Scanner is skipping nextLine() after using next() or nextFoo()?
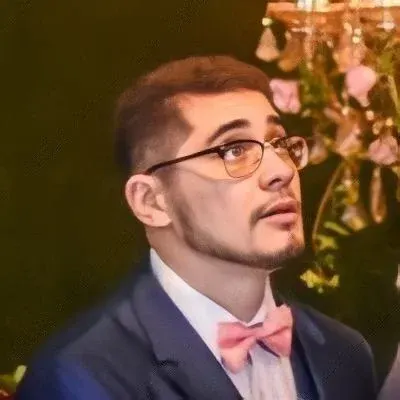
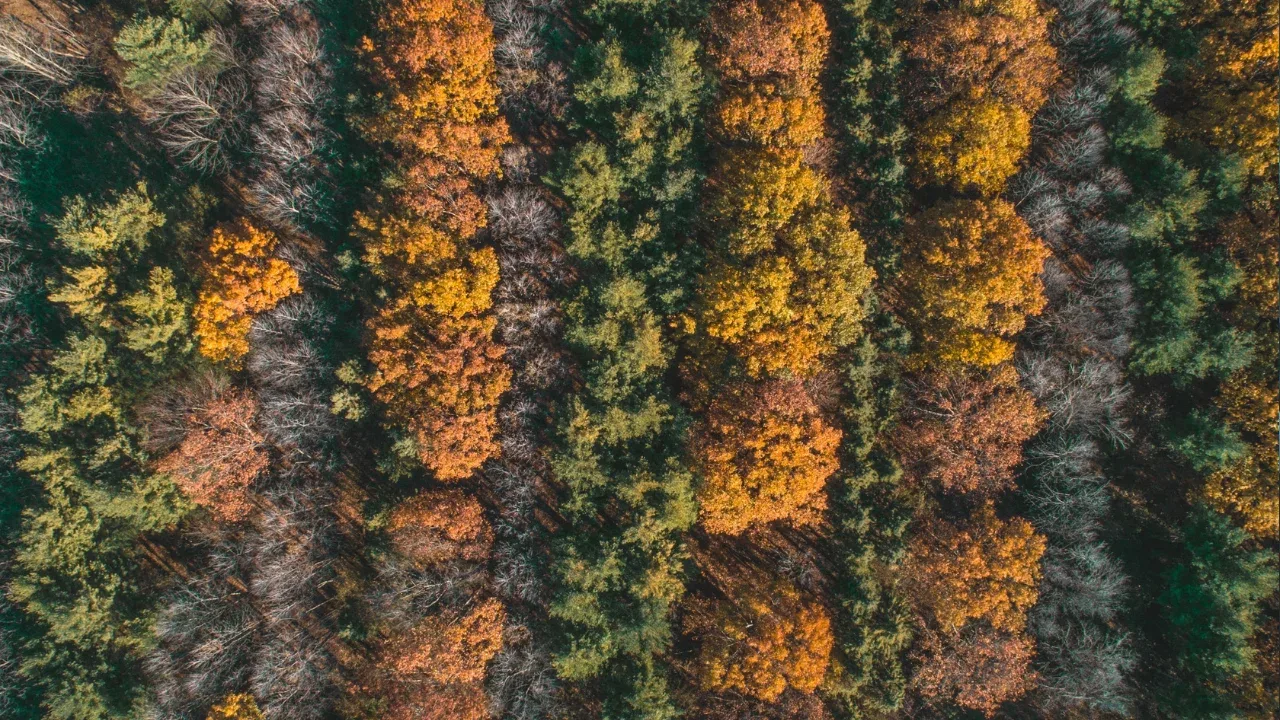
Scanner is skipping nextLine() after using next() or nextFoo()? 😕
Are you facing an issue with the Scanner class where the nextLine()
method is being skipped after using the next()
or nextFoo()
methods? 🤔 This may be a common problem when using the Scanner class in Java. But don't worry, we've got you covered with an easy solution! 💪
Let's take a look at your code example to understand the issue:
System.out.println("Enter numerical value");
int option;
option = input.nextInt(); // Read numerical value from input
System.out.println("Enter 1st string");
String string1 = input.nextLine(); // Read 1st string (this is skipped)
System.out.println("Enter 2nd string");
String string2 = input.nextLine(); // Read 2nd string (this appears right after reading numerical value)
The problem is that after entering the numerical value, the first input.nextLine()
is being skipped, and the second input.nextLine()
is executed immediately. This results in an unexpected behavior where you can't input the first string. 😫
Understanding the issue
The root cause of this problem lies in how the Scanner
class handles input. When you use the nextInt()
method to read an integer, it only consumes the numerical value, leaving the newline character (\n
) in the input buffer. 😲
Now, when the nextLine()
method is called after nextInt()
, it reads the remaining newline character from the buffer and considers it as the input. This leads to the first nextLine()
being skipped. 🚫
Solution: Consume the newline character
To fix this issue, we need to consume the newline character (\n
) from the input buffer before calling nextLine()
to read the string. We can achieve this by adding an extra input.nextLine()
after nextInt()
. Let me show you how to modify your code:
System.out.println("Enter numerical value");
int option;
option = input.nextInt(); // Read numerical value from input
input.nextLine(); // Consume the newline character
System.out.println("Enter 1st string");
String string1 = input.nextLine(); // Read 1st string
System.out.println("Enter 2nd string");
String string2 = input.nextLine(); // Read 2nd string
By adding input.nextLine()
right after nextInt()
, we ensure that the newline character is consumed, and subsequent nextLine()
calls work correctly. 🙌
Give it a try!
Now it's time for you to try the updated code! Simply modify your code as shown above, and you should be able to input both strings as expected. 👍
Run the program, enter your desired input, and voila! The scanner will now behave properly and execute the nextLine()
calls as intended.
If you encounter any issues or have any questions, feel free to leave a comment below. Our community is always here to help you out. 🤗
Share your experience!
Did this solution work for you? Share your experience in the comments below! ✨ We'd love to hear if we were able to resolve the skipping nextLine()
issue with the Scanner class.
If you found this article helpful, don't forget to share it with your fellow developers! Let's make everyone's coding experience smoother by tackling these little hurdles along the way. 🌟
Now go ahead, try out the solution, and happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
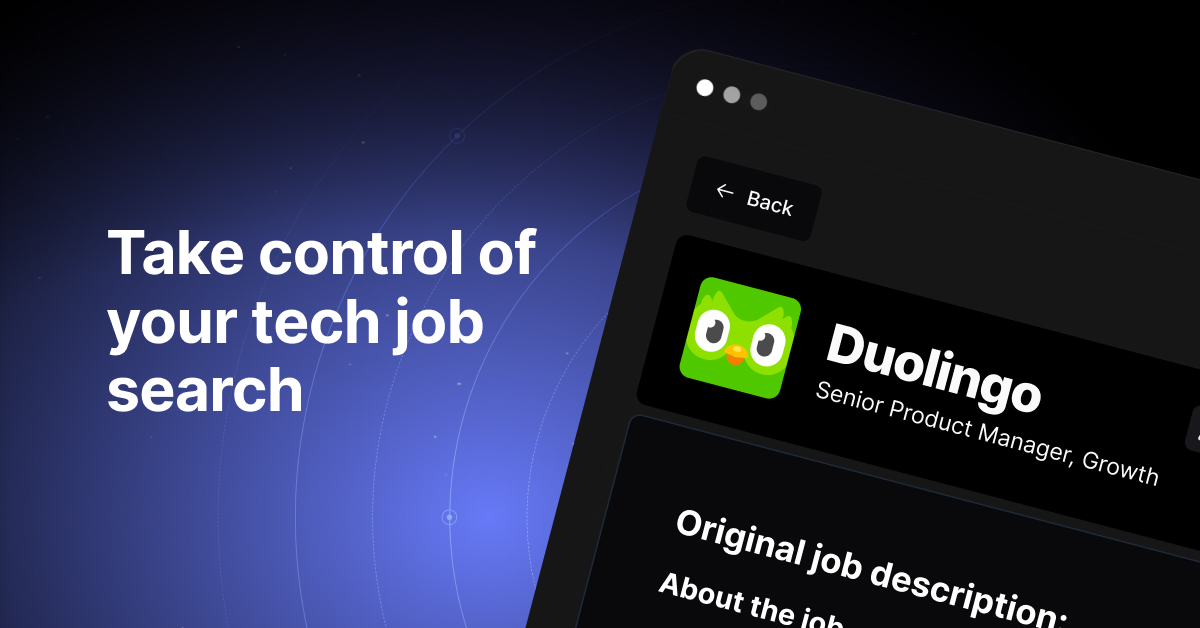