Showing a Spring transaction in log
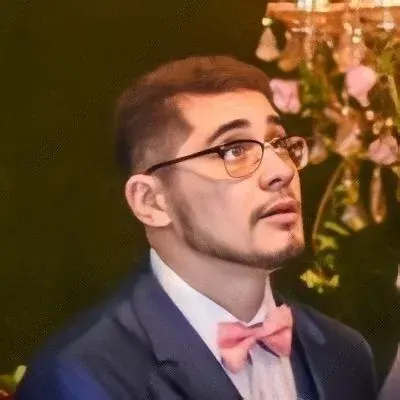
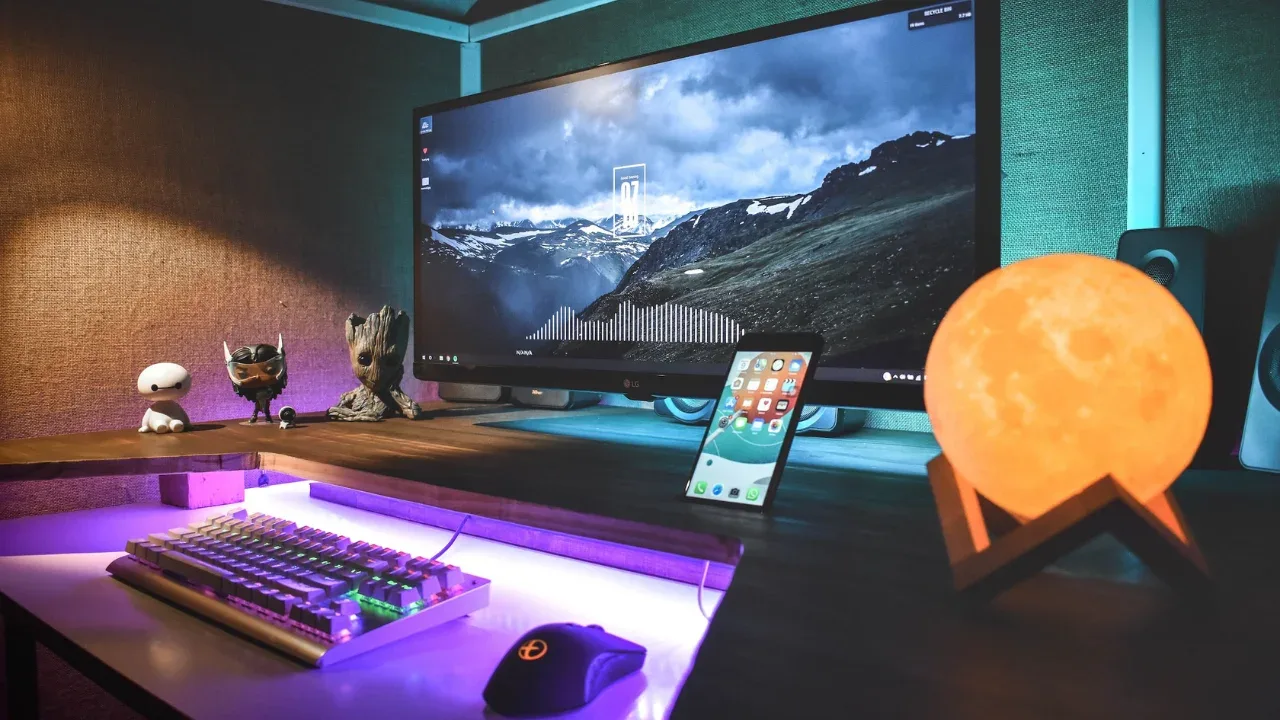
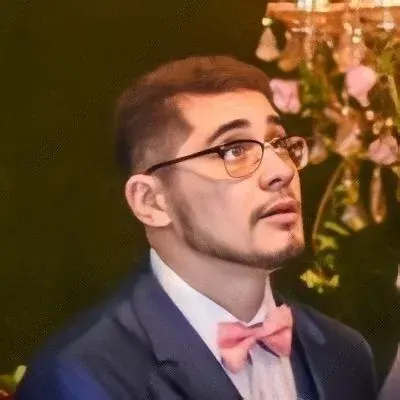
"Logging Spring Transactions: Understanding the Magic Happening Behind the Scenes" š«š°
š£ Hey there, fellow tech enthusiasts! Are you stuck trying to figure out how to log transactions in your Spring application? Don't worry, we've got you covered! In this blog post, we'll address the common issues developers face when trying to log Spring transactions and provide you with easy solutions. š§šŖ
š Understanding the Need:
As a diligent developer, it's vital to ensure that your transactions are properly configured in Spring. Wanting to log transactions is a smart move! Logging allows you to peek under the hood and see what's happening behind the scenes, confirming that you've set everything up correctly. š§š
š” The Solution:
Here are a few foolproof ways to log your Spring transactions and feel confident about your setup:
1. Configure Logging Frameworks:
By default, Spring uses the Logback framework for logging. You need to set up the appropriate log level and logging configuration to see transaction-related log messages. For example, in a Spring Boot application, you can define this in the application.properties
or application.yml
file:
logging.level.org.springframework.transaction: TRACE
This configuration will enable TRACE-level logging for all Spring transaction-related activities. šāļø
2. Enable Transaction Logging:
Spring provides AOP (Aspect-Oriented Programming) support to intercept and log transactions. With a few simple configuration steps, you can enable logging of each transaction's start, commit, and rollback operations. Here's an example of how to achieve this using annotations:
@Configuration
@EnableTransactionManagement
public class AppConfig {
@Bean
public PlatformTransactionManager transactionManager(DataSource dataSource) {
return new DataSourceTransactionManager(dataSource);
}
@Bean
public TransactionInterceptor transactionInterceptor(PlatformTransactionManager transactionManager) {
TransactionInterceptor interceptor = new TransactionInterceptor();
interceptor.setTransactionManager(transactionManager);
interceptor.setTransactionAttributes(new Properties());
Properties transactionAttributes = new Properties();
transactionAttributes.setProperty("*.txn*", "PROPAGATION_REQUIRED,-Exception");
interceptor.setTransactionAttributes(transactionAttributes);
return interceptor;
}
}
By configuring the TransactionInterceptor
bean and using wildcards in transaction attributes, you can log transactions based on methods matching specific patterns. šš
3. Leverage Logging Libraries:
To enhance your transaction logging experience, you can leverage existing logging libraries integrated with Spring, such as slf4j
or log4j
. These libraries provide advanced features like log rotation, custom log formats, and easy integration with existing logging infrastructures.
Make sure to include the necessary dependencies in your project's build file, such as slf4j-api
, slf4j-log4j12
, and log4j
.
š£ The Call-To-Action:
Now that you have the secret sauce for logging Spring transactions, it's time to unleash your logging powers and gain valuable insights into your application's transactional behavior! Remember, logging is not just for debugging! Share your success stories and engage with like-minded developers in the comments below. Let's log our way into a better understanding of our applications! š»šš¬
Happy logging, folks! Keep coding and stay awesome! šāØ