Spring Java Config: how do you create a prototype-scoped @Bean with runtime arguments?
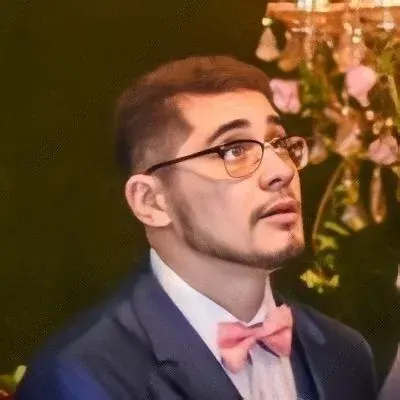
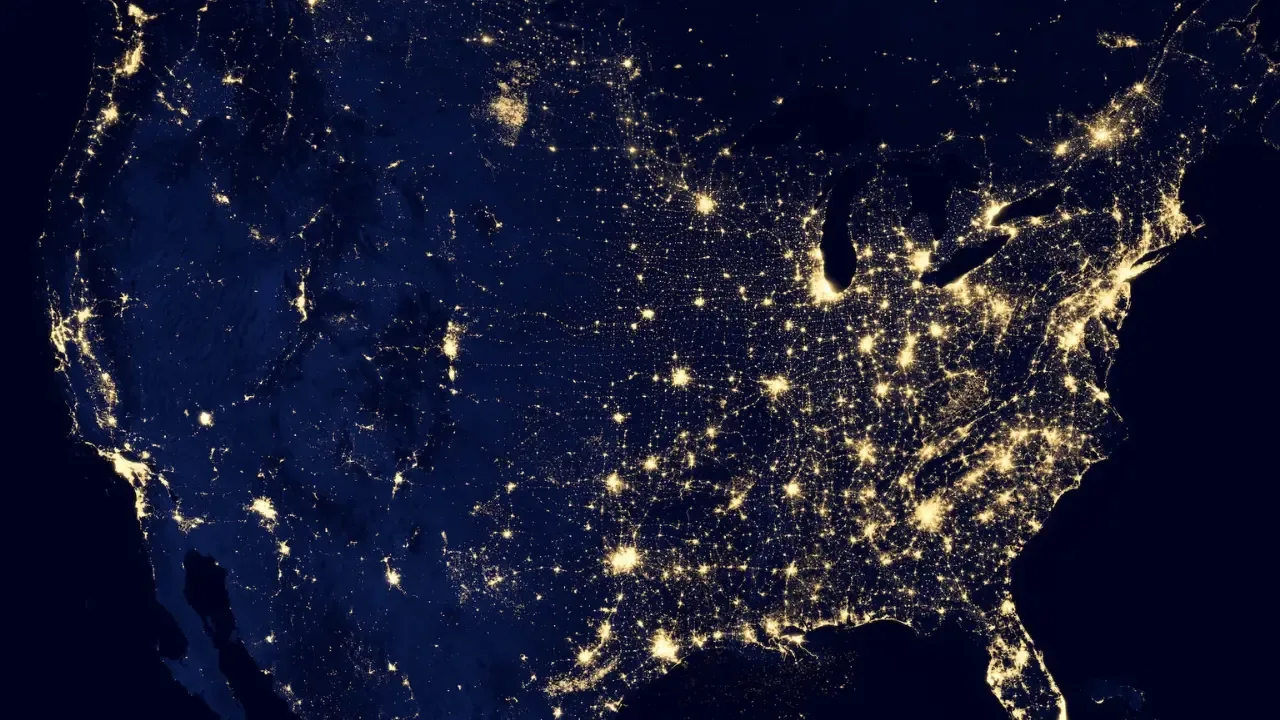
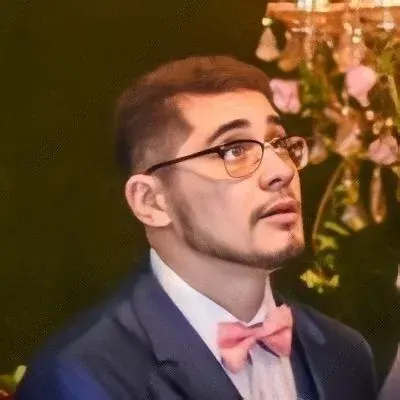
Creating a Prototype-Scoped @Bean with Runtime Arguments in Spring Java Config
Are you struggling to create a prototype-scoped @Bean
with runtime arguments in Spring Java Config? Don't worry, I've got you covered! 🙌 In this blog post, I'll address this common issue and provide you with an easy solution. Let's dive in! 💻
The Problem: Runtime Arguments with Prototype-Scoped Bean
You have a scenario where you need to acquire or instantiate a prototype-scoped bean with constructor arguments that are only obtainable at runtime. In your code example, you are using Spring's Java Config with the ApplicationContext
to get the Thing
bean with the name
argument.
@Autowired
private ApplicationContext appCtx;
public void onRequest(Request request) {
String name = request.getParameter("name");
Thing thing = appCtx.getBean(Thing.class, name);
// ...
}
The Thing
class has a constructor that takes the name
argument, and the other dependencies (SomeComponent
and AnotherComponent
) are autowired inside the class. This code works perfectly fine with Spring XML config, but how can you achieve the same with Java Config?
The Solution: Custom Factory Method Bean
By default, Spring Java Config does not support passing runtime arguments directly to the @Bean
method. However, there's an easy and elegant way to achieve this by creating a custom factory method bean. Let's see how!
Step 1: Create a Factory Interface
First, create a factory interface that defines the method for creating the Thing
bean. This interface will serve as the contract for the factory implementation.
public interface ThingFactory {
Thing createThing(String name);
}
Step 2: Implement the Factory Interface
Next, implement the ThingFactory
interface in a class and annotate it with @Component
to make it a Spring-managed bean. In the implementation, create the Thing
bean using the runtime argument(s) and return it.
@Component
public class ThingFactoryImpl implements ThingFactory {
@Override
public Thing createThing(String name) {
return new Thing(name);
}
}
Step 3: Autowire the Factory
Now, you can autowire the ThingFactory
in the class where you need the Thing
bean with the runtime argument(s). Spring will inject the ThingFactory
instance, and you can use it to create the Thing
bean.
@Autowired
private ThingFactory thingFactory;
public void onRequest(Request request) {
String name = request.getParameter("name");
Thing thing = thingFactory.createThing(name);
// ...
}
Why Use a Custom Factory Method Bean?
You might be wondering why you need to go through the trouble of creating a custom factory method bean instead of using the straightforward approach that XML config offers. Here are a few reasons:
Flexibility: Custom factory method beans allow you to have more control over the creation of your beans and handle runtime arguments dynamically.
Testability: By separating the creation logic into a factory method bean, you can easily write tests for the factory implementation, ensuring that it functions correctly.
Reduced Coupling: Using a factory method bean helps decouple the bean creation logic from the business logic, making your code more maintainable and easier to understand.
Conclusion and Call-to-Action
Congratulations! 👏 You've learned how to create a prototype-scoped @Bean
with runtime arguments in Spring Java Config. By using a custom factory method bean, you can achieve the same flexibility and control that XML config offers. So, say goodbye to unnecessary factories and embrace the Spring way! 🌼
If you found this blog post helpful, don't hesitate to share it with your friends and colleagues who might benefit from it. And if you have any questions or additional insights, feel free to leave a comment below. Happy coding! 💻😄