Spring Boot configure and use two data sources
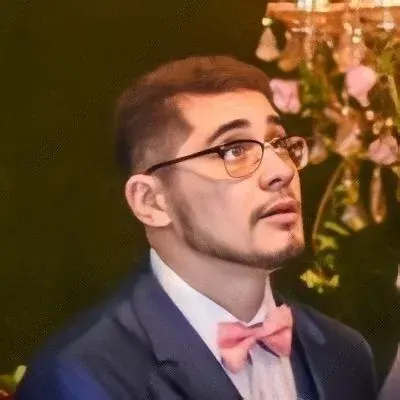
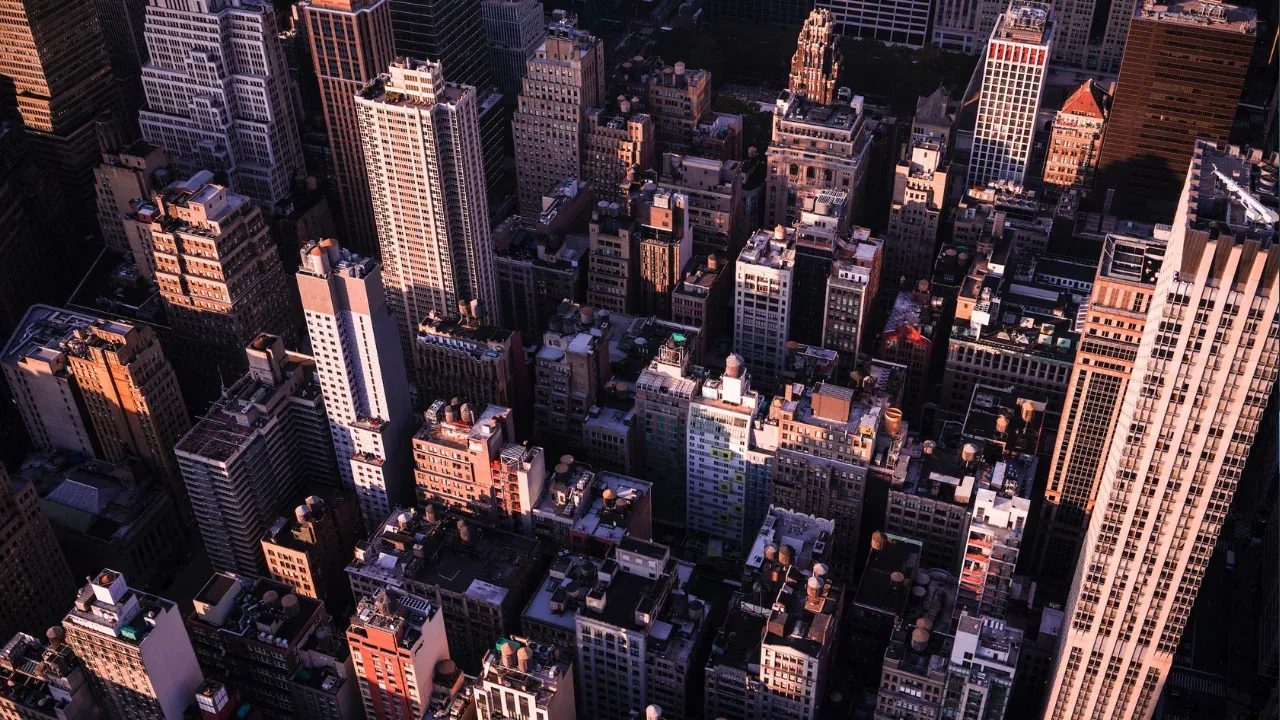
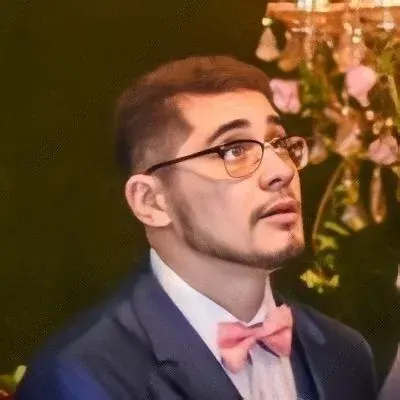
š Blog Post: Spring Boot - Configure and Use Two Data Sources
š Hey there tech enthusiasts! Today, let's dive into a common question: "How can I configure and use two data sources in Spring Boot?" If you're facing this challenge, worry not! We've got you covered with easy solutions. š
š Understanding the Problem
To start, let's take a look at the context of this question. The user wants to configure an additional data source alongside an existing one. They've provided the configuration for the first data source in the application.properties
file and the skeleton of the Application class. The challenge lies in modifying the application.properties
file to include the second data source and correctly autowiring it to be used by a different repository. Let's break it down step by step. šŖ
š” Step 1: Modifying application.properties
To add a second data source, open the application.properties
file and add the following properties:
# First data source
spring.datasource.url = [url]
spring.datasource.username = [username]
spring.datasource.password = [password]
spring.datasource.driverClassName = oracle.jdbc.OracleDriver
# Second data source
spring.second-datasource.url = [url]
spring.second-datasource.username = [username]
spring.second-datasource.password = [password]
spring.second-datasource.driverClassName = org.postgresql.Driver
Replace [url]
, [username]
, and [password]
with the details of your second data source. Notice that we've added a prefix second-datasource
to differentiate it from the first data source.
⨠Pro Tip: You can add additional properties specific to the second data source, just prefix them with spring.second-datasource
.
š Step 2: Modifying the Application Class Next, we need to modify the Application class to configure the second data source. Add the following code:
@SpringBootApplication
public class SampleApplication {
@Autowired
private Environment env;
@Bean(name = "secondDataSource")
public DataSource secondDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setUrl(env.getProperty("spring.second-datasource.url"));
dataSource.setUsername(env.getProperty("spring.second-datasource.username"));
dataSource.setPassword(env.getProperty("spring.second-datasource.password"));
dataSource.setDriverClassName(env.getProperty("spring.second-datasource.driverClassName"));
return dataSource;
}
// Add other beans, configurations, and necessary code
public static void main(String[] args) {
SpringApplication.run(SampleApplication.class, args);
}
}
The new secondDataSource()
method uses the properties we defined earlier to configure the second data source.
š Congratulations! You've successfully configured and added a second data source in Spring Boot. Now, you can autowire it to be used by a different repository.
š Wrapping Up We hope this step-by-step guide helps you in configuring and using two data sources in your Spring Boot application. Remember, you can extend this approach to add even more data sources if needed. Play around with the properties and customize them according to your requirements. š ļø
š¢ Share your Experience! We would love to hear about your experience with configuring multiple data sources in Spring Boot. Did you face any challenges? Do you have any additional tips or tricks? Share them in the comments section below and join the discussion! Let's learn and grow together. šš£ļø
š” Stay Tuned! Make sure to subscribe to our blog for more exciting tech tips and tricks. Stay up to date with the latest trends and challenges in the tech world. Until next time, happy coding! š»š
(Note: This blog post assumes a basic understanding of Spring Boot and database configuration. If you're new to Spring Boot, we recommend brushing up on the basics before diving into multiple data source configuration.)