Spring: @Component versus @Bean
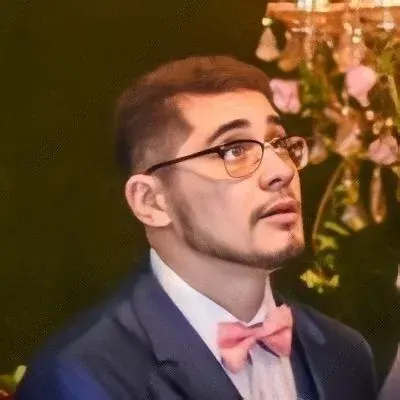
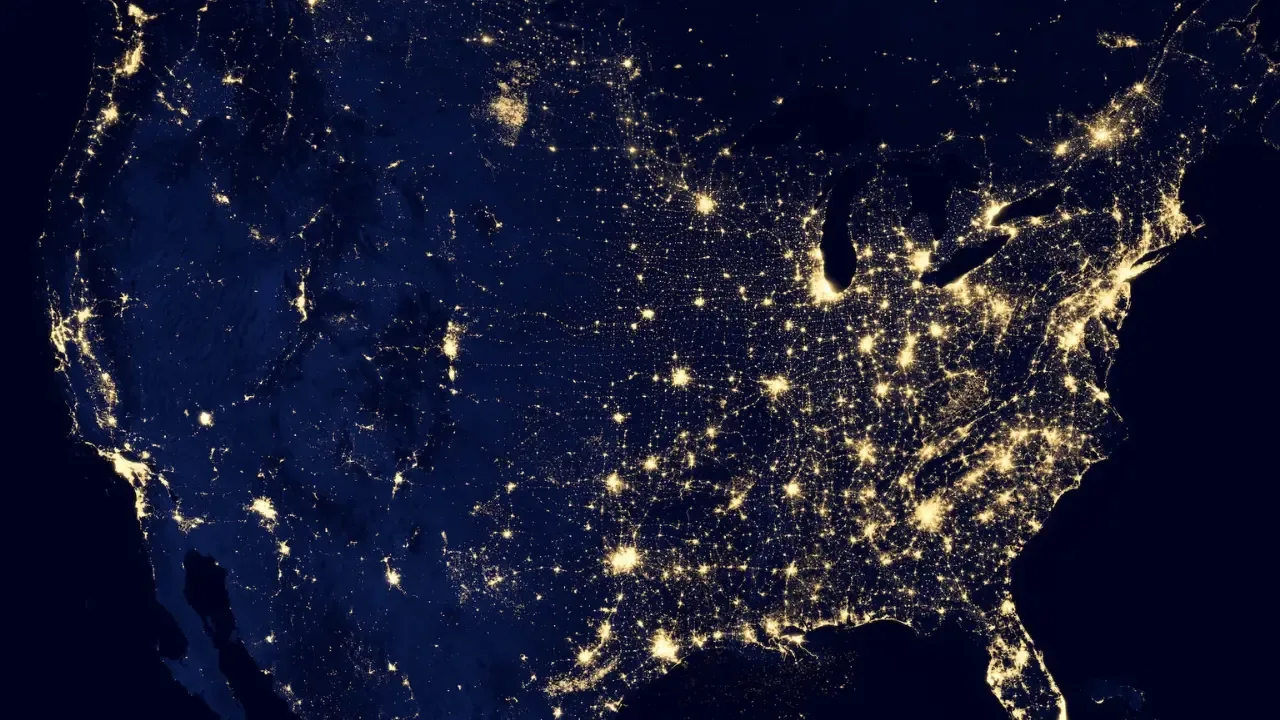
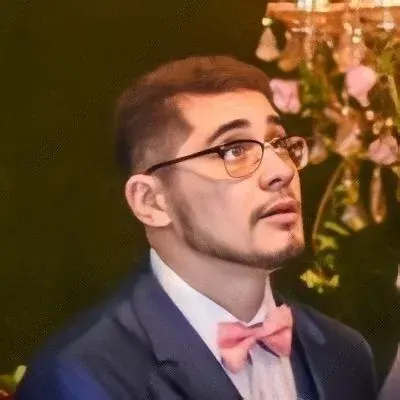
Spring: @Component vs @Bean: Which to Use and When? 😕
Spring framework provides two popular annotations - @Component and @Bean - to define beans. But when should you use one over the other? And why do we even have two options? 🤔
In this blog post, I'll break down the differences between @Component
and @Bean
and help you understand their common issues, easy solutions, and when to use each annotation. Let's dive right in! 💪
Understanding @Component and @Bean 🧐
@Component: Introduced in Spring 2.5, @Component
is used as a generic annotation to mark a class as a Spring-managed component. It allows Spring to automatically detect and create beans by using classpath scanning. This eliminates the need for XML bean definitions and provides a convenient way to manage beans in your application.
@Bean: On the other hand, @Bean
was introduced in Spring 3.0 and is used in combination with @Configuration
. With @Bean
, you can fully eliminate the need for an XML file and use Java configuration instead. It allows you to explicitly define beans and their dependencies in a configuration class.
The Final Goal: Creating Beans 🌱
Now, let's address the main question: Could we have just used @Component
instead of introducing @Bean
? 🤔 After all, the ultimate goal in both cases is to create beans.
The answer lies in the flexibility and explicitness of @Bean
. While @Component
is great for simple cases where you don't need fine-grained control over bean creation, @Bean
provides more advanced features that come in handy for complex scenarios. With @Bean
, you can manually instantiate beans, specify dependency injection, and even customize their lifecycle.
Common Issues and Easy Solutions 🔧
1. Creating Non-Spring Objects
A common issue arises when creating non-Spring managed objects as beans. For example, if you need to create a bean from a third-party library class, you can't directly annotate it with @Component
. Instead, you can use @Bean
in a configuration class to define and manage the bean.
@Configuration
public class MyConfig {
@Bean
public ThirdPartyLibraryClass thirdPartyLibraryBean() {
return new ThirdPartyLibraryClass();
}
}
2. Fine-grained Control over Dependency Injection
In some cases, you might need fine-grained control over dependency injection, such as injecting different implementations based on conditions. With @Bean
, you can achieve this by using conditional logic while declaring the bean method.
@Configuration
public class MyConfig {
@Bean
@ConditionalOnProperty(name = "my.feature.enabled", havingValue = "true")
public MyInterface myBean() {
return new MyImplementation1();
}
@Bean
@ConditionalOnProperty(name = "my.feature.enabled", havingValue = "false")
public MyInterface myBean() {
return new MyImplementation2();
}
}
3. External Configuration
Another advantage of @Bean
is its flexibility to utilize external configuration values. When defining a bean method, you can inject external properties or values directly into the method parameters.
@Configuration
public class MyConfig {
@Value("${my.property.name}")
private String myPropertyValue;
@Bean
public MyBean myBean() {
return new MyBean(myPropertyValue);
}
}
So, When Should You Use Each Annotation? 🤔
In a nutshell, use @Component
when you want simplicity and auto-detection of beans, and your requirements are straightforward. It's an excellent choice for most use cases.
However, if you need more control, want to create non-Spring objects as beans, or require conditional configurations, opt for @Bean
in combination with @Configuration
. It gives you the power to define and manage beans explicitly.
Your Turn! ✍️
I hope this blog post cleared up the confusion around @Component
and @Bean
annotations. Now it's your turn to decide which annotation suits your needs best! Share your thoughts, experiences, and any further questions you have in the comments below. Let's start a Spring-tastic conversation! 💬💡
🔍 Explore more about Spring annotations here.
📣 Don't forget to share this post with your fellow developers who are navigating the Spring world. Knowledge is best when shared! 🚀