setMaxResults for Spring-Data-JPA annotation?
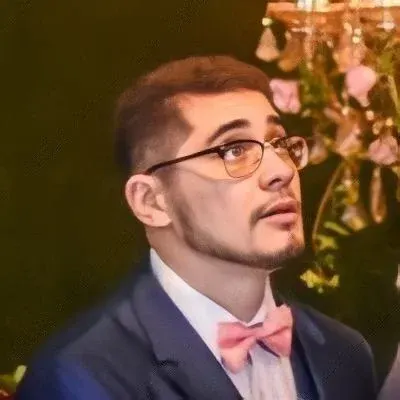
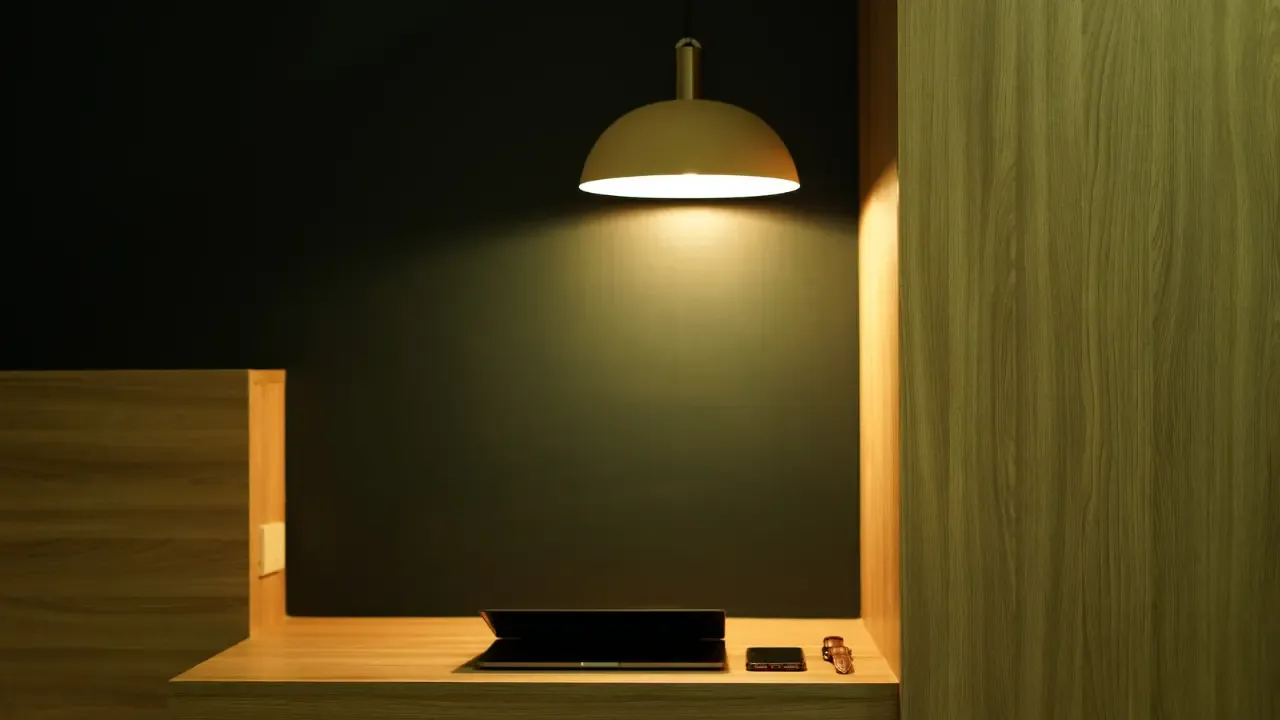
📝🔥✨💻
Title: Mastering setMaxResults for Spring-Data-JPA Annotation
Introduction
Are you feeling confused about how to use the setMaxResults()
method with annotation in Spring-Data-JPA? Don't worry, you're not alone! In this blog post, we will delve into this common issue and provide easy solutions to help you out. So, let's jump right in!
Understanding the Issue
By using the setMaxResults(n)
method, you can limit the number of results returned by a query in JPA. However, there is no direct annotation available in Spring-Data-JPA to achieve this. This limitation can be frustrating for developers like you who want to take full advantage of Spring-Data-JPA without resorting to manual implementations.
Finding a Solution
While there is no built-in support for setMaxResults()
in Spring-Data-JPA, we can still achieve the desired result by using the Pageable
interface. Here's how you can do it:
Modify the
findByOtherObj()
method in yourUserRepository
interface as follows:
@Query(value = "FROM User u WHERE u.otherObj = ?1")
public User findByOtherObj(OtherObj otherObj, Pageable pageable);
Update your repository implementation class to include the required
Pageable
parameter:
@Repository
public class UserRepositoryImpl implements UserRepository {
@PersistenceContext
private EntityManager entityManager;
@Override
public User findByOtherObj(OtherObj otherObj, Pageable pageable) {
TypedQuery<User> query = entityManager.createQuery("FROM User u WHERE u.otherObj = ?1", User.class);
query.setParameter(1, otherObj);
query.setMaxResults(pageable.getPageSize());
query.setFirstResult(pageable.getOffset());
return query.getSingleResult();
}
}
Here, we manually set the setMaxResults()
value based on the pageable
object, which provides control over the number of results to be fetched.
Why is this Feature Not Built-In?
You may be wondering why such an important feature is not directly available in Spring-Data-JPA. Well, the reality is that different databases have different ways of handling result limiting. For example, MySQL uses the LIMIT
clause, while Oracle uses ROWNUM
. To provide a consistent and portable API, Spring-Data-JPA chose not to include this feature out-of-the-box.
Engage and Share Your Thoughts
We hope this guide has helped you overcome the challenge of using setMaxResults()
in Spring-Data-JPA. Have you encountered any other issues or have suggestions for alternative solutions? We would love to hear from you! Leave a comment below or join the conversation on our social media channels.
Conclusion
In this blog post, we explored the common issue of using setMaxResults()
with annotation in Spring-Data-JPA. Although there is no direct support for this method, we demonstrated how to achieve the same result using the Pageable
interface. Don't let this limitation deter your progress - Spring-Data-JPA is a powerful tool that can be customized to fit your needs.
Remember, limitations are just opportunities waiting to be conquered! Keep exploring, keep learning, and keep coding like a pro! 💪🚀
Stay Connected
Follow us on Twitter, Facebook, and Instagram for regular updates and more helpful guides! 📲💻🌟
Have a tech question or topic you'd like us to cover? Drop us a line at techblogger@techblog.com. We'd love to hear from you!
Keep Coding! 👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
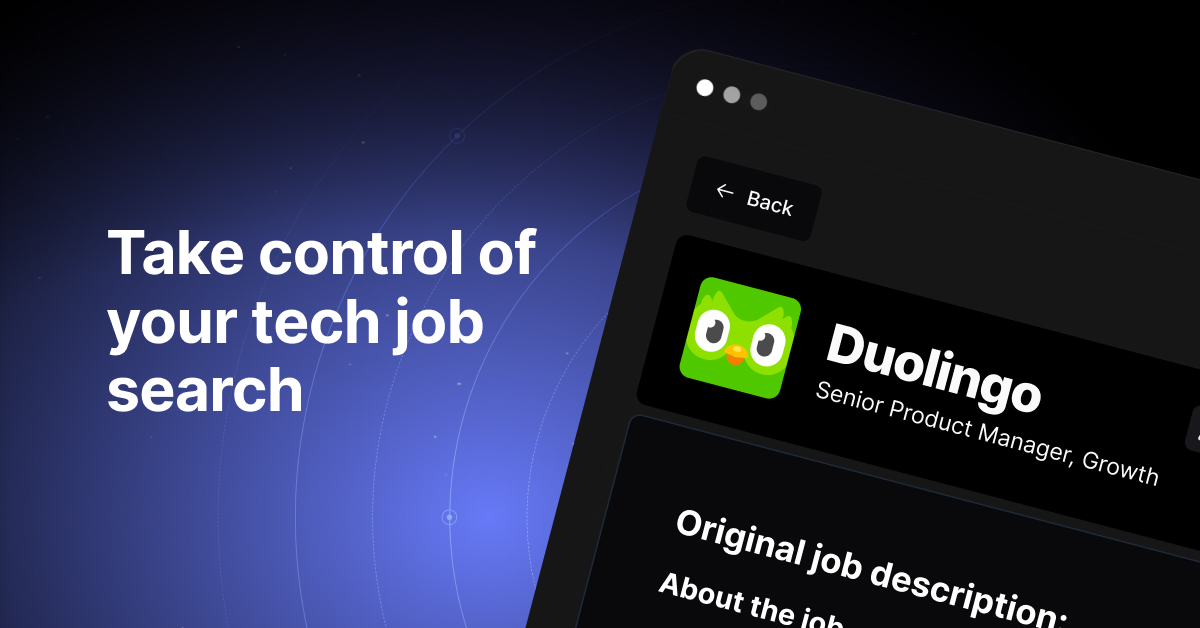