comment below.
Conclusion
In this blog post, we discussed the common issue of getting the "No EntityManager with actual transaction available for current thread" error when trying to persist an entity model in a Spring MVC web application. We explored possible causes and provided easy-to-implement solutions to fix the error. Now it's your turn! Apply these solutions to your code and test it out. Share your experience, questions, or any other insights in the comments section.
Happy coding! 👨💻💥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
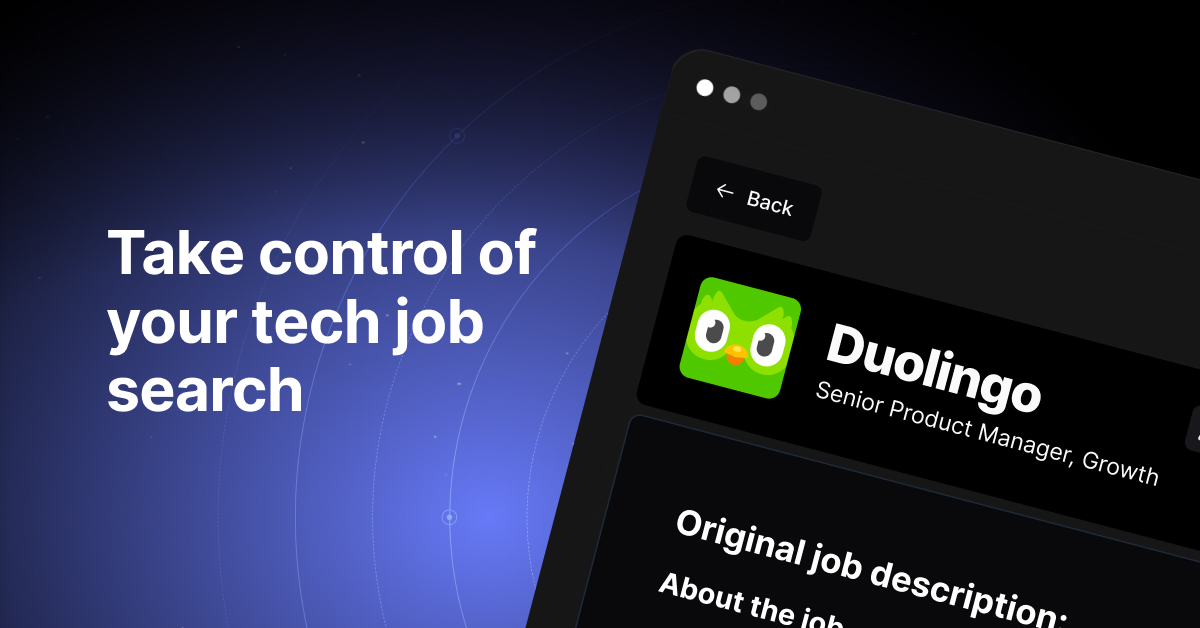