Spring MVC @PathVariable getting truncated
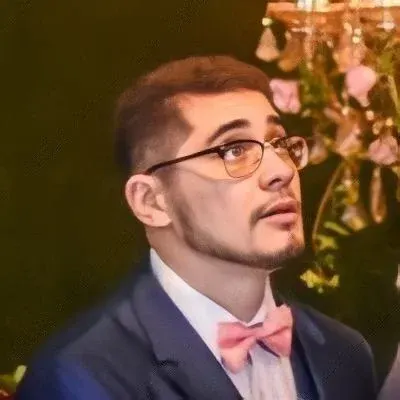
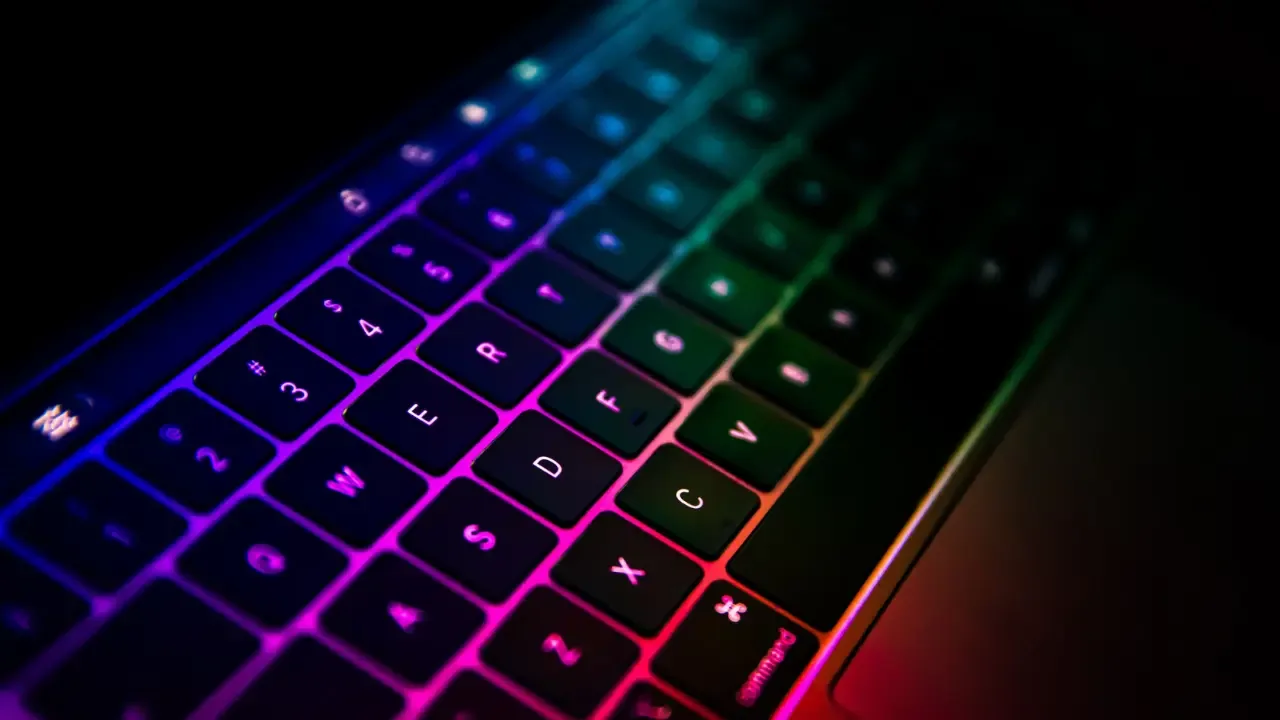
🖊️ Hey there tech enthusiasts! 👋 Welcome back to my tech blog! In today's post, we're going to tackle a common problem encountered by many developers when using Spring MVC's @PathVariable
annotation. So, if you've ever faced the issue of your path variables getting truncated, fear not! 🚫✂️ We've got some easy solutions for you! Let's dive right in! 💪
The problem at hand revolves around a RESTful controller that provides access to some valuable information. Here's a code snippet to give you some context:
@RequestMapping(method = RequestMethod.GET, value = Routes.BLAH_GET + "/{blahName}")
public ModelAndView getBlah(@PathVariable String blahName, HttpServletRequest request,
HttpServletResponse response) {
Now, here's where the trouble starts. If you hit the server with a path variable containing special characters, it gets truncated. 😱 For example, when accessing the following URL:
http://localhost:8080/blah-server/blah/get/blah2010.08.19-02:25:47
The blahName
parameter in the getBlah
method will get set to blah2010.08
. 😦 However, worry not! The actual path information is still available via request.getRequestURI()
. Phew! 😅
But now, the burning question arises: How do we prevent Spring from truncating our precious @PathVariable
? 🤔
🔧 Thankfully, there are a couple of easy solutions to this predicament:
1️⃣ Encoding the path variable: By encoding the path variable using UriUtils.encodePathSegment
method from Spring, we can ensure that special characters are properly handled. You simply need to modify your code like this:
public ModelAndView getBlah(@PathVariable("blahName") String encodedBlahName, HttpServletRequest request,
HttpServletResponse response) {
String blahName = UriUtils.decode(encodedBlahName, StandardCharsets.UTF_8);
// Rest of the logic
2️⃣ Using regular expressions in @RequestMapping
: Another handy solution is to use regular expressions to define the allowed characters in your path variable. For instance, if you only want to allow alphanumeric characters, dots, and colons, you can modify your code like this:
@RequestMapping(method = RequestMethod.GET, value = Routes.BLAH_GET + "/{blahName:[\\w\\.\\:]+}")
public ModelAndView getBlah(@PathVariable String blahName, HttpServletRequest request,
HttpServletResponse response) {
// Rest of the logic
And voila! ✨ Your path variable won't get truncated anymore!
📣 And that's a wrap, folks! 🎉 We hope this guide helped you overcome the issue of Spring MVC's @PathVariable
getting truncated. Now you can handle those special characters without any worries. If you found this post useful, don't forget to share it with your fellow developers and spread the knowledge! 🤩💡
💌 We'd love to hear your thoughts on this topic! Have you ever encountered this problem? How did you solve it? Let us know in the comments below. Let's engage in a meaningful discussion! 💬😊
And as always, stay tuned for more exciting tech tips and tricks. Until next time, happy coding! ⌨️🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
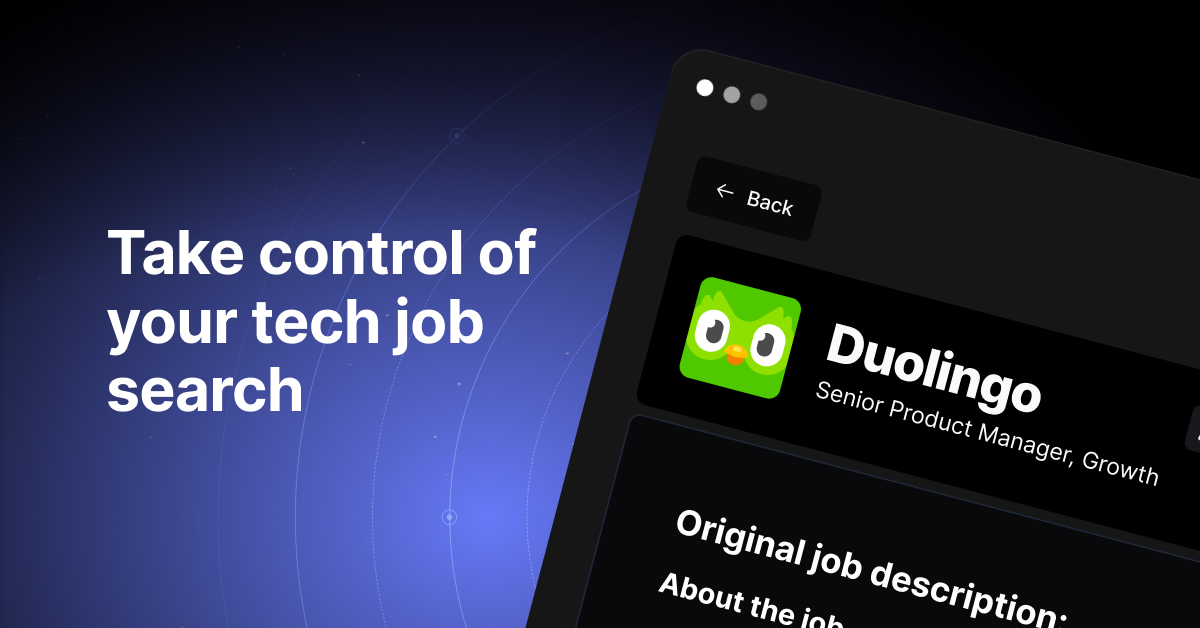