@RequestParam in Spring MVC handling optional parameters
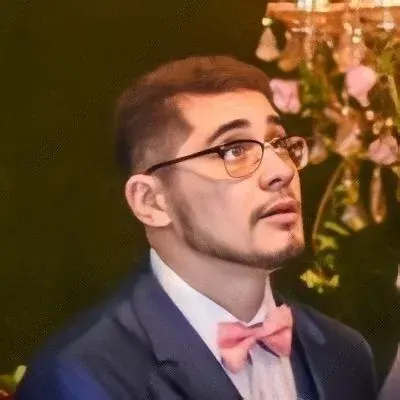
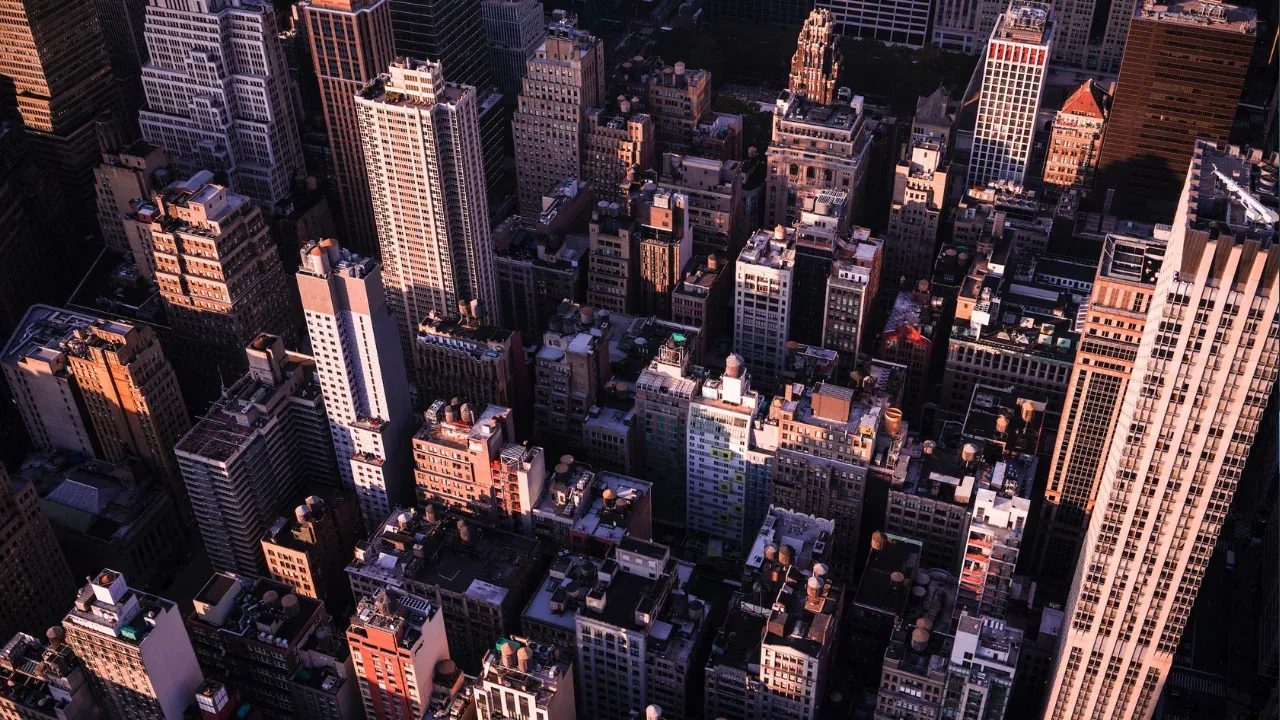
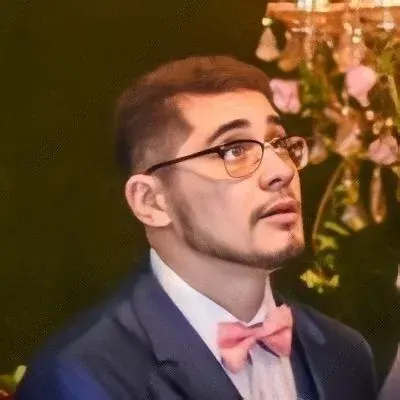
Handling Optional Parameters with @RequestParam in Spring MVC
Are you struggling with handling optional parameters in your Spring MVC application? Do you want to know how to handle different types of requests in your controllers? Look no further! In this blog post, I will walk you through the common issues and provide easy solutions for using the @RequestParam
annotation in Spring MVC.
The Problem
Let's start by examining the problem at hand. You have a Spring controller that needs to handle two types of requests:
http://localhost:8080/submit/id/ID123432?logout=true
http://localhost:8080/submit/id/ID123432?name=sam&password=543432
You might think that defining a single controller method to handle both requests would be the way to go. However, you encounter an issue. If you define a controller method like this:
@RequestMapping(value = "/submit/id/{id}", method = RequestMethod.GET, produces = "text/xml")
public String showLoginWindow(@PathVariable("id") String id,
@RequestParam(value = "logout", required = false) String logout,
@RequestParam("name") String username,
@RequestParam("password") String password,
@ModelAttribute("submitModel") SubmitModel model,
BindingResult errors) throws LoginException {...}
The problem arises when you make a request with the "logout" parameter. It is not accepted by the controller.
The Solution
To handle optional parameters in Spring MVC, you can make use of the defaultValue
attribute of the @RequestParam
annotation. By providing a default value, you ensure that the parameter is always present, even if the request does not explicitly include it. Let's update the controller method to address this issue:
@RequestMapping(value = "/submit/id/{id}", method = RequestMethod.GET, produces = "text/xml")
public String showLoginWindow(@PathVariable("id") String id,
@RequestParam(value = "logout", defaultValue = "false") String logout,
@RequestParam("name") String username,
@RequestParam("password") String password,
@ModelAttribute("submitModel") SubmitModel model,
BindingResult errors) throws LoginException {...}
With this change, the "logout" parameter is now optional, and if it is not present in the request, its default value is set to "false". This allows the controller to accept both types of requests mentioned earlier.
Avoiding Bean Method Mapping Exceptions
Now, you might be wondering what to do if you want to handle the two types of requests separately, but Spring complains with the exception "There is already 'Controller' bean method ... mapped". You can solve this problem by using different URL mappings for each controller method. Let's update our controller to handle each request separately:
@RequestMapping(value = "/submit/id/{id}", method = RequestMethod.GET, produces = "text/xml", params = "logout")
public String handleLogoutRequest(@PathVariable("id") String id,
@RequestParam("logout") String logout,
@ModelAttribute("submitModel") SubmitModel model,
BindingResult errors) throws LoginException {...}
@RequestMapping(value = "/submit/id/{id}", method = RequestMethod.GET, produces = "text/xml", params = "!logout")
public String handleLoginRequest(@PathVariable("id") String id,
@RequestParam("name") String username,
@RequestParam("password") String password,
@ModelAttribute("submitModel") SubmitModel model,
BindingResult errors) throws LoginException {...}
By specifying the params
attribute in the @RequestMapping
annotation, we can differentiate between the two controller methods based on the presence or absence of the "logout" parameter. This allows Spring to map each method to the corresponding type of request without throwing any exceptions.
Conclusion
Handling optional parameters in Spring MVC can be challenging, but with the right approach, you can overcome these hurdles. By using the @RequestParam
annotation and providing default values or specifying different URL mappings, you can handle different types of requests effortlessly.
I hope this guide has provided you with valuable insights and solutions to your Spring MVC woes. Give these techniques a try in your own application and let me know how they work for you!
If you have any further questions or face any difficulties, feel free to leave a comment below. Happy coding! 😊🚀