Split Java String by New Line
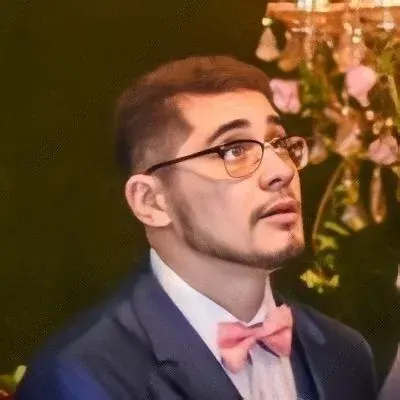
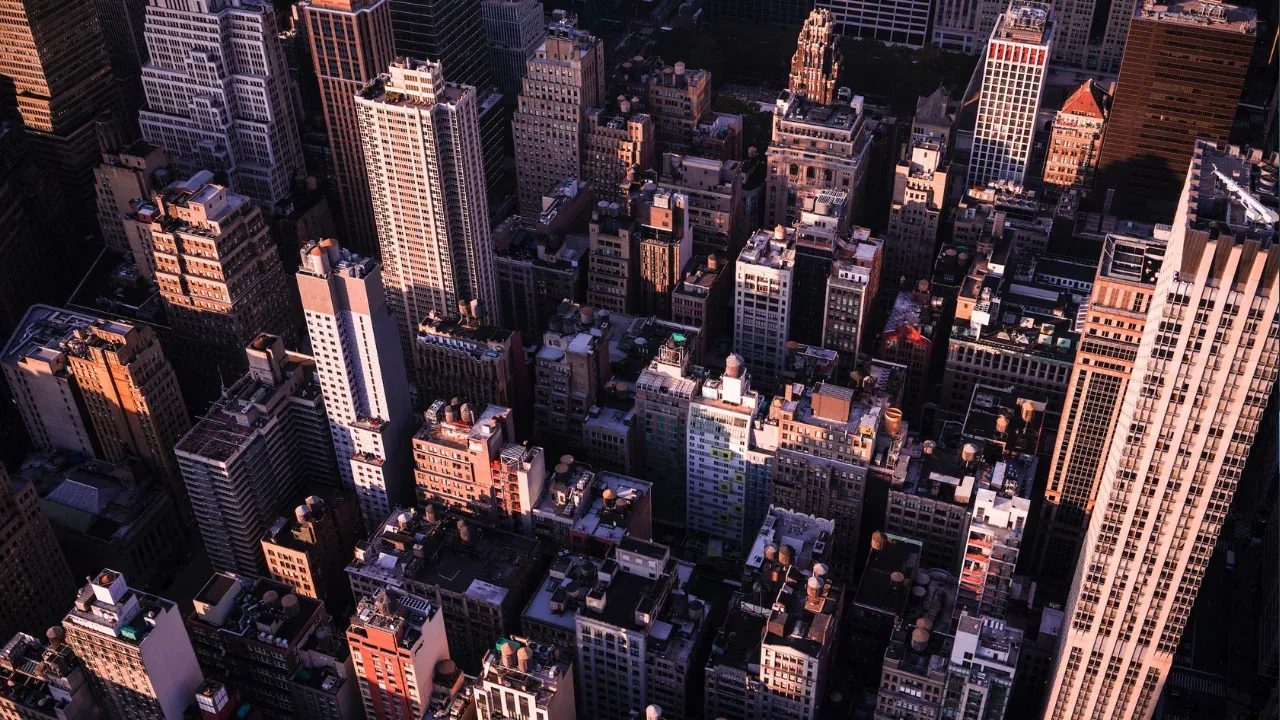
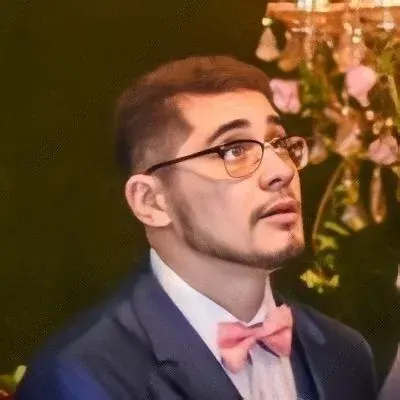
Splitting Java String by New Line: Easy Solutions for Common Issues
Are you struggling to split a Java String by a new line? 😩 Don't worry, you're not alone! Many Java developers face this challenge when working with text manipulation. In this blog post, we will explore common issues and provide easy solutions to help you conquer this problem. Let's dive in! 🚀
The Problem
One common mistake developers make when trying to split a Java String by a new line is using the incorrect regex or escape characters. In the provided example, the author tried using a regex pattern "\n"
to split the String, but it didn't work as expected. 😕
The Solution
To split a Java String by a new line, you need to use the correct regex pattern. In Java, newline characters can vary depending on the operating system. The three common newline characters are:
\n
: Unix and Linux newline character.\r
: Mac OS classic newline character.\r\n
: Windows newline character.
To handle all these cases, you can use the following regex pattern: "\r?\n|\r"
. Let's update the code snippet accordingly:
public void insertUpdate(DocumentEvent e) {
String split[], docStr = null;
Document textAreaDoc = (Document)e.getDocument();
try {
docStr = textAreaDoc.getText(textAreaDoc.getStartPosition().getOffset(), textAreaDoc.getEndPosition().getOffset());
} catch (BadLocationException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
split = docStr.split("\r?\n|\r");
}
With this regex pattern, you can handle different newline characters and split the String accordingly. 🙌
Bonus Tip: Trim Leading and Trailing Spaces
Sometimes, when splitting a String by a new line, you may encounter leading or trailing spaces in each split section. If you want to remove these spaces, you can simply add .trim()
to each split String in the resulting array:
split = docStr.split("\r?\n|\r");
for (int i = 0; i < split.length; i++) {
split[i] = split[i].trim();
}
Conclusion
Splitting a Java String by a new line can be a tricky task, but with the right understanding and the proper regex pattern, it becomes a breeze. 🌬️ Remember to use "\r?\n|\r"
as your regex pattern to handle different newline characters, and don't forget to trim any leading or trailing spaces if necessary. Now go ahead and conquer those text manipulation challenges! 💪
Have you encountered any other Java String manipulation issues? Share your experience in the comments below and let's discuss! 👇