Sending Email in Android using JavaMail API without using the default/built-in app
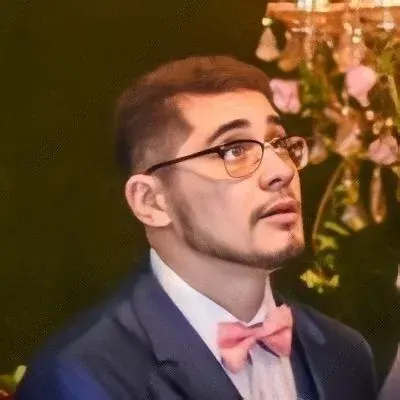
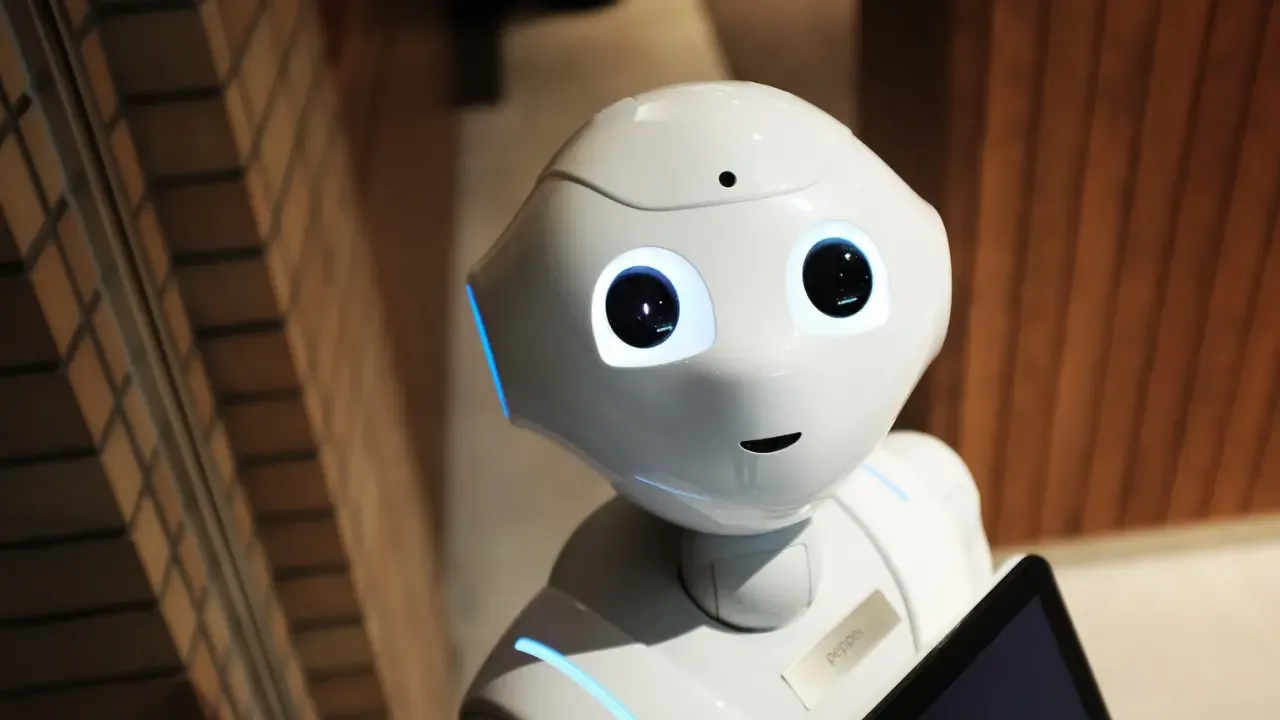
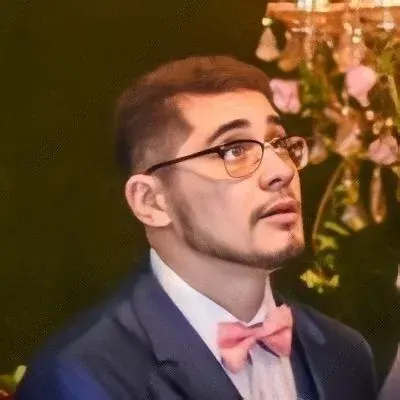
Sending Email in Android using JavaMail API 📧
Are you looking to create your own mail sending application in Android? Well, you've come to the right place! In this blog post, we'll explore how to send emails in Android using the JavaMail API without relying on the default/built-in app. 😎
The Challenge 💪
If you've tinkered with Android's Intent
class before, you might be familiar with the following code snippet:
Intent emailIntent = new Intent(android.content.Intent.ACTION_SEND);
The problem with this approach is that it launches the built-in Android application for sending emails. While this may work for some use cases, you may want to send the email directly without involving the user interface of another app. So, how do we accomplish this?
The Solution 🚀
To send emails in Android without the default app, we can use the JavaMail API, a powerful and versatile library. Let's break down the steps:
Step 1: Add the JavaMail API Library
First, we need to include the JavaMail API library in our Android project. You can download the JAR file from the official Oracle website or include it as a dependency using Gradle or Maven. Make sure to check the library's documentation for the latest versions and integration instructions.
Step 2: Set up the Email Configuration
Before we can send emails, we need to configure our email settings. This includes the email server, port number, username, password, and other required properties. Here's an example of how you can set up the email configuration using the JavaMail API:
Properties properties = new Properties();
properties.put("mail.smtp.host", "your_email_server");
properties.put("mail.smtp.port", "your_port_number");
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
properties.put("mail.smtp.starttls.required", "true");
Authenticator authenticator = new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_username", "your_password");
}
};
Step 3: Compose and Send the Email
Now that we've set up the email configuration, we can create an instance of the Session
class, which represents a mail session. We'll also create a MimeMessage
object to compose our email. Here's an example:
Session session = Session.getDefaultInstance(properties, authenticator);
try {
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress("your_email_address"));
message.setRecipient(Message.RecipientType.TO, new InternetAddress("recipient_email_address"));
message.setSubject("Your email subject");
message.setText("Hello, this is the body of your email!");
Transport.send(message);
} catch (MessagingException e) {
e.printStackTrace();
}
Congratulations! 🎉 You've successfully sent an email using the JavaMail API in Android!
Common Issues and Troubleshooting 🛠️
Issue 1: "JavaMail API classes are not found."
If you encounter this issue, make sure you have correctly added the JavaMail API library to your project. Double-check the JAR file location or the dependency configuration. It's also important to ensure that you are using the correct import statements in your code.
Issue 2: "Authentication failed."
If you receive an authentication error, verify that the username and password you provided are correct. Additionally, ensure that your email server supports the chosen authentication method.
Your Turn! 💌
We hope this guide has helped you learn how to send emails in Android using the JavaMail API without relying on the default app. Now it's your turn to explore and implement it in your own project! If you encounter any issues or have any questions, feel free to leave a comment below.
Happy coding! 💻✨