Sort a Map<Key, Value> by values
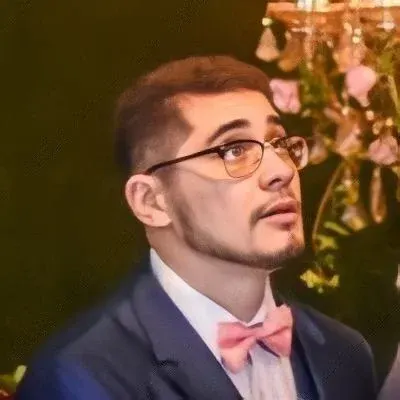
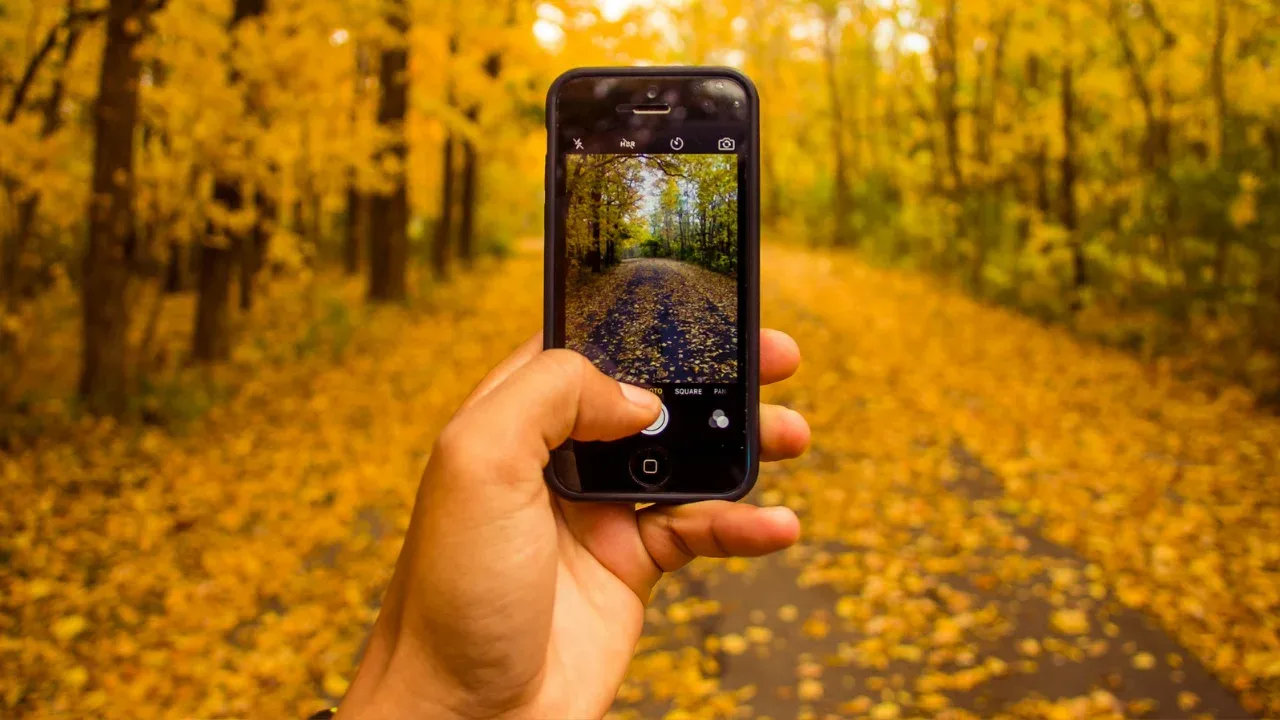
How to Sort a Map by Values: An Easy Solution πΊοΈπ€π
Are you struggling to sort a Map<Key, Value>
by its values? You're not alone! Sorting a map based on its values can be a tricky task, especially when dealing with non-unique values. But don't worry, I've got you covered! In this guide, I'll walk you through an easy solution that will save you time and effort.
The Problem π©
Let's first understand the problem at hand. You have a Map
object that pairs keys with corresponding values. However, you need to sort the map based on the values, not the keys. Unfortunately, the Map
interface doesn't provide a built-in sorting mechanism for this specific scenario.
Usually, people resort to converting the keySet
into an array and sorting it using an array sort with a custom comparator. This can quickly become convoluted and difficult to maintain, especially as the complexity of your map grows.
The Solution π‘
Fortunately, there is an easier way to sort a Map
by its values. The trick is to make use of the java.util.stream API, introduced in Java 8. This powerful API allows you to streamline your code and perform complex operations on collections, such as sorting a map by values.
Here's how you can achieve this:
import java.util.*;
import java.util.stream.*;
public class MapSortingExample {
public static void main(String[] args) {
Map<String, Integer> map = new HashMap<>();
map.put("Apple", 6);
map.put("Orange", 3);
map.put("Banana", 9);
Map<String, Integer> sortedMap = map.entrySet()
.stream()
.sorted(Map.Entry.comparingByValue())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue,
(oldValue, newValue) -> oldValue, LinkedHashMap::new));
System.out.println(sortedMap);
}
}
Running this code will produce the following output:
{Orange=3, Apple=6, Banana=9}
Voila! The map is now sorted in ascending order based on its values. π
Explanation π§
Let's break down the solution step by step:
We start by creating a stream of map entries using
map.entrySet().stream()
. This allows us to operate on each key-value pair individually.Next, we use the
sorted()
method, which takes aComparator
as an argument. In our case, we useMap.Entry.comparingByValue()
as the comparator to sort the entries by their values.The
collect()
method is then used to gather the sorted entries into a new map. We specify the key and value usingMap.Entry::getKey
andMap.Entry::getValue
methods, respectively.To maintain the order of the sorted entries, we create a
LinkedHashMap
by passingLinkedHashMap::new
as the fourth argument to thecollect()
method.
And that's it! You now have a sorted map based on its values without the need for complex conversions or custom comparators.
Take it to the Next Level π
Now that you've learned a simple solution to sorting a map by values, why not apply it to your own project? Experiment with different types of maps, custom comparators, or even reverse sorting order.
Don't hesitate to share your experiences, ask questions, or suggest improvements in the comments below. Let's build a community of tech enthusiasts who help each other grow! π±
So go ahead, give it a try, and unlock the power of sorted maps! πͺ
Happy coding! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
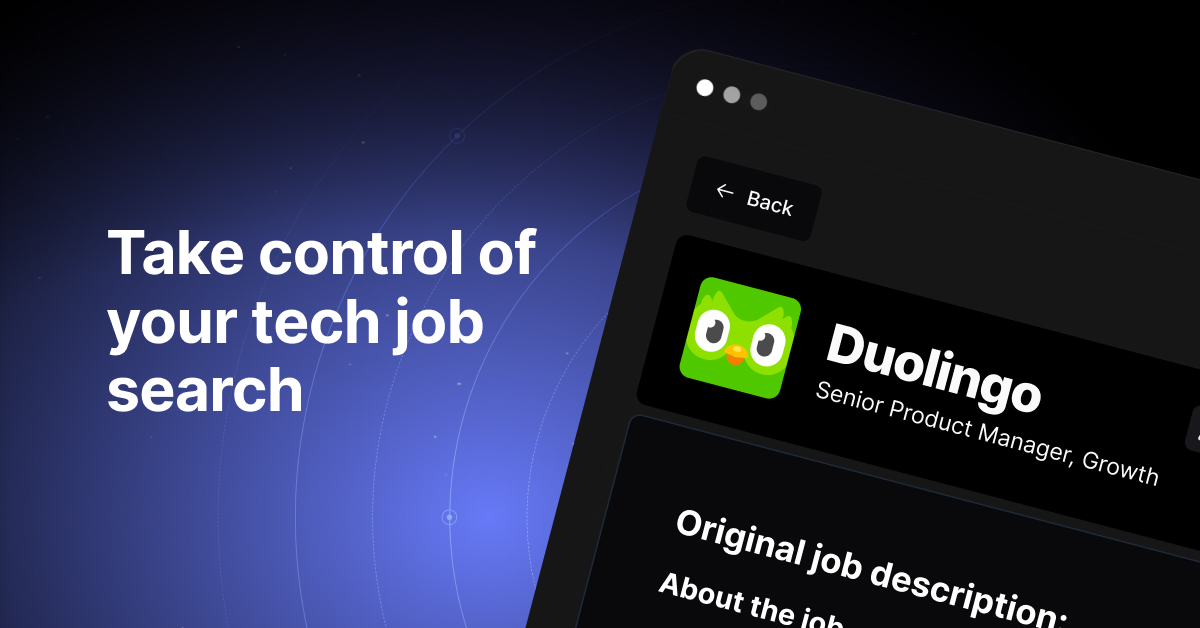