Spring MVC - How to return simple String as JSON in Rest Controller
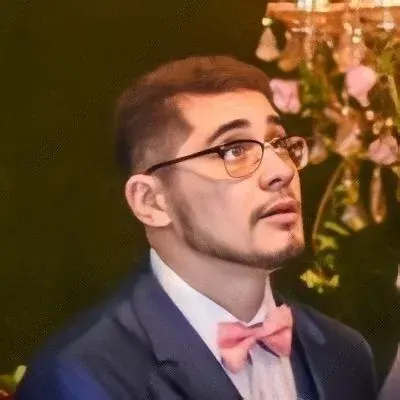
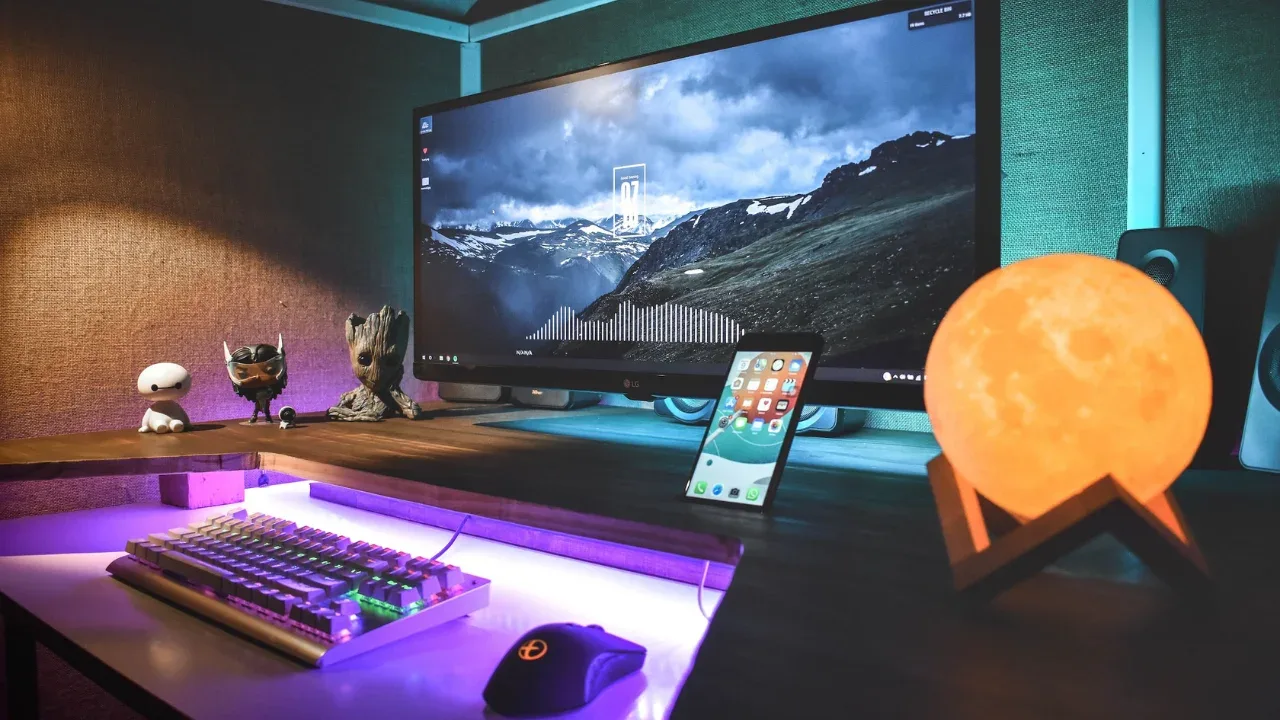
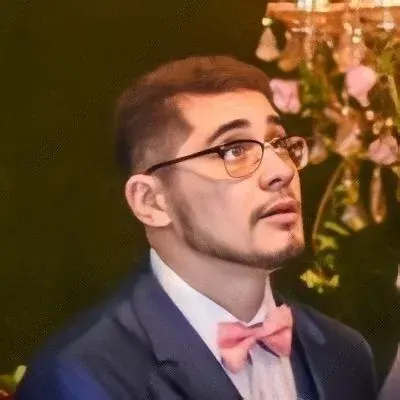
How to Return a Simple String as JSON in a Spring MVC Rest Controller ππ‘
So you have a Spring MVC Rest Controller and you want to return a simple string as JSON in the response body. You might think it's as easy as returning the string directly, but Spring will add the string into the response body as is, without any JSON formatting. But worry not, there's a straightforward solution! π
The Hacky Way with Quotes π€βοΈ
One way to achieve this is by adding quotes to the string manually. You can do this by simply appending "
before and after the string. But let's be honest, that feels like a hack and can get messy if you have complex strings or need to return multiple variables. So, let's explore a cleaner approach. πͺ
The Jackson Library to the Rescue! π¦ΈββοΈπ
To return a simple string as JSON, you can leverage the powerful Jackson library, which Spring provides out of the box for JSON serialization and deserialization. Here's what you need to do: π οΈ
Add the Jackson dependency to your project. If you're using Maven, add the following dependency to your
pom.xml
file:<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>{jackson-version}</version> </dependency>
Replace
{jackson-version}
with the appropriate version of Jackson you're using.Annotate your controller method with
@ResponseBody
to indicate that the return value should be written directly to the response body. This saves you from manually writing to the response body yourself!@RestController public class TestController { @RequestMapping("/getString") public @ResponseBody String getString() { return "Hello World"; } }
That's it! By annotating your method with @ResponseBody
, Spring will automatically use Jackson to serialize your plain string into valid JSON. π
Example: Returning a String as JSON π
Let's see an example of how this works in action:
@RestController
public class TestController {
@RequestMapping("/getString")
public @ResponseBody String getString() {
return "Hello World";
}
}
When you make a request to /getString
, the response body will contain the following JSON:
"Hello World"
Notice the quotes around the string? That's how Jackson serializes your plain string into valid JSON format π
Add Some Swagger to Your JSON ππ₯
Now that you know how to return a simple string as JSON in your Spring MVC Rest Controller, why not take it a step further and make your API documentation more engaging? Swagger is an awesome tool that automatically generates API documentation based on annotations in your code.
Try adding Swagger to your project to create beautiful and interactive documentation for your API! You can find more information and examples on the official Swagger website: https://swagger.io π
So go ahead, give it a try, and level up your JSON game with Spring MVC! Happy coding! πβ¨
Have any questions or faced any issues? Feel free to drop a comment below and let's discuss! π¬π