Serializing with Jackson (JSON) - getting "No serializer found"?
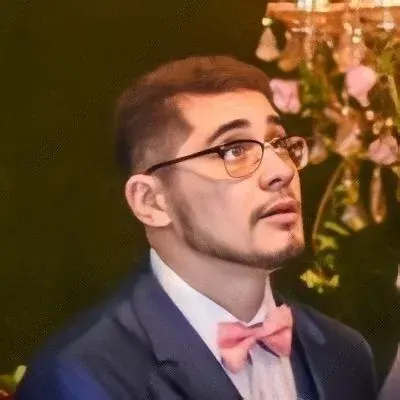
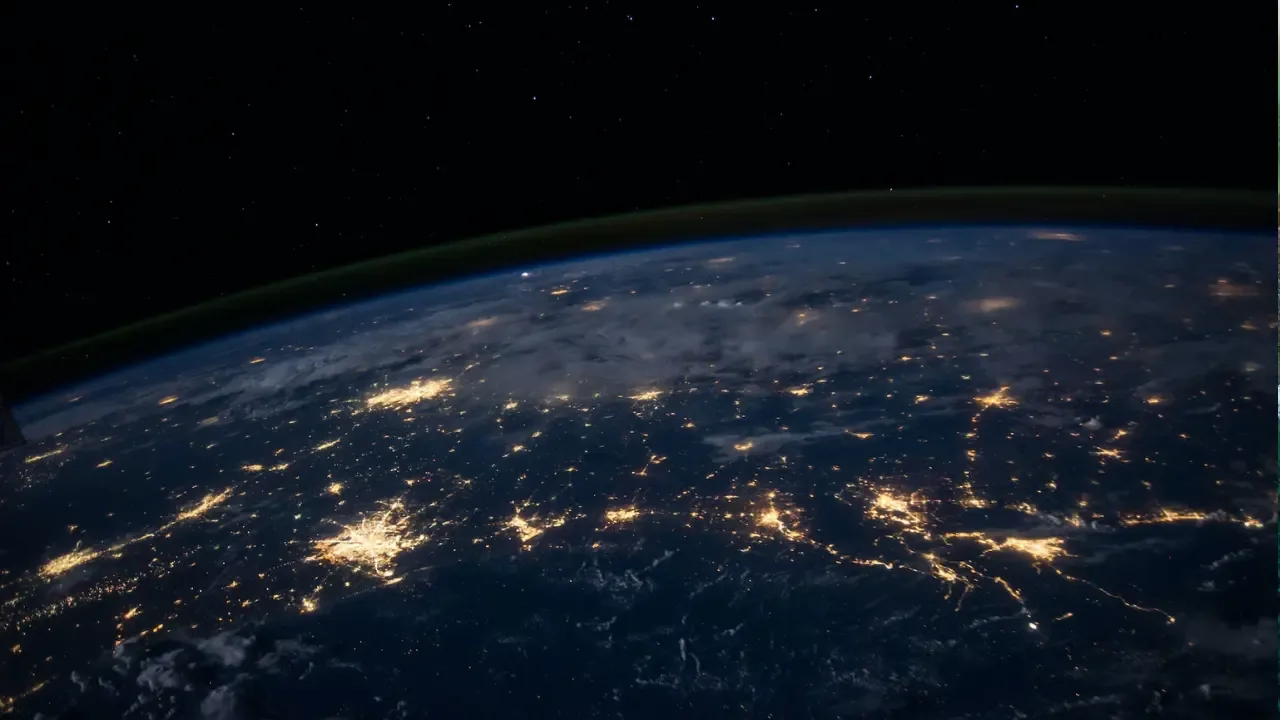
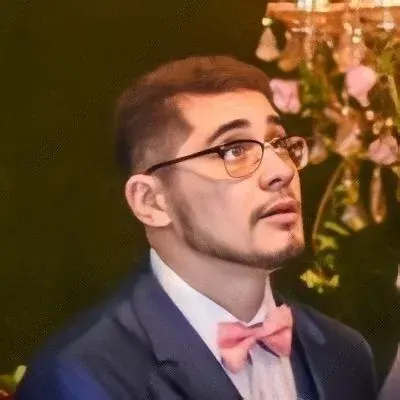
Serializing with Jackson (JSON) - getting "No serializer found"?
š Hey there tech enthusiasts! In today's blog post, we are going to dive into the mysterious world of serializing objects with Jackson (JSON). š
š¤ Have you ever encountered a frustrating error while trying to serialize a simple object using Jackson? Did you see the following exception?
org.codehaus.jackson.map.JsonMappingException: No serializer found for class MyPackage.TestA and no properties discovered to create BeanSerializer (to avoid exception, disable SerializationConfig.Feature.FAIL_ON_EMPTY_BEANS)
š„ Don't panic! You've come to the right place. We'll guide you through common issues and provide easy solutions to overcome this problem. Let's understand what's happening.
Understanding the Error
When you see the "No serializer found" exception, it means that Jackson is unable to find a serializer for the given class (in this case, MyPackage.TestA
). š±
To serialize an object into JSON using Jackson, it needs to know how to convert the object into its JSON representation. By default, Jackson relies on certain conventions like the presence of public getters and setters, fields with public visibility, or Jackson annotations to determine how to serialize an object.
The Simple Class and Code
Before we dive into solutions, let's take a look at the simple class and code provided in the question:
public class TestA {
String SomeString = "asd";
}
TestA testA = new TestA();
ObjectMapper om = new ObjectMapper();
try {
String testAString = om.writeValueAsString(testA); // error here!
TestA newTestA = om.readValue(testAString, TestA.class);
} catch (JsonGenerationException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (JsonMappingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
This code creates an instance of TestA
, attempts to serialize it into a JSON string using ObjectMapper
, and then tries to deserialize the JSON string back into a TestA
object.
Solution 1: Adding Getters and Setters
One common reason for the "No serializer found" exception is the absence of public getters and setters for the class's fields. š
In the provided TestA
class, the SomeString
field is not accompanied by a getter and setter method. To fix this, we need to add getters and setters for all the fields we want to serialize:
public class TestA {
private String SomeString;
public String getSomeString() {
return SomeString;
}
public void setSomeString(String someString) {
SomeString = someString;
}
}
Voila! š By adding the getters and setters, Jackson should be able to find the appropriate serializer for the TestA
class and successfully serialize and deserialize the object.
Solution 2: Configuring ObjectMapper
Another approach to solve the "No serializer found" issue is to configure the ObjectMapper
to handle empty beans differently. š ļø
By default, Jackson throws an exception if it encounters an empty bean. To disable this behavior and allow serialization of empty beans, you can set the FAIL_ON_EMPTY_BEANS
feature to false
:
ObjectMapper om = new ObjectMapper();
om.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
With this configuration, Jackson will skip over empty beans during serialization, preventing the "No serializer found" exception from being thrown.
Conclusion
And we're done! š We hope this guide has helped you understand and solve the "No serializer found" exception when serializing objects with Jackson.
Remember, the key takeaways are:
Ensure your class has public getters and setters for the fields you want to serialize.
Configure the
ObjectMapper
to handle empty beans accordingly.
Feel free to share your thoughts and experiences in the comments below, and don't forget to spread the word by sharing this post with your fellow developers.
Happy coding! šØāš»š©āš»