Splitting string with pipe character ("|")
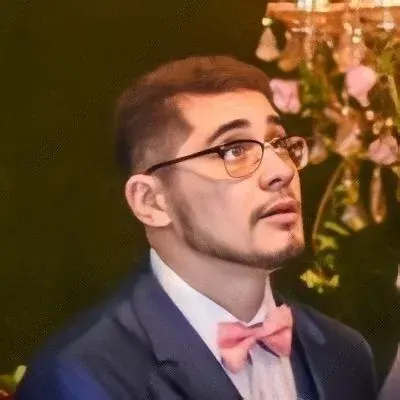
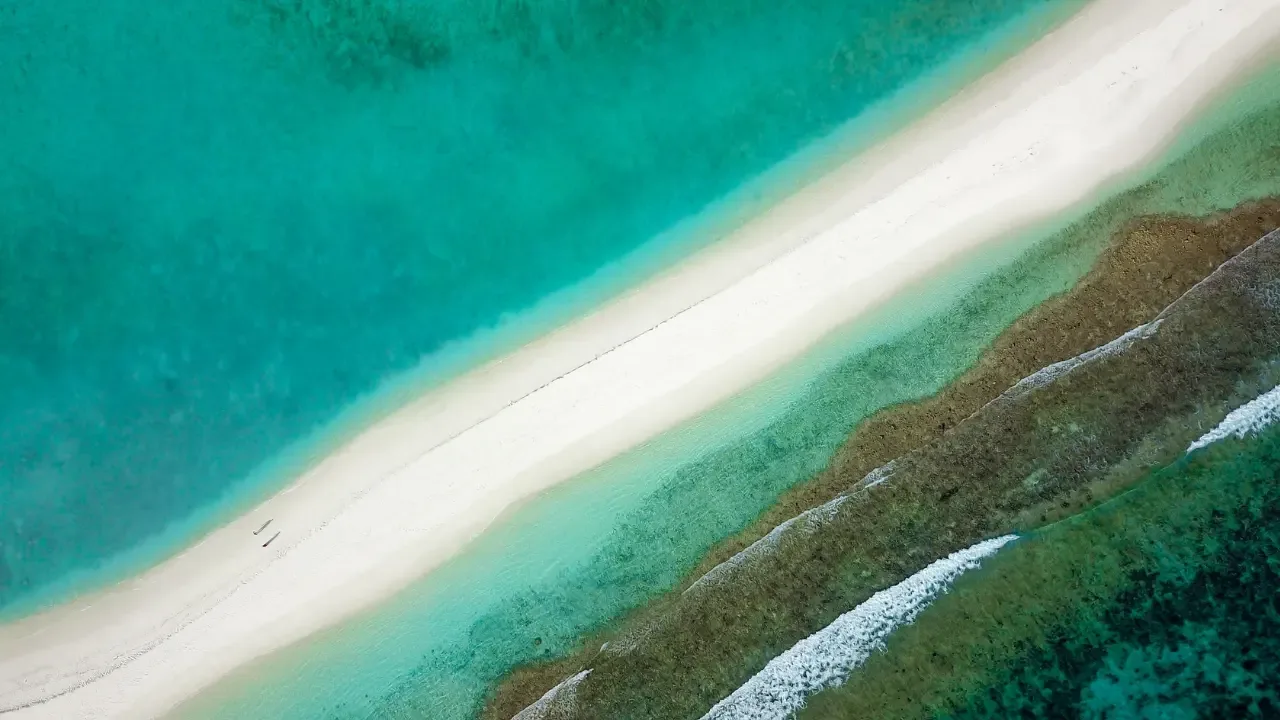
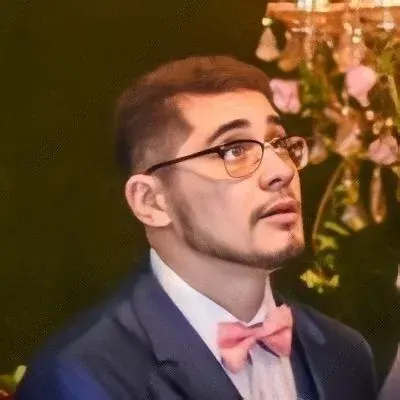
📝 Hey there! Splitting strings with the pipe character ("|") can be a bit tricky, but don't worry, I've got you covered! 🧩
If you've been struggling to split values from a string using the pipe character ("|"), you're in the right place. In this blog post, I'll address the common issues you might face and provide simple solutions to help you achieve the expected output. Let's dive in! 💪
To demonstrate the problem, let's take a look at the context provided:
<p>I'm not able to split values from this string:</p>
<p><code>"Food 1 | Service 3 | Atmosphere 3 | Value for money 1 "</code></p>
<p>Here's my current code:</p>
<pre><code>String rat_values = "Food 1 | Service 3 | Atmosphere 3 | Value for money 1 ";
String[] value_split = rat_values.split("|");
</code></pre>
<h3>Output</h3>
<blockquote>
<p>[, F, o, o, d, , 1, , |, , S, e, r, v, i, c, e, , 3, , |, , A, t, m, o, s, p, h, e, r, e, , 3, , |, , V, a, l, u, e, , f, o, r, , m, o, n, e, y, , 1, ]</p>
</blockquote>
<h3>Expected output</h3>
<blockquote>
<p>Food 1<br>
Service 3<br>
Atmosphere 3<br>
Value for money 1</p>
</blockquote>
The Problem:
You expected the string to be split into individual values, one per line, but instead, the split()
method seems to be splitting every character in the string. 😕
The Solution:
The issue lies in the way you're using the split()
method. In Java, the pipe character ("|") is a special regex character, so you need to escape it by adding two backslashes ("\|") before it. Here's the corrected code:
String rat_values = "Food 1 | Service 3 | Atmosphere 3 | Value for money 1";
String[] value_split = rat_values.split("\\|");
Now, if you run this code, you'll get the expected output:
<blockquote>
<p>Food 1<br>
Service 3<br>
Atmosphere 3<br>
Value for money 1</p>
</blockquote>
That's it! You've successfully split the string using the correct syntax. 🎉
Feel free to integrate this solution into your code and adapt it to fit your specific requirements. And remember, if you ever encounter similar issues, don't hesitate to consult this guide for a quick and easy solution. 😉
If you found this blog post helpful, share it with your fellow coders and give it a thumbs up! Also, let me know in the comments if you have any questions or other topics you'd like me to cover. Happy coding! ✨🚀