Spring Boot REST service exception handling
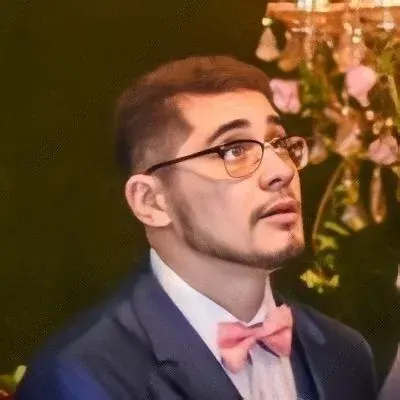
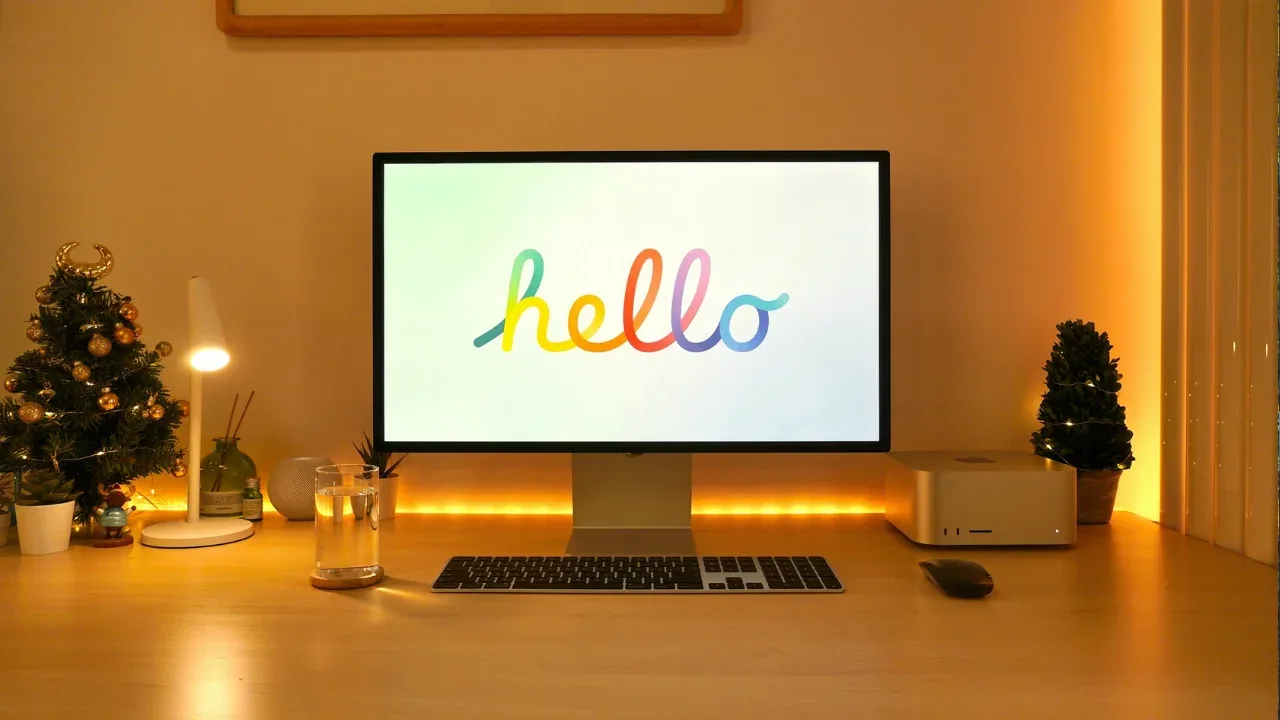
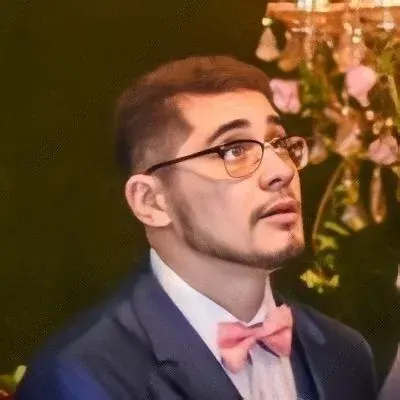
Handling Exceptions in Spring Boot REST Services
š Welcome to our blog post, where we'll dive into handling exceptions in Spring Boot REST services. If you're struggling with the default /error mapping in Spring Boot and want to return meaningful error responses to your clients, you're in the right place. Let's get started!
Understanding the Default Behavior
ā”ļø By default, Spring Boot sets up a global error page at /error that handles all exceptions and redirects to a page or URL. However, this may not be ideal for REST services, where we want to return error responses directly to the client.
š¤ You may find the default behavior of Spring Boot confusing, especially if you're coming from a background of writing REST applications with Node.js. In REST services, it is common practice to return the error response directly in the HTTP response, instead of redirecting the client.
Setting Up a Global Error Handler
š To achieve our goal of returning error responses without any redirects, we need to define a custom global error handler. Thankfully, Spring Boot provides two main approaches to accomplish this: implementing the ErrorController interface or using @ControllerAdvice.
š ErrorController Implementation: You can create your own class that implements the ErrorController interface. This approach allows you to handle any exception thrown by your request mapping methods or auto-generated by Spring (e.g., 404 if no handler method is found). More details on the ErrorController interface can be found in the Spring Boot documentation.
š @ControllerAdvice: Another approach is to use the @ControllerAdvice annotation. This annotation allows you to define an advice class that will handle exceptions globally across all your controllers. By using @ExceptionHandler methods in your advice class, you can catch and handle specific exception types, or even handle all exceptions by catching Throwable. Check out the Spring Framework documentation for more information on @ControllerAdvice.
Returning Custom Error Responses
š® Now, let's focus on returning custom-formatted error responses for your REST service. Our goal is to log the error to NoSQL with a unique UUID and return the appropriate HTTP error code along with the UUID in the JSON response body.
š” With either approach mentioned above, you have full control over the error response. You can leverage Spring's powerful ResponseEntity class to build and return the desired response. For example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Throwable.class)
public ResponseEntity<ErrorResponse> handleException(Throwable ex) {
// Log the error to NoSQL with UUID
String uuid = logErrorToNoSQL(ex);
// Build the error response
ErrorResponse error = new ErrorResponse(HttpStatus.INTERNAL_SERVER_ERROR, uuid);
// Return the error response with the appropriate HTTP status code
return new ResponseEntity<>(error, error.getStatus());
}
}
š„ In the above example, we handle any Throwable (all exceptions) and create an ErrorResponse object containing the UUID and the corresponding HTTP status code. Finally, we return the error response using ResponseEntity.
Keep Exploring!
š Congrats! Now you know how to handle exceptions in Spring Boot REST services, log errors, and return custom error responses. Remember, you can further enhance this approach by catching and handling specific exception types or even creating more specific error response classes.
š We hope you found this blog post helpful in tackling your exception handling issues. If you have any additional questions or suggestions, let us know in the comments below. Happy coding!