Set ImageView width and height programmatically?
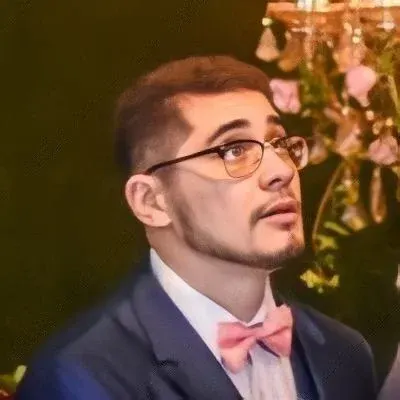
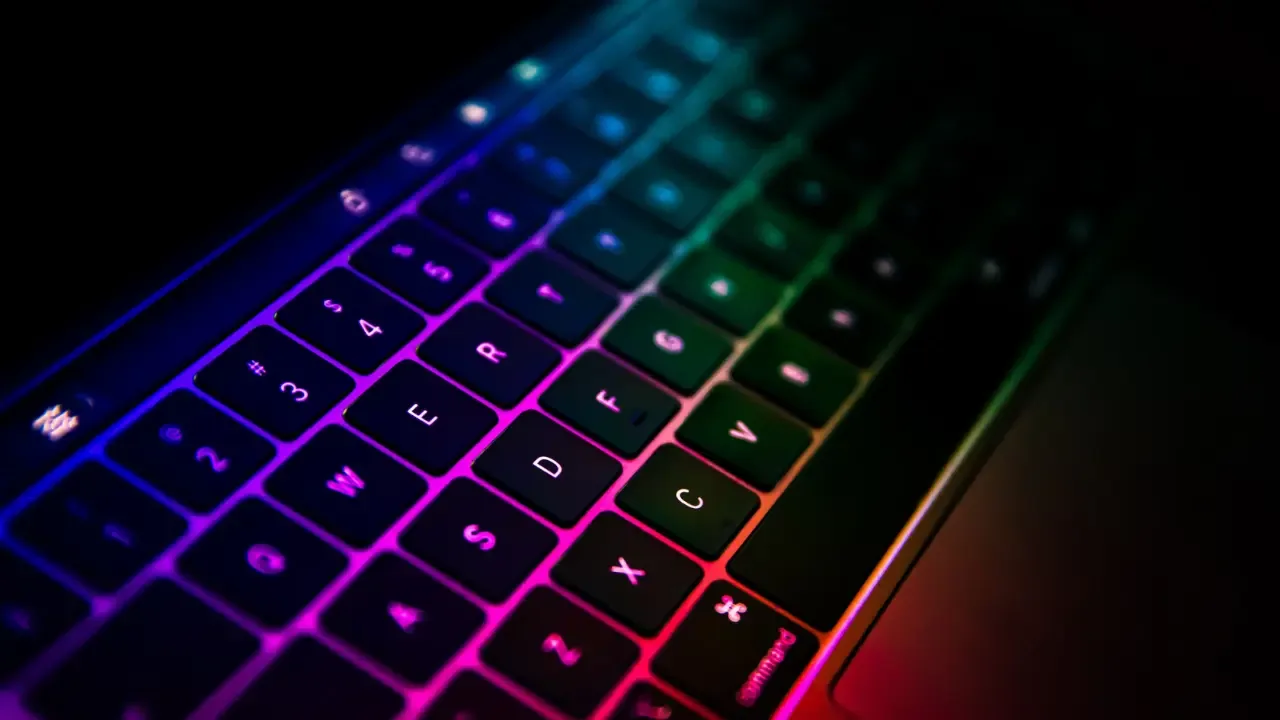
How to Set ImageView Width and Height Programmatically? 😎📏
If you've ever wondered how to set the width and height of an ImageView
programmatically in your Android app, you've come to the right place! 🎯
The Problem 😕
The default behavior of an ImageView
in Android is to wrap the content it displays. This means that by default, the width and height of the ImageView
will be determined by the size of the image it is displaying. But what if you want to set the width and height to specific values programmatically, without relying on the image size?
The Solution 🙌
To programmatically set the width and height of an ImageView
, you can use the LayoutParams
class provided by the parent view. Here's how:
val imageView = ImageView(context)
val layoutParams = ViewGroup.LayoutParams(widthInPixels, heightInPixels)
imageView.layoutParams = layoutParams
In the code snippet above, widthInPixels
and heightInPixels
represent the desired width and height of the ImageView
in pixels, respectively. You can replace these values with your own measurements. ✨
Example Usage 🖼️
Let's say you have an ImageView
defined in your layout XML file with the id imageView
. You can access this ImageView
programmatically and set its width and height like this:
val imageView = findViewById<ImageView>(R.id.imageView)
val layoutParams = imageView.layoutParams.apply {
width = widthInPixels
height = heightInPixels
}
imageView.layoutParams = layoutParams
Here, widthInPixels
and heightInPixels
are again the desired width and height values in pixels. Simply replace them with your own measurements as needed. You also need to make sure that you cast the result of findViewById()
to ImageView
so that you can access the layoutParams
property.
Common Pitfalls and Tips 💡
Make sure you set the width and height before the
ImageView
is laid out on the screen. Otherwise, the changes won't take effect.If you want to set the width and height programmatically for an
ImageView
that is already visible on the screen, you can callrequestLayout()
orinvalidate()
after updating thelayoutParams
to force a redraw.Remember to use pixels as the measurement unit when setting the width and height programmatically. You can convert from other units (like dp) using the appropriate conversion method provided by the
DisplayMetrics
class.
Summary 📝
Setting the width and height of an ImageView
programmatically in Android is easy once you know how to use the LayoutParams
class. By following the steps and examples provided in this article, you can confidently set the width and height of your ImageView
to your desired values, independent of the image size.
Now it's your turn! Try out the code snippets provided and let me know in the comments how it worked for you. If you have any questions or run into any issues, don't hesitate to ask. Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
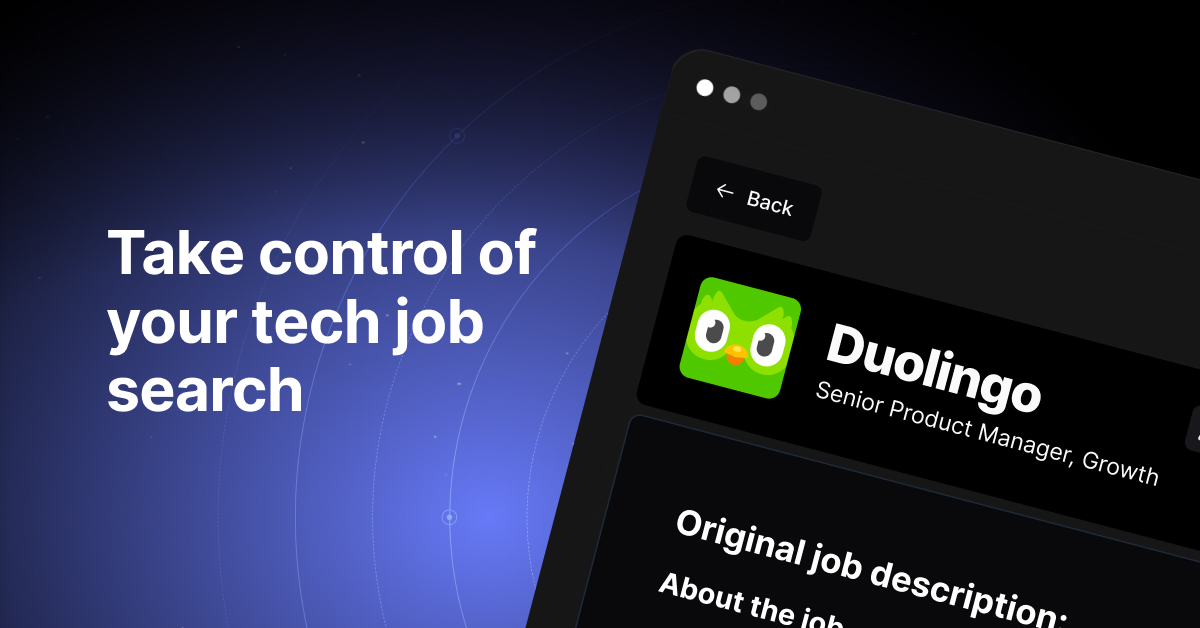