Spring MVC type conversion : PropertyEditor or Converter?
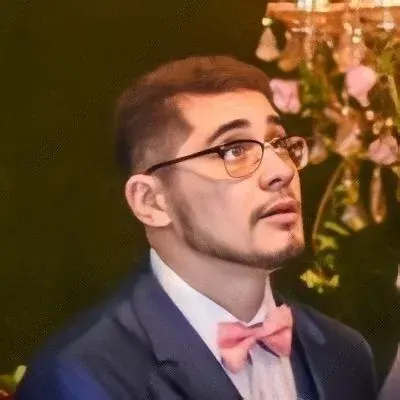
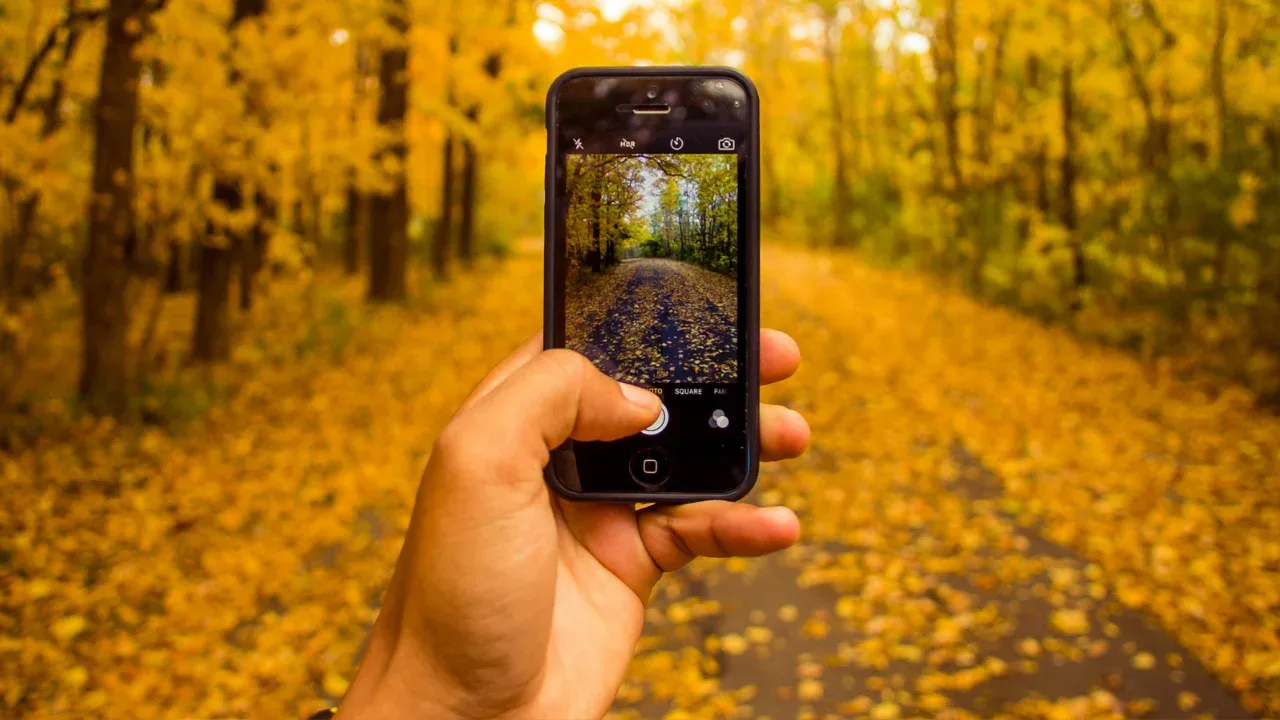
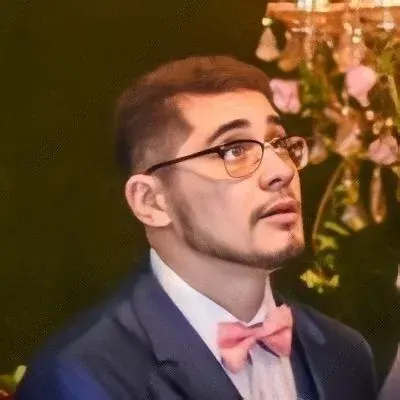
Spring MVC Type Conversion: PropertyEditor or Converter?
Are you working with Spring MVC and facing issues with data binding and conversion? Do you find it frustrating to configure XML or write lengthy code to handle conversions? Don't worry, we've got you covered! In this blog post, we will explore two different approaches to handle type conversion in Spring MVC: PropertyEditors and Converters. We'll discuss the pros and cons of each approach and provide easy solutions to common problems.
PropertyEditor: The Traditional Approach š°
If you are already using Spring MVC, you might be familiar with PropertyEditors. In the past, PropertyEditors were the go-to solution for data binding and conversion. They provide a straightforward way to convert data types, without requiring any XML configurations. Let's take a closer look at how PropertyEditors work.
public class CategoryEditor extends PropertyEditorSupport {
// Converts a String to a Category (when submitting form)
@Override
public void setAsText(String text) {
Category c = new Category(text);
this.setValue(c);
}
// Converts a Category to a String (when displaying form)
@Override
public String getAsText() {
Category c = (Category) this.getValue();
return c.getName();
}
}
In the example above, we have a CategoryEditor
that extends PropertyEditorSupport
. It provides methods to convert a String
to a Category
object and vice versa. To bind this editor to a specific field in your controller, you can use the @InitBinder
annotation.
@InitBinder
public void initBinder(WebDataBinder binder) {
binder.registerCustomEditor(Category.class, new CategoryEditor());
}
This way, the conversion logic defined in CategoryEditor
will be applied to the corresponding field.
The New Kid on the Block: Converters š
With the release of Spring 3.x, a new alternative to PropertyEditors was introduced ā Converters. Converters provide a modern, type-safe approach to handle type conversion in Spring MVC. Let's take a look at how Converters work.
public class StringToCategory implements Converter<String, Category> {
@Override
public Category convert(String source) {
Category c = new Category(source);
return c;
}
}
public class CategoryToString implements Converter<Category, String> {
@Override
public String convert(Category source) {
return source.getName();
}
}
In the example above, we have two Converters: StringToCategory
and CategoryToString
. These converters implement the Converter
interface and provide type-specific conversion logic. Note that with Converters, you don't need to cast the values, thanks to genericity.
Now you might be wondering how to bind these Converters to specific fields in your controller. This is where things get a bit tricky compared to PropertyEditors.
Binding Converters: The Tedious Way š¤
One drawback of using Converters is that there is no simple annotation or programmatic facility to bind them directly in a controller. The most common approach is to configure them using XML or Java config. Let's explore both options.
XML Configuration
<bean id="conversionService" class="org.springframework.context.support.ConversionServiceFactoryBean">
<property name="converters">
<set>
<bean class="com.example.StringToCategory" />
<bean class="com.example.CategoryToString" />
</set>
</property>
</bean>
By defining a conversionService
bean with the required converters, you can configure the type conversion at the application level.
Java Configuration
@EnableWebMvc
@Configuration
public class WebConfig extends WebMvcConfigurerAdapter {
@Override
protected void addFormatters(FormatterRegistry registry) {
registry.addConverter(new StringToCategory());
registry.addConverter(new CategoryToString());
}
}
If you are using Spring 3.1 or higher, you can leverage Java config by extending WebMvcConfigurerAdapter
and overriding the addFormatters
method.
Pros and Cons: Which One to Choose? š¤·āāļø
Now that we have discussed both PropertyEditors and Converters, let's weigh the pros and cons of each approach to help you make an informed decision.
PropertyEditor Pros:
ā Simple setup, no XML or Java config required ā Straightforward binding within the same class ā Easy to do a general binding across all controllers with just a few lines of XML configuration
PropertyEditor Cons:
ā Requires separate classes for each conversion ā May lead to casting issues
Converter Pros:
ā Type-safe and modern approach ā No casting required ā More control over the conversion process
Converter Cons:
ā Tedious setup, requires XML or Java config ā No simple way to bind converters directly in a controller
Conclusion and Call-to-Action š
While Converters provide a more modern and type-safe approach to type conversion in Spring MVC, they do come with some drawbacks, especially in terms of binding and configuration. On the other hand, PropertyEditors offer a simpler setup but may lead to casting issues and require separate classes for each conversion.
In most cases, PropertyEditors should suffice for simple conversions within a specific field. However, if you need more control over the conversion process or want to avoid casting, Converters might be a better option.
We hope this blog post has helped you understand the differences between PropertyEditors and Converters in Spring MVC. If you have any questions or would like to share your experiences, feel free to leave a comment below. Happy coding! šš»