Reverse a string in Java
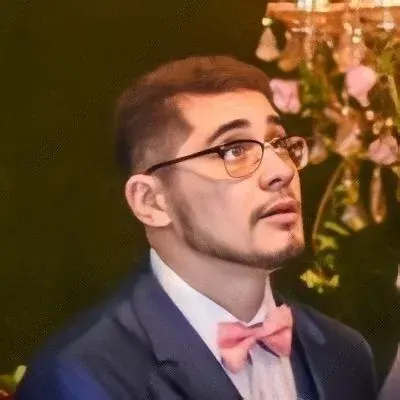
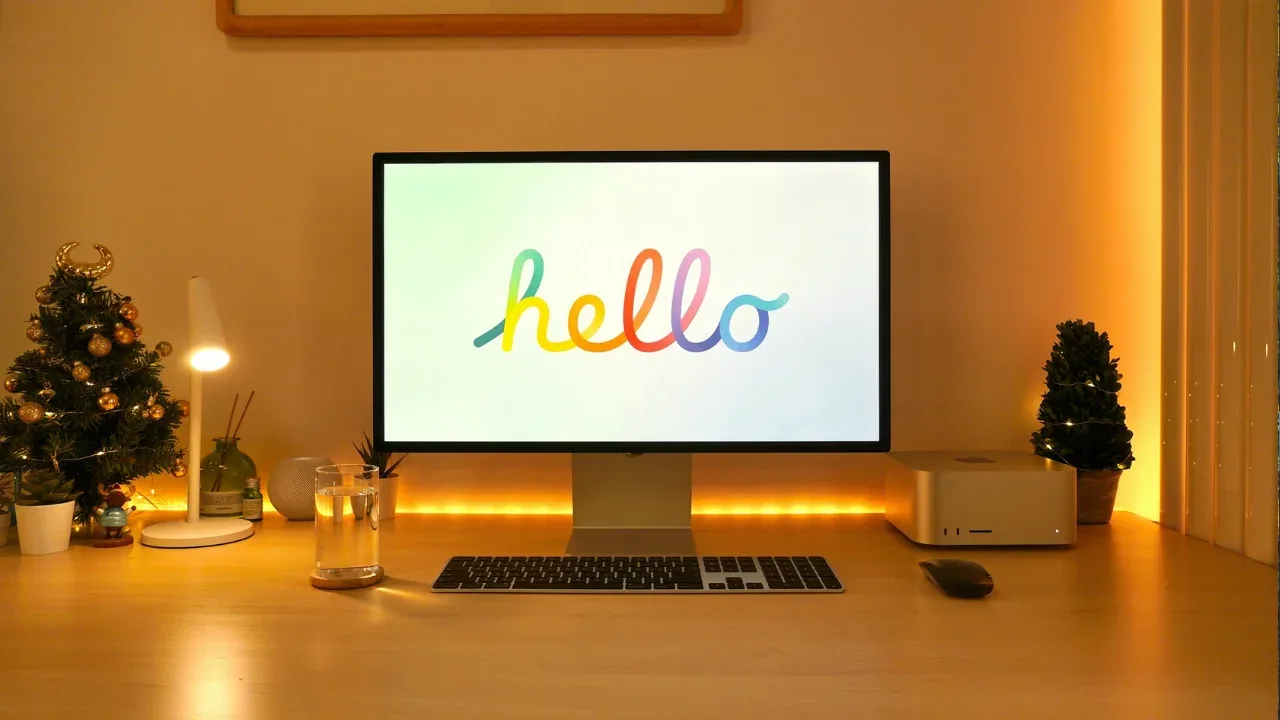
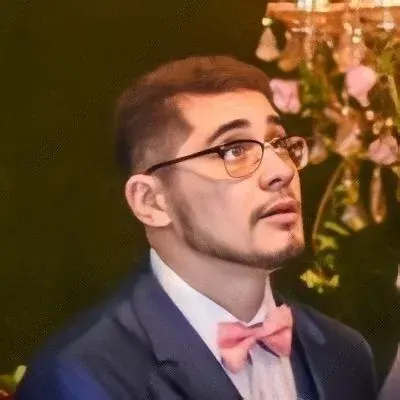
Reverse a String in Java: Easy Solutions for a Common Problem! 🔄
Have you ever found yourself needing to reverse a string in Java? Maybe you are working on a project where you need to display a string backward, or you simply want to manipulate a string in a different way. Whatever the reason may be, you're in the right place!
In this article, we will explore common issues when it comes to reversing a string in Java and provide you with easy solutions. So, let's dive right in!
Common Issue: Reversing a String
Let's consider a scenario where we have a string variable hi
containing the value "Hello World". Our task is to reverse this string and print it out. How can we achieve that?
Solution: Utilizing Built-in Java Functions
Rather than manually writing code to reverse the string, Java provides us with a built-in function called StringBuilder.reverse()
that can do the job for us. 😎
Here's how we can use it:
String hi = "Hello World";
String reversedString = new StringBuilder(hi).reverse().toString();
System.out.println(reversedString); // Output: "dlroW olleH"
In this solution, we create a StringBuilder
object using the input string hi
. We then chain the reverse()
function to reverse the string and finally use toString()
to convert it back to a regular string.
That's it! We've successfully reversed the string in just a few lines of code. 👍
Get Creative: Reverse Each Individual Word
While we're at it, let's get a bit more creative! Another frequently encountered scenario is reversing each individual word within a string. For example, turning "Hello World" into "olleH dlroW".
String originalString = "Hello World";
String[] words = originalString.split(" ");
StringBuilder reversedWords = new StringBuilder();
for (String word : words) {
reversedWords.append(new StringBuilder(word).reverse().toString()).append(" ");
}
String reversedString = reversedWords.toString().trim();
System.out.println(reversedString); // Output: "olleH dlroW"
In this code snippet, we split the original string into an array of words using the split()
function. Then, we loop through each word, reverse it using the StringBuilder
and append it to our reversedWords
object. Finally, we obtain the reversed string by converting reversedWords
back to a string, trimming any extra spaces, and printing it out.
Your Turn: Let's Reverse Some Strings!
Now that you have the tools and solutions at hand, it's time for you to put them into practice! Take a moment to experiment with reversing strings in Java using the techniques we've shared.
If you come across any questions or want to share your own creative solutions, we'd love to hear from you! Leave a comment below or head over to our tech community to engage with like-minded developers.
Happy coding! 🚀