Spring Cache @Cacheable - not working while calling from another method of the same bean
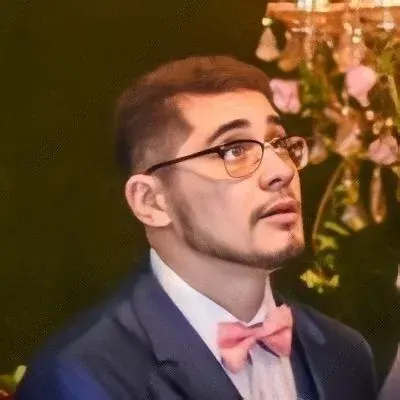
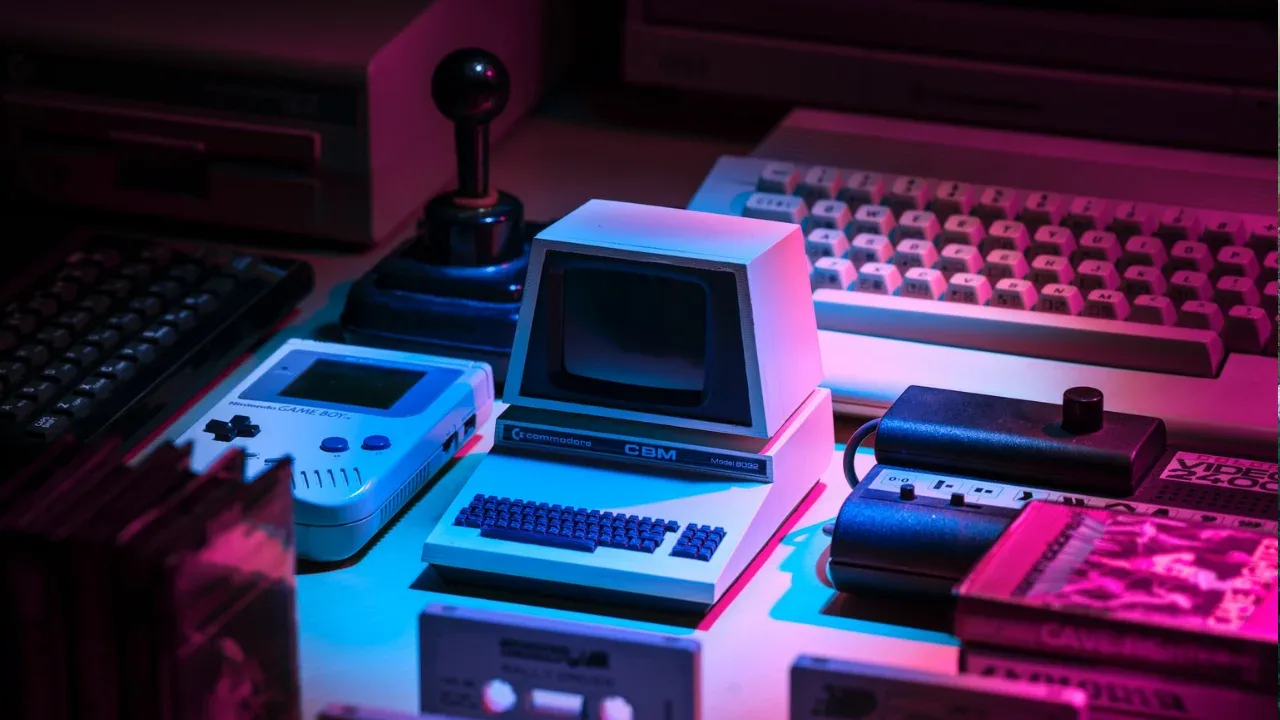
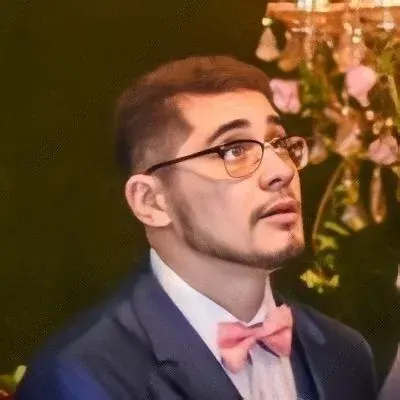
📝 Spring Cache @Cacheable - Not Working While Calling from Another Method of the Same Bean
Have you ever faced the issue where Spring cache doesn't work when calling a cached method from another method within the same bean? Well, you're not alone! This problem is quite common and can be quite frustrating. But fear not, for I am here to guide you through this issue and provide easy solutions. Let's dive in! 💪
To better understand the problem, let's take a look at an example:
@Configuration
public class AppConfig {
@Bean
public CacheManager cacheManager() {
// Configure your preferred cache manager here
}
}
@Service
public class MyService {
@Cacheable("myCache")
public Object cachedMethod() {
// Some expensive computation or external API call
}
public void anotherMethod() {
// Call to the cached method within the same bean
Object result = cachedMethod();
}
}
In the code snippet above, we have a MyService
bean with a cached method called cachedMethod()
. However, when we call cachedMethod()
from another method within the same bean, like anotherMethod()
, the cache is not being used. 😞
So, what's causing this issue? Well, the problem lies in the way Spring cache proxies work. When you call a method from within the same bean, Spring bypasses the proxy and directly invokes the target method. As a result, the caching aspect is not applied, and the cache is not utilized.
🛠️ Solution: Self-Invocation
Thankfully, there's a simple solution to this problem. We can utilize self-invocation to ensure that the proxy is invoked, and the cache is utilized. Here's how you can modify your code:
@Service
public class MyService {
@Autowired
private MyService self;
@Cacheable("myCache")
public Object cachedMethod() {
// Some expensive computation or external API call
}
public void anotherMethod() {
// Call to the cached method within the same bean using self-invocation
Object result = self.cachedMethod();
}
}
By autowiring the bean itself (MyService self
), we obtain a reference to the proxy rather than the actual bean. Therefore, when we invoke self.cachedMethod()
, Spring applies the caching aspect, and the cache is utilized successfully. 🎉
🎯 Call-To-Action: Share Your Experience
Have you faced this Spring cache issue before? How did you solve it? Share your experience and solutions in the comments section below! Let's help each other overcome these challenges and make our Spring applications cache-efficient. Don't forget to share this blog post with your fellow developers who might be facing the same issue. Happy caching! 🚀