Replacing all non-alphanumeric characters with empty strings
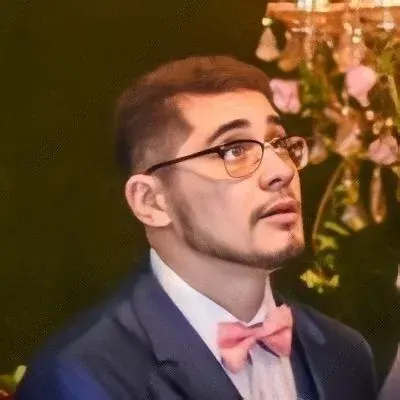
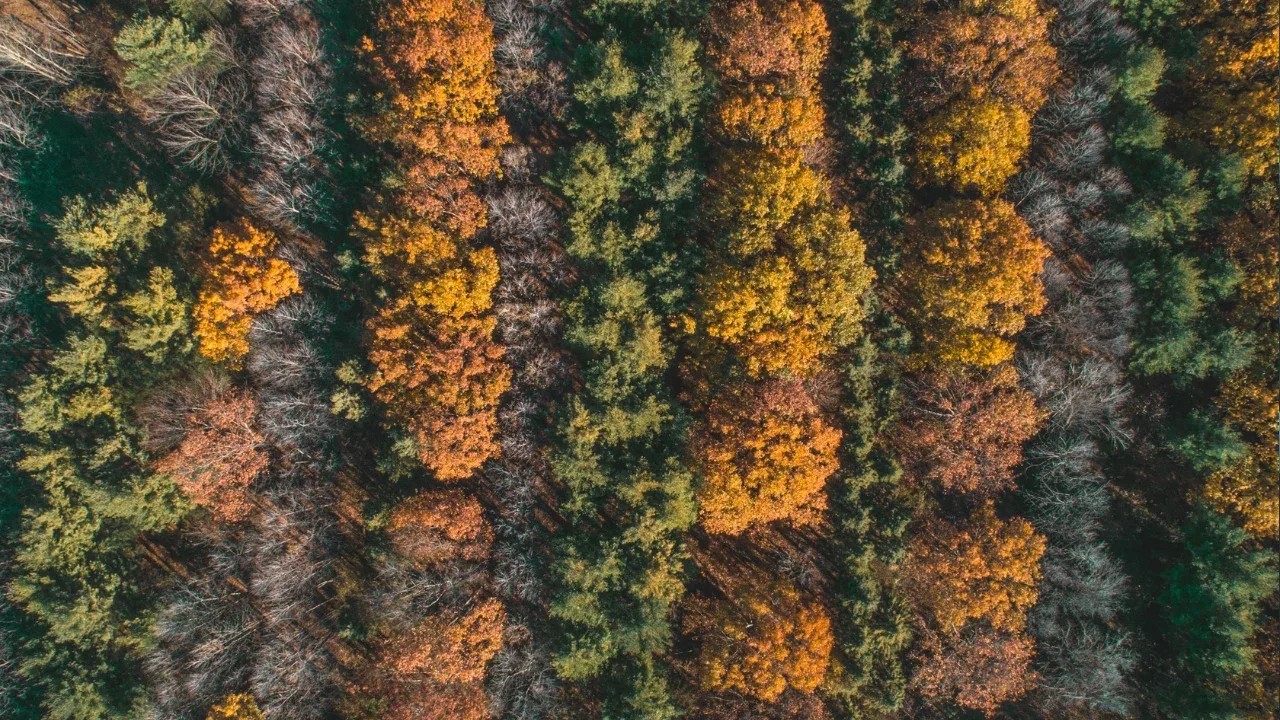
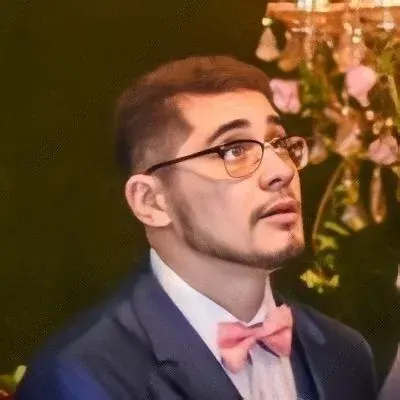
📝 Tech Blog: Removing Non-Alphanumeric Characters Made Easy! 😎
Are non-alphanumeric characters giving you a hard time? Don't fret! In this blog post, we'll tackle the common issue of replacing all non-alphanumeric characters with empty strings and provide you with some quick and easy solutions. 💪
The Problem: So, you tried using the following code snippet, but it didn't work as expected:
return value.replaceAll("/[^A-Za-z0-9 ]/", "");
Let's discuss what went wrong and explore alternative solutions. 🤔
Understanding the Issue:
The problem lies within the regular expression pattern used in the replaceAll
method. In Java, regular expression patterns should not be enclosed in forward slashes (/), as you would typically do in some other programming languages. This usage is more common in JavaScript or Perl, for example.
Solution 1: To fix the issue, we need to remove the forward slashes from the regular expression pattern. The correct code snippet would be:
return value.replaceAll("[^A-Za-z0-9 ]", "");
Here, we removed the forward slashes and placed the regular expression pattern within square brackets [].
Solution 2:
If you prefer a more readable approach, you can use the Pattern
and Matcher
classes from the java.util.regex
package:
import java.util.regex.Pattern;
import java.util.regex.Matcher;
Pattern pattern = Pattern.compile("[^A-Za-z0-9 ]");
Matcher matcher = pattern.matcher(value);
return matcher.replaceAll("");
This solution is particularly useful when you need to perform multiple replacements or more complex regex operations in your code. 🧩
Solution 3: If you're working with strings in JavaScript, the correct code to achieve the desired result would be:
return value.replaceAll(/[^A-Za-z0-9 ]/g, "");
Here, we use the forward slashes (/) to delimit the regular expression pattern and add the 'g' flag at the end to replace all occurrences in the string.
Call-to-Action: We hope these solutions have helped you conquer the challenge of removing non-alphanumeric characters! If you have any questions or need further assistance, feel free to leave a comment below. Also, don't forget to share this blog post with your tech-savvy friends who might find it useful. 🌟
Now go forth and code with confidence, my friends! Happy coding! 😄💻