Spring CrudRepository findByInventoryIds(List<Long> inventoryIdList) - equivalent to IN clause
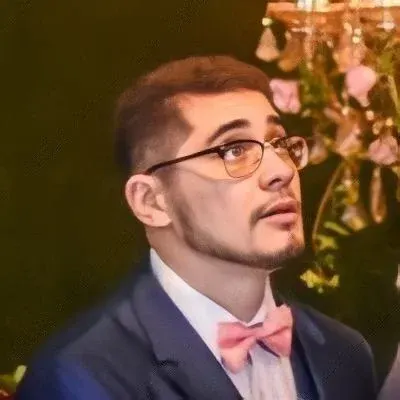
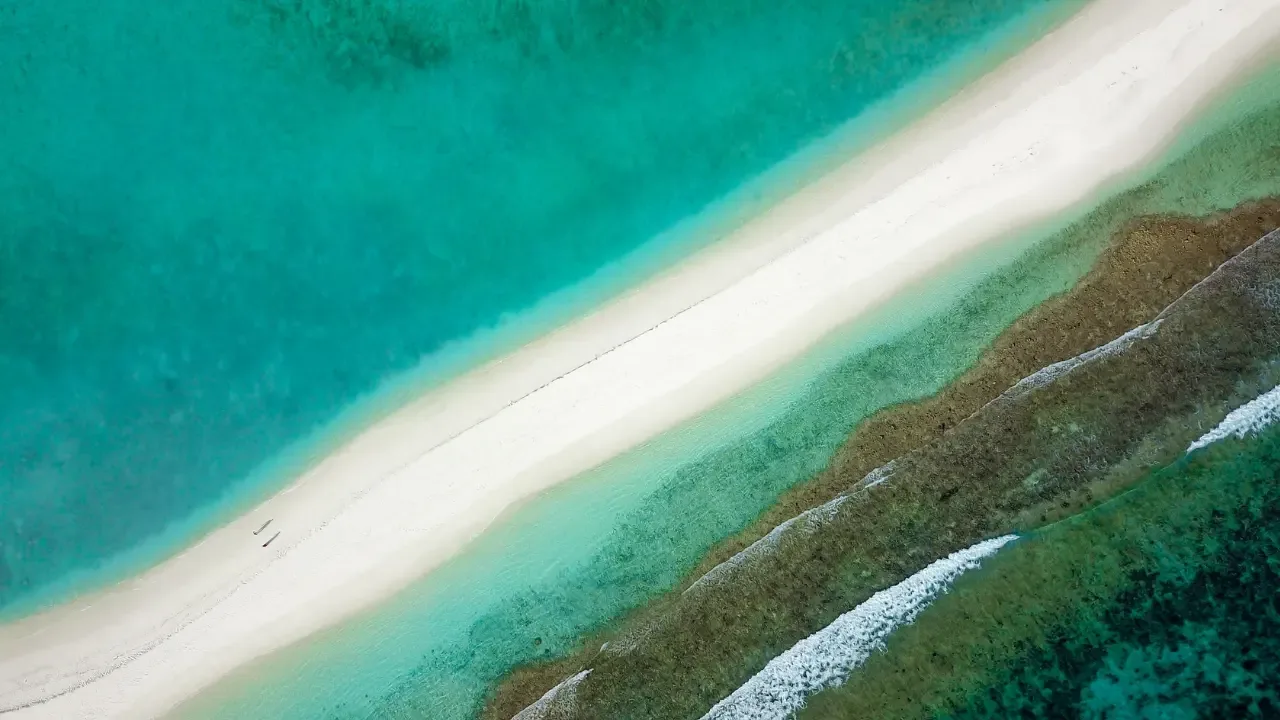
🔄 Spring CrudRepository findByInventoryIds(List<Long> inventoryIdList) - equivalent to IN clause
Do you find yourself in a situation where you need to perform a query in Spring CrudRepository based on a list of inventory ids? 🤔 You might be wondering if there's an equivalent to the "IN clause" for a field in Spring CrudRepository. Let's dive in and find out!
🔎 The Problem
Let's say you have a method in your Spring CrudRepository called findByInventoryIds(List\<Long> inventoryIdList)
. You want to retrieve all the entities that match the list of inventory ids provided. However, you're not quite sure if this is supported by Spring CrudRepository out of the box.
🆘 The Solution Unfortunately, Spring CrudRepository doesn't have a built-in equivalent to the "IN clause" for fields. But fear not, there are elegant options available to address this issue! Let's explore a couple of alternatives:
Using Query Methods with Iterables
One option is to use a Query Method with an Iterable of inventory ids instead of a list. This way, you can pass a collection of inventory ids to the method and retrieve the desired entities. Here's an example:
@Repository
public interface YourRepository extends CrudRepository<YourEntity, Long> {
List<YourEntity> findByInventoryIdIn(Iterable<Long> inventoryIdList);
}
Now you can call this method with a list of inventory ids:
List<Long> inventoryIds = List.of(1L, 2L, 3L);
List<YourEntity> entities = yourRepository.findByInventoryIdIn(inventoryIds);
Using Native Queries
If using Query Methods doesn't suit your needs, another option is to define a native query. This allows you to write the query manually, including the "IN clause" for the field. Here's an example:
@Repository
public interface YourRepository extends CrudRepository<YourEntity, Long> {
@Query(value = "SELECT * FROM your_table WHERE inventory_id IN (:inventoryIds)", nativeQuery = true)
List<YourEntity> findByInventoryIds(@Param("inventoryIds") List<Long> inventoryIdList);
}
In this case, you would call the method the same way as before:
List<Long> inventoryIds = List.of(1L, 2L, 3L);
List<YourEntity> entities = yourRepository.findByInventoryIds(inventoryIds);
🚀 Take Action Now that you have a clear understanding of how to handle queries based on a list of inventory ids in Spring CrudRepository, it's time to put this knowledge into practice! 🙌 Experiment with both options and see which one works best for your specific use case.
Tell us about your experience in the comments below! Have you encountered any other challenges with Spring CrudRepository? We're here to help! 😊
Don't forget to share this article with your fellow developers who might find it helpful. Together, we can make coding easier and more enjoyable! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
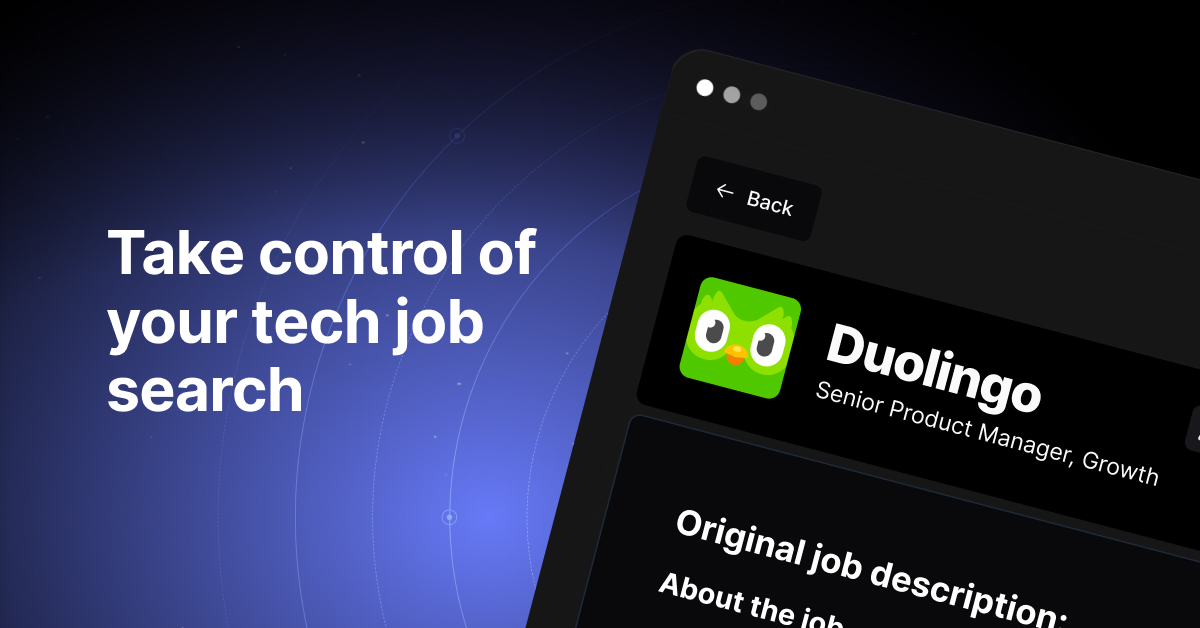