@RequestParam vs @PathVariable
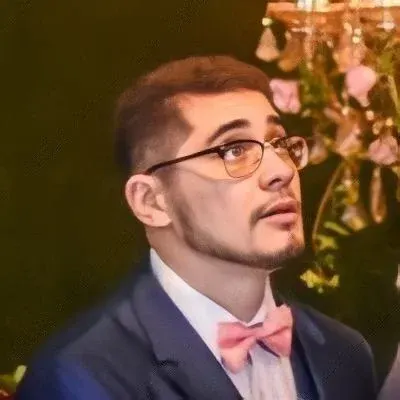

RequestParam vs PathVariable: Handling Special Characters
Understanding the Difference
š¹ When working with Spring MVC in Java, we often come across the need to handle special characters in request parameters and path variables. Two commonly used annotations for this purpose are @RequestParam
and @PathVariable
.
š¤ But what exactly is the difference between them, especially when dealing with special characters?
The Problem
⨠Let's take a look at the scenario mentioned in the question:
@GetMapping("/example")
public String exampleMethod(@RequestParam String param, @PathVariable String variable) {
// code here
}
The issue arises when we encounter special characters such as +
in the values of these parameters.
ā
With @RequestParam
, if the parameter value contains a +
, it will be accepted as a space. For example, if we pass param=hello+world
, the value received by the method will be hello world
.
ā On the other hand, @PathVariable
does not consider +
as a space. So, if we pass variable=hello+world
, the value received will remain hello+world
.
The Solution
š To handle special characters consistently across both annotations, we can employ the UriUtils
class from Spring's org.springframework.web.util
package.
ā Here's an updated version of our example method:
import org.springframework.web.util.UriUtils;
import java.nio.charset.StandardCharsets;
@GetMapping("/example")
public String exampleMethod(@RequestParam String param, @PathVariable String variable) {
String decodedParam = UriUtils.decode(param, StandardCharsets.UTF_8);
String decodedVariable = UriUtils.decode(variable, StandardCharsets.UTF_8);
// code here, now using decodedParam and decodedVariable
}
By using UriUtils.decode
with the StandardCharsets.UTF_8
parameter, we can correctly handle special characters, including +
, in both @RequestParam
and @PathVariable
.
Take Action
š¢ Don't let special characters trip you up! Use these tips and tricks to handle them smoothly in your Spring MVC applications.
š¬ If you have any questions or faced other challenges with handling special characters, let us know in the comments below. We would love to help you out!
š Share this post with your fellow developers who might be struggling with special characters in request parameters and path variables. Spread the knowledge!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
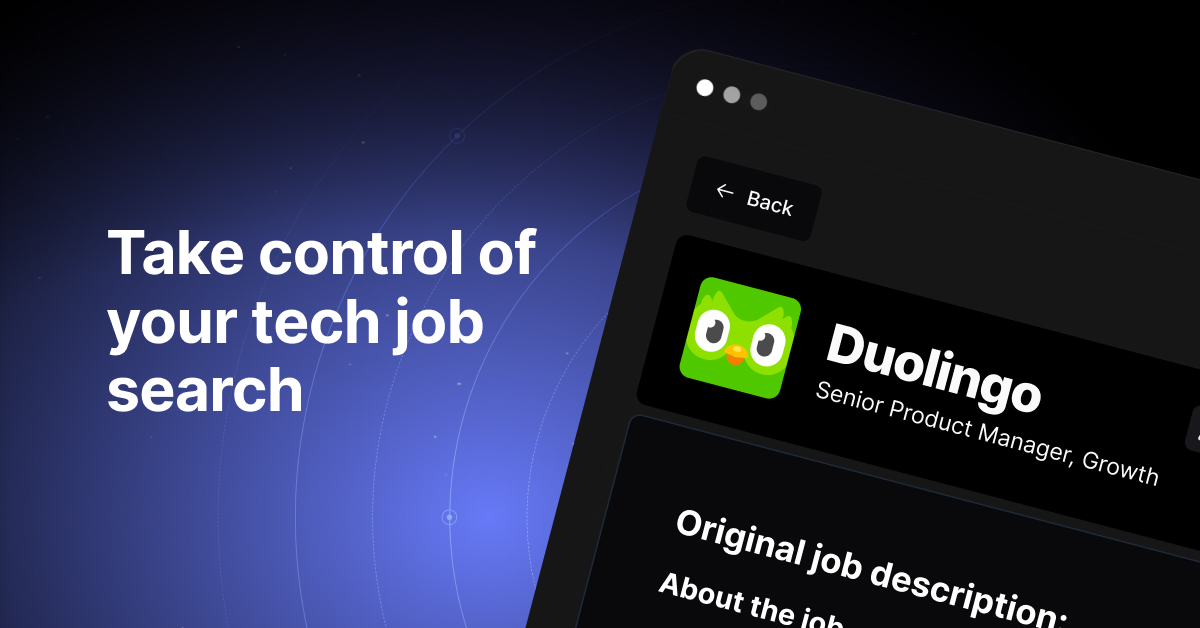