Spring MVC - How to get all request params in a map in Spring controller?
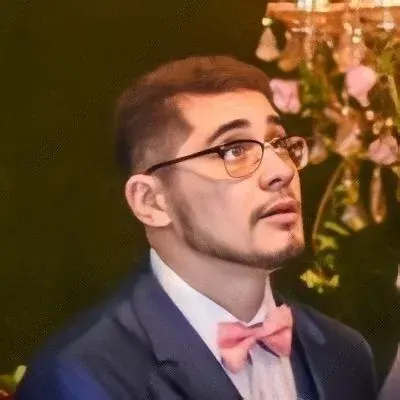
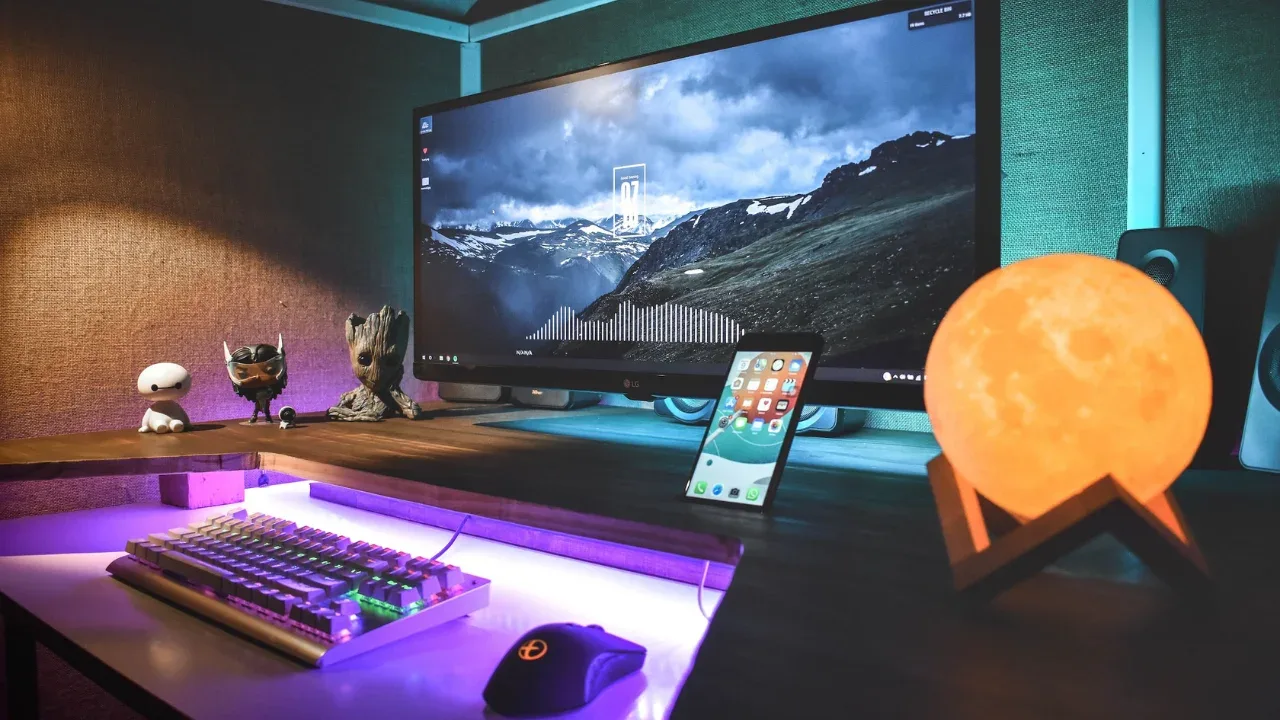
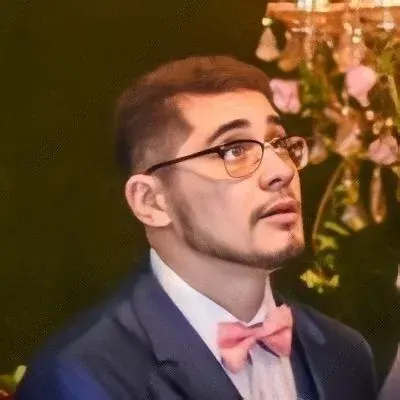
πΊπ Hey there tech enthusiasts! Have you ever found yourself in a situation where you want to capture all the request parameters in a map in your Spring controller, but you don't have access to the parameter names? Fear not! In this blog post, we will discuss a super easy solution to this common problem. So let's dive right in! πͺ
The scenario we have is a user accessing a URL like this: ../search/?attr1=value1&attr2=value2&attr4=value4
. But the catch is, we don't know the names of attr1
, attr2
, and attr4
in advance. How can we handle this in our Spring MVC controller?
π The Solution:
To solve this problem, we can make use of the HttpServletRequest
object and the @RequestParam
annotation in Spring MVC. Let's see how it's done step by step.
1οΈβ£ First, ensure that you have the HttpServletRequest
object as a parameter in your controller method. This can be achieved by adding it as an argument in your method signature, just like this:
@RequestMapping(value = "/search", method = RequestMethod.GET)
public void search(HttpServletRequest request, ModelMap model) {
// Handle request parameters here
}
2οΈβ£ Next, inside your controller method, create a Map<String, String>
object to store the request parameter names and their corresponding values. Let's call it allRequestParams
:
Map<String, String> allRequestParams = new HashMap<>();
3οΈβ£ Now, iterate over all the request parameters using the getParameterMap()
method of the HttpServletRequest
object:
Map<String, String[]> requestParams = request.getParameterMap();
for (Map.Entry<String, String[]> entry : requestParams.entrySet()) {
String paramName = entry.getKey();
String paramValue = entry.getValue()[0]; // Since we are using a Map<String, String>
allRequestParams.put(paramName, paramValue);
}
4οΈβ£ And that's it! You now have all the request parameter names and values stored in the allRequestParams
map. Feel free to use this map for further processing or to pass it to any other methods or services as needed.
π Here's an example summary of the complete solution:
@RequestMapping(value = "/search", method = RequestMethod.GET)
public void search(HttpServletRequest request, ModelMap model) {
Map<String, String> allRequestParams = new HashMap<>();
Map<String, String[]> requestParams = request.getParameterMap();
for (Map.Entry<String, String[]> entry : requestParams.entrySet()) {
String paramName = entry.getKey();
String paramValue = entry.getValue()[0];
allRequestParams.put(paramName, paramValue);
}
// Further processing or passing the map to other methods/services
}
π‘ So there you have it! You now know how to easily capture all request parameters in a map, even when you don't know their names in advance.
π₯ Now it's your turn! Give this solution a try and let us know how it works for you. Got any other cool tips or tricks related to Spring MVC? Share them in the comments below! If you found this blog post helpful, don't forget to share it with your fellow developers. Keep coding and stay awesome! β¨π